Invoking APIs with OpenAPITool
Last Updated: December 27, 2024
OpenAPITool
is discontinued and removed inhaystack-experimental==0.4.0
. As an alternative, you can use openapi-llm.
Many APIs available on the Web provide an OpenAPI specification that describes their structure and syntax.
OpenAPITool
is an experimental Haystack component that allows you to call an API using payloads generated from human instructions.
Here’s a brief overview of how it works:
- At initialization, it loads the OpenAPI specification from a URL or a file.
- At runtime:
- Converts human instructions into a suitable API payload using a Chat Language Model (LLM).
- Invokes the API.
- Returns the API response, wrapped in a Chat Message.
Let’s see this component in action…
Setup
! pip install "haystack-experimental==0.3.0" haystack-ai jsonref
In this notebook, we will be using some APIs that require an API key. Let’s set them as environment variables.
import os
os.environ["OPENAI_API_KEY"]="..."
# free API key: https://www.firecrawl.dev/
os.environ["FIRECRAWL_API_KEY"]="..."
# free API key: https://serper.dev/
os.environ["SERPERDEV_API_KEY"]="..."
Call an API without credentials
In the first example, we use Open-Meteo, a Free Weather API that does not require authentication.
We use OPENAI
as LLM provider. Other supported providers are ANTHROPIC
and COHERE
.
from haystack.dataclasses import ChatMessage
from haystack_experimental.components.tools.openapi import OpenAPITool, LLMProvider
from haystack.utils import Secret
tool = OpenAPITool(generator_api=LLMProvider.OPENAI,
spec="https://raw.githubusercontent.com/open-meteo/open-meteo/main/openapi.yml")
tool.run(messages=[ChatMessage.from_user("Weather in San Francisco, US")])
{'service_response': [ChatMessage(content='{"latitude": 37.763283, "longitude": -122.41286, "generationtime_ms": 0.07700920104980469, "utc_offset_seconds": -25200, "timezone": "America/Los_Angeles", "timezone_abbreviation": "PDT", "elevation": 18.0, "current_weather_units": {"time": "iso8601", "interval": "seconds", "temperature": "\\u00b0C", "windspeed": "km/h", "winddirection": "\\u00b0", "is_day": "", "weathercode": "wmo code"}, "current_weather": {"time": "2024-07-29T06:45", "interval": 900, "temperature": 13.2, "windspeed": 12.7, "winddirection": 263, "is_day": 1, "weathercode": 45}}', role=<ChatRole.USER: 'user'>, name=None, meta={})]}
Incorporate OpenAPITool
in a Pipeline
Next, let’s create a simple Pipeline where the service response is translated into a human-understandable format using the Language Model.
We use a
ChatPromptBuilder
to create a list of Chat Messages for the LM.
from haystack import Pipeline
from haystack.components.builders import ChatPromptBuilder
from haystack.components.generators.chat import OpenAIChatGenerator
messages = [ChatMessage.from_user("{{user_message}}"), ChatMessage.from_user("{{service_response}}")]
builder = ChatPromptBuilder(template=messages)
pipe = Pipeline()
pipe.add_component("meteo", tool)
pipe.add_component("builder", builder)
pipe.add_component("llm", OpenAIChatGenerator(generation_kwargs={"max_tokens": 1024}))
pipe.connect("meteo", "builder.service_response")
pipe.connect("builder", "llm.messages")
<haystack.core.pipeline.pipeline.Pipeline object at 0x7f8899207e50>
🚅 Components
- meteo: OpenAPITool
- builder: ChatPromptBuilder
- llm: OpenAIChatGenerator
🛤️ Connections
- meteo.service_response -> builder.service_response (List[ChatMessage])
- builder.prompt -> llm.messages (List[ChatMessage])
result = pipe.run(data={"meteo": {"messages": [ChatMessage.from_user("weather in San Francisco, US")]},
"builder": {"user_message": [ChatMessage.from_user("Explain the weather in San Francisco in a human understandable way")]}})
print(result["llm"]["replies"][0].content)
The current weather in San Francisco is 13.2°C with a windspeed of 12.7 km/h coming from the west-southwest. It is currently daytime and the weather code indicates that it is partly cloudy.
Use an API with credentials in a Pipeline
In this example, we use Firecrawl: a project that scrape Web pages (and Web sites) and convert them into clean text. Firecrawl has an API that requires an API key.
In the following Pipeline, we use Firecrawl to scrape a news article, which is then summarized using a Language Model.
messages = [ChatMessage.from_user("{{user_message}}"), ChatMessage.from_user("{{service_response}}")]
builder = ChatPromptBuilder(template=messages)
pipe = Pipeline()
pipe.add_component("firecrawl", OpenAPITool(generator_api=LLMProvider.OPENAI,
spec="https://raw.githubusercontent.com/mendableai/firecrawl/main/apps/api/openapi.json",
credentials=Secret.from_env_var("FIRECRAWL_API_KEY")))
pipe.add_component("builder", builder)
pipe.add_component("llm", OpenAIChatGenerator(generation_kwargs={"max_tokens": 1024}))
pipe.connect("firecrawl", "builder.service_response")
pipe.connect("builder", "llm.messages")
<haystack.core.pipeline.pipeline.Pipeline object at 0x7f8899ea6ef0>
🚅 Components
- firecrawl: OpenAPITool
- builder: ChatPromptBuilder
- llm: OpenAIChatGenerator
🛤️ Connections
- firecrawl.service_response -> builder.service_response (List[ChatMessage])
- builder.prompt -> llm.messages (List[ChatMessage])
user_prompt = "Given the article below, list the most important facts in a bulleted list. Do not include repetitions. Max 5 points."
result = pipe.run(data={"firecrawl": {"messages": [ChatMessage.from_user("Scrape https://lite.cnn.com/2024/07/18/style/rome-ancient-papal-palace/index.html")]},
"builder": {"user_message": [ChatMessage.from_user(user_prompt)]}})
print(result["llm"]["replies"][0].content)
- Remains of a medieval palace believed to be where popes lived before the Vatican have been excavated in Rome.
- The site is located near the Archbasilica of St John Lateran in Rome.
- The building's initial structure dates back to Emperor Constantine in the 4th century and was expanded between the 9th and 13th centuries.
- The papacy resided in the palace until 1305 when it temporarily moved to Avignon in France.
- The discovery was made ahead of renovations for the 2025 Catholic Holy Year, expected to attract over 30 million pilgrims and tourists to Rome.
Create a Pipeline with multiple OpenAPITool
components
In this example, we show a Pipeline where multiple alternative APIs can be invoked depending on the user query. In particular, a Google Search (via Serper.dev) can be performed or a single page can be scraped using Firecrawl.
⚠️ The approach shown is just one way to achieve this using conditional routing. We are currently experimenting with tool support in Haystack, and there may be simpler ways to achieve the same result in the future.
import json
decision_prompt_template = """
You are a virtual assistant, equipped with the following tools:
- `{"tool_name": "search_web", "tool_description": "Access to Google search, use this tool whenever information on recents events is needed"}`
- `{"tool_name": "scrape_page", "tool_description": "Use this tool to scrape and crawl web pages"}`
Select the most appropriate tool to resolve the user's query. Respond in JSON format, specifying the user request and the chosen tool for the response.
If you can't match user query to an above listed tools, respond with `none`.
######
Here are some examples:
```json
{
"query": "Why did Elon Musk recently sue OpenAI?",
"response": "search_web"
}
{
"query": "What is on the front-page of hackernews today?",
"response": "scrape_page"
}
{
"query": "Tell me about Berlin",
"response": "none"
}
Choose the best tool (or none) for each user request, considering the current context of the conversation specified above.
{“query”: {{query}}, “response”: } """
def get_tool_name(replies): try: tool_name = json.loads(replies)[“response”] return tool_name except: return “error”
routes = [ { “condition”: “{{replies[0] | get_tool_name == ‘search_web’}}”, “output”: “{{query}}”, “output_name”: “search_web”, “output_type”: str, }, { “condition”: “{{replies[0] | get_tool_name == ‘scrape_page’}}”, “output”: “{{query}}”, “output_name”: “scrape_page”, “output_type”: str, }, { “condition”: “{{replies[0] | get_tool_name == ’none’}}”, “output”: “{{replies[0]}}”, “output_name”: “no_tools”, “output_type”: str, }, { “condition”: “{{replies[0] | get_tool_name == ’error’}}”, “output”: “{{replies[0]}}”, “output_name”: “error”, “output_type”: str, }, ]
```python
from haystack.components.builders import PromptBuilder, ChatPromptBuilder
from haystack.components.routers import ConditionalRouter
from haystack.components.generators import OpenAIGenerator
messages = [ChatMessage.from_user("{{query}}")]
search_web_chat_builder = ChatPromptBuilder(template=messages)
scrape_page_chat_builder = ChatPromptBuilder(template=messages)
search_web_tool = OpenAPITool(generator_api=LLMProvider.OPENAI,
spec="https://bit.ly/serper_dev_spec_yaml",
credentials=Secret.from_env_var("SERPERDEV_API_KEY"))
scrape_page_tool = OpenAPITool(generator_api=LLMProvider.OPENAI,
spec="https://raw.githubusercontent.com/mendableai/firecrawl/main/apps/api/openapi.json",
credentials=Secret.from_env_var("FIRECRAWL_API_KEY"))
pipe = Pipeline()
pipe.add_component("prompt_builder", PromptBuilder(template=decision_prompt_template))
pipe.add_component("llm", OpenAIGenerator())
pipe.add_component("router", ConditionalRouter(routes, custom_filters={"get_tool_name": get_tool_name}))
pipe.add_component("search_web_chat_builder", search_web_chat_builder)
pipe.add_component("scrape_page_chat_builder", scrape_page_chat_builder)
pipe.add_component("search_web_tool", search_web_tool)
pipe.add_component("scrape_page_tool", scrape_page_tool)
pipe.connect("prompt_builder", "llm")
pipe.connect("llm.replies", "router.replies")
pipe.connect("router.search_web", "search_web_chat_builder")
pipe.connect("router.scrape_page", "scrape_page_chat_builder")
pipe.connect("search_web_chat_builder", "search_web_tool")
pipe.connect("scrape_page_chat_builder", "scrape_page_tool")
<haystack.core.pipeline.pipeline.Pipeline object at 0x7f8899ea57e0>
🚅 Components
- prompt_builder: PromptBuilder
- llm: OpenAIGenerator
- router: ConditionalRouter
- search_web_chat_builder: ChatPromptBuilder
- scrape_page_chat_builder: ChatPromptBuilder
- search_web_tool: OpenAPITool
- scrape_page_tool: OpenAPITool
🛤️ Connections
- prompt_builder.prompt -> llm.prompt (str)
- llm.replies -> router.replies (List[str])
- router.search_web -> search_web_chat_builder.query (str)
- router.scrape_page -> scrape_page_chat_builder.query (str)
- search_web_chat_builder.prompt -> search_web_tool.messages (List[ChatMessage])
- scrape_page_chat_builder.prompt -> scrape_page_tool.messages (List[ChatMessage])
query = "Who won the UEFA European Football Championship?"
pipe.run({"prompt_builder": {"query": query}, "router": {"query": query}})
{'llm': {'meta': [{'model': 'gpt-4o-mini-2024-07-18',
'index': 0,
'finish_reason': 'stop',
'usage': {'completion_tokens': 23,
'prompt_tokens': 248,
'total_tokens': 271}}]},
'search_web_tool': {'service_response': [ChatMessage(content='{"searchParameters": {"q": "UEFA European Football Championship winner", "type": "search", "engine": "google"}, "answerBox": {"title": "Spain national football teamUEFA European Championship / Latest Champion", "answer": "Spain national football team"}, "organic": [{"title": "UEFA European Championship - Wikipedia", "link": "https://en.wikipedia.org/wiki/UEFA_European_Championship", "snippet": "The most recent championship, held in Germany in 2024, was won by Spain, who lifted a record fourth European title after beating England 2\\u20131 in the final at the ...", "sitelinks": [{"title": "Finals", "link": "https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals"}, {"title": "European Championship in", "link": "https://en.wikipedia.org/wiki/European_Championship_in_football"}, {"title": "UEFA Women\'s Championship", "link": "https://en.wikipedia.org/wiki/UEFA_Women%27s_Championship"}, {"title": "Euro 2024", "link": "https://en.wikipedia.org/wiki/UEFA_Euro_2024"}], "position": 1}, {"title": "Winners List of the UEFA European Championship - The Euros", "link": "https://www.topendsports.com/events/soccer/uefa-euros/winners.htm", "snippet": "Ten different countries have won the tournament: Spain have won four times, Germany has three titles, France and Italy with two titles while Portugal, ...", "position": 2}, {"title": "List of UEFA European Championship finals - Wikipedia", "link": "https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals", "snippet": "The winners of the first ever final, held in Paris in 1960, were the Soviet Union, who defeated Yugoslavia 2\\u20131 after extra time, while in the latest one, hosted ...", "sitelinks": [{"title": "History", "link": "https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals#History"}, {"title": "List of finals", "link": "https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals#List_of_finals"}, {"title": "Results by nation", "link": "https://en.wikipedia.org/wiki/List_of_UEFA_European_Championship_finals#Results_by_nation"}], "position": 3}, {"title": "UEFA Euro Winners List from 1960 to today - Marca.com", "link": "https://www.marca.com/en/football/uefa-euro/winners.html", "snippet": "Check the updated ranking of all the winners of the UEFA Euro Cup year by year. Record of European Championships throughout history in Marca English.", "sitelinks": [{"title": "Euro 2024 Live Scores", "link": "https://www.marca.com/en/scores/football/uefa-euro.html"}, {"title": "Euro 2024 Schedule", "link": "https://www.marca.com/en/football/uefa-euro/schedule.html"}, {"title": "Euro Stadiums Germany 2024", "link": "https://www.marca.com/en/football/uefa-euro/stadiums.html"}], "position": 4}, {"title": "Most titles | History | UEFA EURO", "link": "https://www.uefa.com/uefaeuro/history/winners/", "snippet": "View the official UEFA EURO winners list at UEFA.com. Find out which teams have lifted the most trophies since the competition began.", "sitelinks": [{"title": "Portugal 1-0 France", "link": "https://www.uefa.com/uefaeuro/match/2017907--portugal-vs-france/"}, {"title": "2012: Spain 4-0 Italy", "link": "https://www.uefa.com/uefaeuro/match/2003351--spain-vs-italy/"}, {"title": "West Germany vs USSR", "link": "https://www.uefa.com/uefaeuro/match/3838--west-germany-vs-ussr/"}, {"title": "Spain (1964)", "link": "https://www.uefa.com/uefaeuro/match/3996--spain-vs-ussr/"}], "position": 5}, {"title": "European Championship | History, Winners, & Facts | Britannica", "link": "https://www.britannica.com/sports/European-Championship", "snippet": "... football tournaments. Learn more about the European Championship, including its winners ... Also known as: Euro, European Nation\'s Cup, UEFA European Championship.", "position": 6}, {"title": "UEFA European Championship News, Stats, Scores - ESPN", "link": "https://www.espn.com/soccer/league/_/name/uefa.euro", "snippet": "Follow all the latest UEFA European Championship football news, fixtures, stats, and more on ESPN.", "position": 7}, {"title": "UEFA Euro winners: Know the champions - full list", "link": "https://olympics.com/en/news/uefa-european-championships-euro-winners-list-champions", "snippet": "Know all the UEFA European Championship winners. The Soviet Union won the first title in 1960 while Spain won the UEFA Euro 2024.", "date": "Jul 11, 2021", "position": 8}, {"title": "History | UEFA EURO", "link": "https://www.uefa.com/uefaeuro/history/", "snippet": "Official UEFA EURO history. Season-by-season guide, extensive all-time stats, plus video highlights of every final to date.", "sitelinks": [{"title": "Most titles", "link": "https://www.uefa.com/uefaeuro/history/winners/"}, {"title": "UEFA European...", "link": "https://www.uefa.com/uefaeuro/history/news/0253-0d81c56ff408-45bf000cd5b6-1000--uefa-european-championship-roll-of-honour/"}, {"title": "2020", "link": "https://www.uefa.com/uefaeuro/history/seasons/2020/"}, {"title": "All-time stats", "link": "https://www.uefa.com/uefaeuro/history/rankings/"}], "position": 9}, {"title": "UEFA EURO all-time winners 2024 - Statista", "link": "https://www.statista.com/statistics/378217/uefa-euro-titles-winners-and-finalists/", "snippet": "La Roja most recently won the competition in 2024, defeating England 2-1 in the EURO 2024 final. Read more. Countries with the most men\'s UEFA ...", "date": "6 days ago", "position": 10}], "peopleAlsoAsk": [{"question": "Who won UEFA European Championship?", "snippet": "Spain national football team\\nUEFA European Championship / Latest Champion", "title": ""}, {"question": "Who is the winner of European Champions League?", "snippet": "Real Madrid CF\\nUEFA Champions League / Latest Champion", "title": ""}, {"question": "How many countries have won the European football Championship?", "snippet": "Final tournament Map of countries\' best results. 10 countries have won, counting Germany and West Germany as one.", "title": "UEFA European Championship - Wikipedia", "link": "https://en.wikipedia.org/wiki/UEFA_European_Championship"}, {"question": "Who is the current women\'s European champion?", "snippet": "The competition is the women\'s equivalent of the UEFA European Championship. The reigning champions are England, who won their home tournament in 2022. The most successful nation in the history of the tournament is Germany, with eight titles.", "title": "UEFA Women\'s Championship - Wikipedia", "link": "https://en.wikipedia.org/wiki/UEFA_Women%27s_Championship"}], "relatedSearches": [{"query": "UEFA Euro 2020 Final"}, {"query": "Uefa european football championship winner list"}, {"query": "Most euro cup winners list"}, {"query": "Last Euro Cup winners"}, {"query": "Uefa european football championship winner 2021"}, {"query": "Euro Cup winners list men\'s"}, {"query": "Euro winners list since 2000"}, {"query": "Euro Cup winners list 2024"}, {"query": "Next Euro Cup 2024"}]}', role=<ChatRole.USER: 'user'>, name=None, meta={})]}}
query = "What is on the front-page of BBC today?"
pipe.run({"prompt_builder": {"query": query}, "router": {"query": query}})
{'llm': {'meta': [{'model': 'gpt-4o-mini-2024-07-18',
'index': 0,
'finish_reason': 'stop',
'usage': {'completion_tokens': 26,
'prompt_tokens': 250,
'total_tokens': 276}}]},
'scrape_page_tool': {'service_response': [ChatMessage(content='{"success": true, "data": {"content": "\\n\\n[British Broadcasting Corporation](/)\\n\\n[Watch Live](/watch-live-news)\\n\\nRegisterSign In\\n\\n* [Home](/)\\n \\n* [News](/news)\\n \\n* [Sport](/sport)\\n \\n* [Business](/business)\\n \\n* [Innovation](/innovation)\\n \\n* [Culture](/culture)\\n \\n* [Travel](/travel)\\n \\n* [Earth](/future-planet)\\n \\n* [Video](/video)\\n \\n* [Live](/live)\\n \\n\\nRegisterSign In\\n\\n[Home](/)\\n\\nNews\\n\\n[Sport](/sport)\\n\\nBusiness\\n\\nInnovation\\n\\nCulture\\n\\nTravel\\n\\nEarth\\n\\n[Video](/video)\\n\\nLive\\n\\n[Audio](https://www.bbc.co.uk/sounds)\\n\\n[Weather](https://www.bbc.com/weather)\\n\\n[Newsletters](https://www.bbc.com/newsletters)\\n\\n[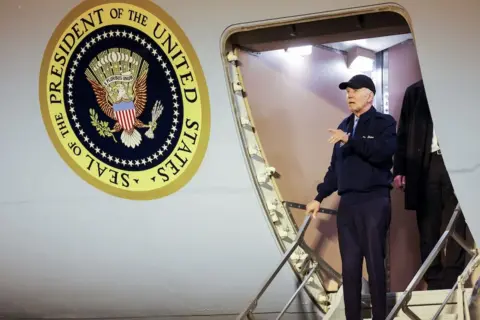\\\\\\n\\\\\\nThe last days of the Biden campaign \\u2013 BBC correspondent\\u2019s account\\\\\\n-----------------------------------------------------------------\\\\\\n\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\'s campaign.\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\n\\n[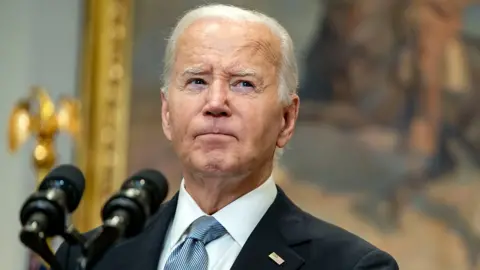\\\\\\n\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\n----------------------------------------------------------------------\\\\\\n\\\\\\nMost of the president\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\n\\\\\\n15 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\n\\n[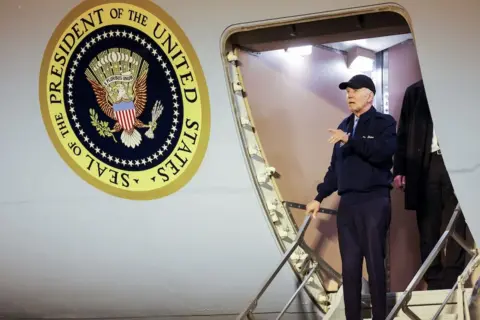\\\\\\n\\\\\\nThe last days of the Biden campaign \\u2013 BBC correspondent\\u2019s account\\\\\\n-----------------------------------------------------------------\\\\\\n\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\'s campaign.\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\n\\n[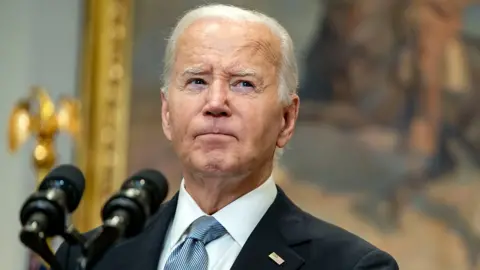\\\\\\n\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\n----------------------------------------------------------------------\\\\\\n\\\\\\nMost of the president\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\n\\\\\\n15 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\n\\n[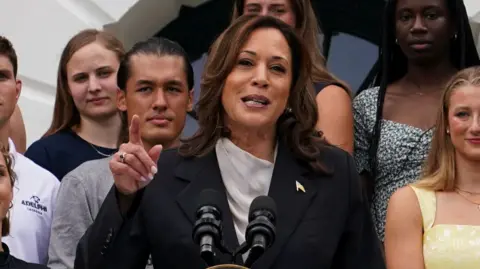\\\\\\n\\\\\\nLIVE\\\\\\n\\\\\\nKamala Harris speaks for first time since Biden left race - as endorsements mount\\\\\\n---------------------------------------------------------------------------------\\\\\\n\\\\\\nThe US vice-president appears at the White House for a pre-scheduled event - as key Democrats line up to back her candidacy.](https://www.bbc.com/news/live/cv2gryx1yx1t)\\n\\n* * *\\n\\n[What Biden quitting means for Harris, the Democrats and Trump\\\\\\n-------------------------------------------------------------\\\\\\n\\\\\\nPresident Biden has upended the 2024 White House race for the Democrats. Here is what it means for Kamala Harris, his party and Trump.\\\\\\n\\\\\\n19 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/cpwd8yzw45qo)\\n\\n[Who could be Kamala Harris\'s running mate?\\\\\\n------------------------------------------\\\\\\n\\\\\\nIt\\u2019s not a done deal but some potential rivals have quickly thrown their support behind her.\\\\\\n\\\\\\n10 mins ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\n\\n[LIVE\\\\\\n\\\\\\nTensions flare as Congress presses Secret Service boss on \'failed\' Trump rally security\\\\\\n---------------------------------------------------------------------------------------\\\\\\n\\\\\\nMembers of both parties have called for Kimberly Cheatle to resign in a House committee hearing that is seeking answers over security failures at the rally on 13 July.](https://www.bbc.com/news/live/c724wqpy4qnt)\\n\\n[The president\'s protectors are hardly noticeable - until things go wrong\\\\\\n------------------------------------------------------------------------\\\\\\n\\\\\\nThe attempted assassination of Donald Trump has raised questions about the Secret Service\'s record.\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c16j896003xo)\\n\\n* * *\\n\\nOnly from the BBC\\n-----------------\\n\\n[\\\\\\n\\\\\\nWhy you are probably sitting for too long\\\\\\n-----------------------------------------\\\\\\n\\\\\\nSitting down is ingrained in most peoples\' days. But staying sedentary for too long can increase the risk of serious health conditions like cardiovascular disease and type 2 diabetes.\\\\\\n\\\\\\n4 hrs ago\\\\\\n\\\\\\nFuture](/future/article/20240722-why-you-are-probably-sitting-down-for-too-long)\\n\\n[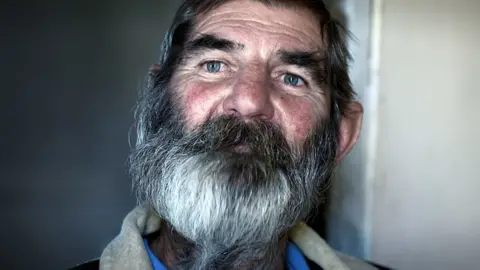\\\\\\n\\\\\\nMass killer who \\u2018hunted\\u2019 black people says police encouraged him\\\\\\n----------------------------------------------------------------\\\\\\n\\\\\\nEx-security guard, Louis van Schoor, killed dozens in South Africa but was only jailed for seven murders.\\\\\\n\\\\\\n17 hrs ago\\\\\\n\\\\\\nAfrica](/news/articles/c51yqdy3q61o)\\n\\n* * *\\n\\n[More news\\\\\\n---------](https://www.bbc.com/news)\\n\\u00a0\\n\\n[\\\\\\n\\\\\\nUAE jails 57 Bangladeshis over protests against own government\\\\\\n--------------------------------------------------------------\\\\\\n\\\\\\nThree defendants were sentenced to life after being convicted of \\"inciting riots\\" in the Gulf state.\\\\\\n\\\\\\n5 hrs ago\\\\\\n\\\\\\nWorld](/news/articles/crgk8gnpg0zo)\\n\\n[\\\\\\n\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\n\\n[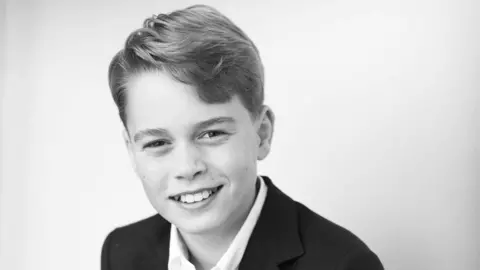\\\\\\n\\\\\\nNew Prince George photo released on 11th birthday\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\n\\\\\\n7 hrs ago\\\\\\n\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\n\\n[Piastri wins in Hungary after Norris team orders row\\\\\\n----------------------------------------------------\\\\\\n\\\\\\nOscar Piastri takes his maiden grand prix victory ahead of McLaren team-mate Lando Norris in a dramatic race in Hungary.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nFormula 1](/sport/formula1/articles/cg3jzy3e8q1o)\\n\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\n-----------------------------------------------------\\\\\\n\\\\\\nThe virus is transmitted from animals such as pigs and fruit bats to humans.\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\n\\n[\\\\\\n\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\n\\n[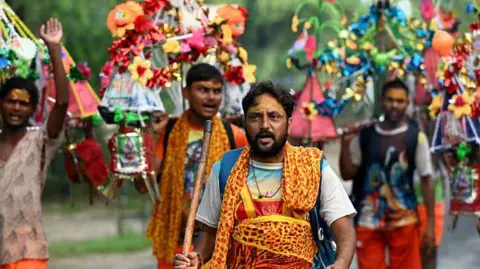\\\\\\n\\\\\\nIndia court blocks order for eateries to display owners\' names\\\\\\n--------------------------------------------------------------\\\\\\n\\\\\\nCritics say ordering restaurants to prominently display names of owners is discriminatory towards Muslims.\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/czrj18yp489o)\\n\\n[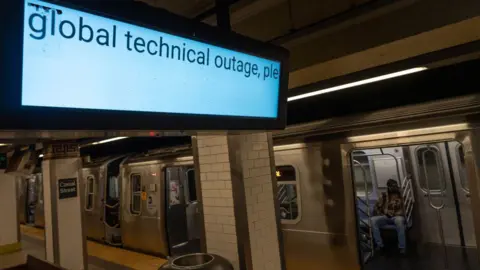\\\\\\n\\\\\\n\'Significant number\' of devices fixed - CrowdStrike\\\\\\n---------------------------------------------------\\\\\\n\\\\\\nCybersecurity firm behind global outage says it continues to focus on restoring all impacted computers.\\\\\\n\\\\\\n13 hrs ago\\\\\\n\\\\\\nBusiness](/news/articles/cgl7e33n1d0o)\\n\\n[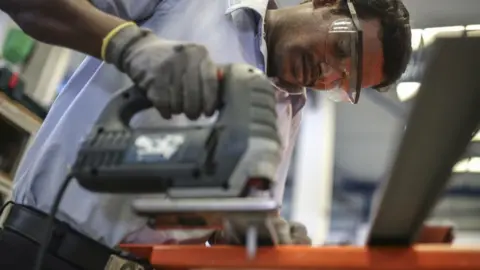\\\\\\n\\\\\\nModi\'s new budget faces jobs crisis test in India\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nMr Modi\'s biggest challenge in his third term will be bridging the rich-poor divide.\\\\\\n\\\\\\n16 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/cq5jwyel12qo)\\n\\n[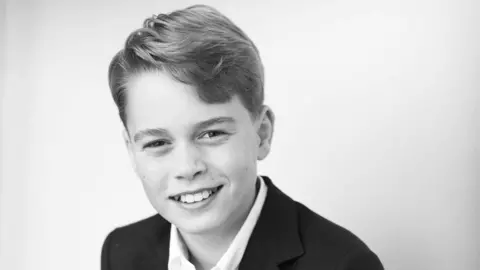\\\\\\n\\\\\\nNew Prince George photo released on 11th birthday\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\n\\\\\\n7 hrs ago\\\\\\n\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\n\\n* * *\\n\\nMust watch\\n----------\\n\\n[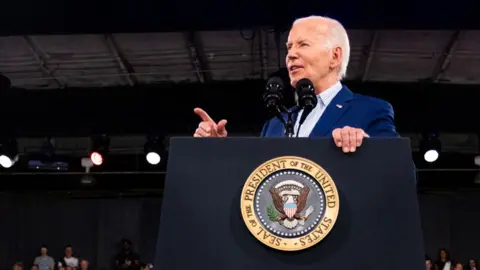\\\\\\n\\\\\\nBiden\\u2019s disastrous few weeks... in 90 seconds\\\\\\n---------------------------------------------\\\\\\n\\\\\\nPresident Biden has faced intense pressure to step aside since his faltering debate performance.\\\\\\n\\\\\\n17 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/videos/cx028eq4qg1o)\\n\\n[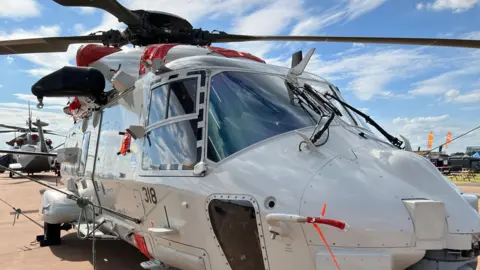\\\\\\n\\\\\\nInside the Netherlands Navy\'s anti-submarine helicopter\\\\\\n-------------------------------------------------------\\\\\\n\\\\\\nThe NH90 was on display at the 2024 Royal International Air Tattoo show at RAF Fairford.\\\\\\n\\\\\\n4 hrs ago\\\\\\n\\\\\\nEngland](/news/videos/cmj24x03r8po)\\n\\n[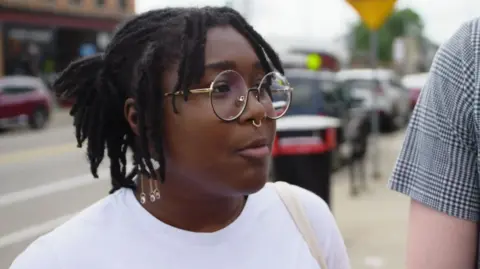\\\\\\n\\\\\\nDemocrats in Michigan react to Biden dropping out\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nDemocratic voters in Michigan give their take on Joe Biden withdrawing from the US presidential race.\\\\\\n\\\\\\n18 hrs ago](/news/videos/c51yrr2z74no)\\n\\n[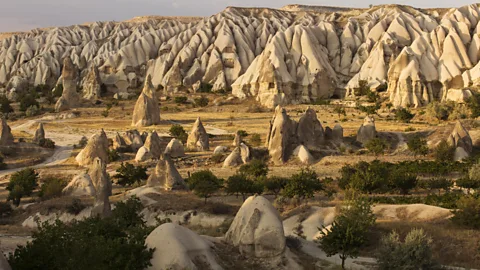\\\\\\n\\\\\\nTurkey\'s answer to \'Burning Man\'\\\\\\n--------------------------------\\\\\\n\\\\\\nA music and art extravaganza takes place in an \'otherworldly\' landscape amid unique volcanic rock formations.\\\\\\n\\\\\\n13 hrs ago\\\\\\n\\\\\\nCulture & Experiences](/reel/video/p0jch19q/turkey-s-answer-to-burning-man-)\\n\\n[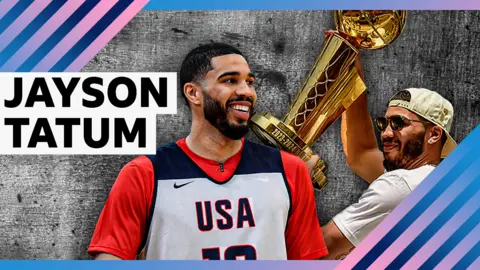\\\\\\n\\\\\\nTatum on handling criticism and \'joy\' of Olympics\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nTeam USA and Boston Celtics power forward Jayson Tatum says \\"basketball can\'t be your sole purpose\\" as he speaks about facing criticism, and the \\"joy\\" that playing in the Olympics brings.\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nOlympic Games](/sport/basketball/videos/cg640yk3n3zo)\\n\\n[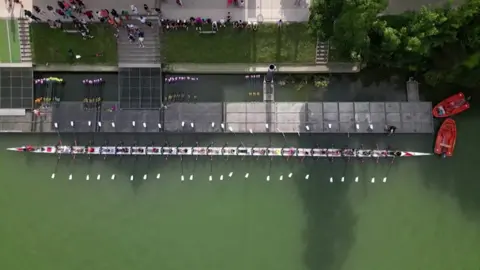\\\\\\n\\\\\\nWorld\'s longest rowing boat to carry Olympic torch\\\\\\n--------------------------------------------------\\\\\\n\\\\\\nThe 24-seater boat will take the Olympic torch down a section of the River Marne on Sunday.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nEurope](/news/videos/cqe6q917y1jo)\\n\\n[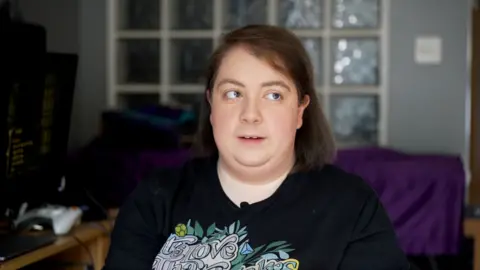\\\\\\n\\\\\\nAccessibility brings disabled gamers a sense of community\\\\\\n---------------------------------------------------------\\\\\\n\\\\\\nGreater accessibility in game development is opening the genre to more people with disabilities.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nScotland](/news/videos/c0jq593xqk8o)\\n\\n[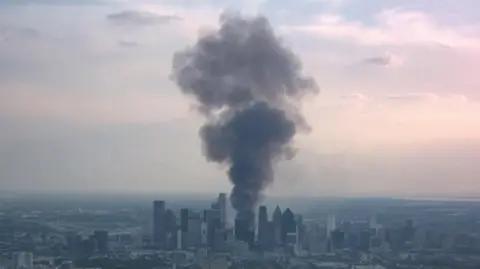\\\\\\n\\\\\\nWatch: Spire collapses as fire engulfs Texas church\\\\\\n---------------------------------------------------\\\\\\n\\\\\\nA blaze at a historic church in Dallas has caused huge plumes of smoke to rise over the Texan city\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nUS & Canada](/news/videos/cjk325014g7o)\\n\\n* * *\\n\\nIn History\\n----------\\n\\n[\\\\\\n\\\\\\n\'He was after my life\': WW1 soldier\'s confession\\\\\\n------------------------------------------------\\\\\\n\\\\\\nWorld War One broke out on 28 July, 1914. Fifty years later, one of the German soldiers, Stefan Westmann, told the BBC about his experiences fighting in the conflict.\\\\\\n\\\\\\nSee more](/culture/article/20240718-a-ww1-soldier-on-the-brutality-of-conflict)\\n\\n* * *\\n\\n[Olympic Games\\\\\\n-------------](https://www.bbc.com/sport/olympics)\\n\\u00a0\\n\\n[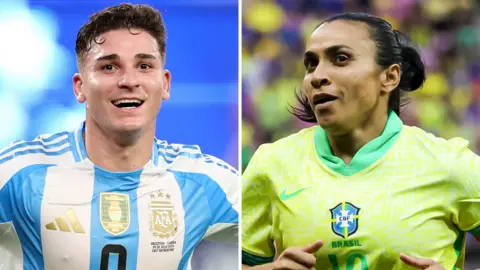\\\\\\n\\\\\\nTen footballers to watch out for at Paris Olympics\\\\\\n--------------------------------------------------\\\\\\n\\\\\\nFrom Manchester City\'s Julian Alvarez to Brazil icon Marta, BBC Sport picks out 10 footballers to watch at the Olympics.\\\\\\n\\\\\\nSee more](/sport/football/articles/cek91m98g48o)\\n\\n* * *\\n\\nUS and Canada news\\n------------------\\n\\n[\'The right move but is it too late?\' Democratic voters react\\\\\\n------------------------------------------------------------\\\\\\n\\\\\\nJust now\\\\\\n\\\\\\nUS & Canada](/news/articles/crgk8rgm87lo)\\n\\n[Who could be Kamala Harris\'s running mate?\\\\\\n------------------------------------------\\\\\\n\\\\\\n10 mins ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\n\\n[Biden has backed Harris. What happens next in US election?\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\n18 mins ago\\\\\\n\\\\\\nUS & Canada](/news/articles/cq5xdq71drro)\\n\\n[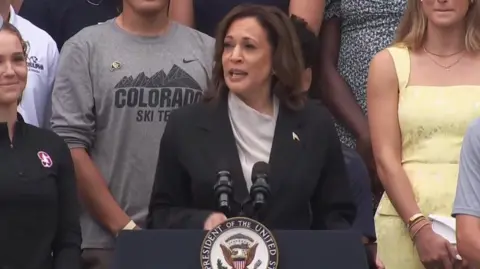\\\\\\n\\\\\\nBiden\'s legacy of accomplishment is unmatched - Harris\\\\\\n------------------------------------------------------\\\\\\n\\\\\\nThe US Vice-President praised Joe Biden\'s track record as US president.\\\\\\n\\\\\\n34 mins ago\\\\\\n\\\\\\nUS & Canada](/news/videos/c51y936y9g2o)\\n\\n* * *\\n\\nGlobal news\\n-----------\\n\\n[At least six killed in Croatia nursing home shooting\\\\\\n----------------------------------------------------\\\\\\n\\\\\\n7 mins ago\\\\\\n\\\\\\nEurope](/news/articles/cn08d7vyj6wo)\\n\\n[Israel orders evacuation of part of Gaza humanitarian zone\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\n\\n[Russian-US journalist jailed for \'false information\'\\\\\\n----------------------------------------------------\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nEurope](/news/articles/cn08d7j1qj5o)\\n\\n[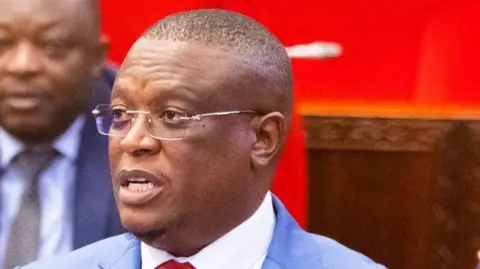\\\\\\n\\\\\\nTanzanian minister sacked after poll rigging remarks\\\\\\n----------------------------------------------------\\\\\\n\\\\\\nNape Nnauye caused outrage for saying he could help an MP rig elections - comments, he said, he made in jest.\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nAfrica](/news/articles/cd1e48677w0o)\\n\\n* * *\\n\\nUK news\\n-------\\n\\n[Campaigners in court over Magna Carta incident\\\\\\n----------------------------------------------\\\\\\n\\\\\\n54 mins ago\\\\\\n\\\\\\nUK](/news/articles/cxw29lg4y8go)\\n\\n[New Prince George photo released on 11th birthday\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n7 hrs ago\\\\\\n\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\n\\n[\'We have one of the best comedy scenes in the UK\'\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n12 hrs ago\\\\\\n\\\\\\nUK](/news/articles/c4ngrdpwlx3o)\\n\\n[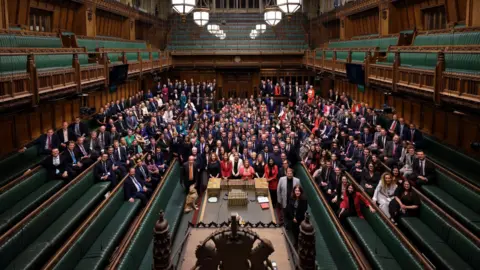\\\\\\n\\\\\\nHow does a surprise MP prepare for life in office?\\\\\\n--------------------------------------------------\\\\\\n\\\\\\nNew MPs in the East describe the experience of unexpectedly picking up the reins of public life.\\\\\\n\\\\\\n12 hrs ago\\\\\\n\\\\\\nEngland](/news/articles/cxr2g7rk3dxo)\\n\\n* * *\\n\\nSport\\n-----\\n\\n[Ferdinand\'s persuasive powers sealed Man Utd\'s Yoro deal\\\\\\n--------------------------------------------------------\\\\\\n\\\\\\n49 mins ago\\\\\\n\\\\\\nMan Utd](/sport/football/articles/c9e950ev0xpo)\\n\\n[Aston Villa complete \\u00a350m deal for Everton\'s Onana\\\\\\n--------------------------------------------------\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nPremier League](/sport/football/articles/cgrlgzx6gpro)\\n\\n[Australia would not pick convicted rapist Olympian\\\\\\n--------------------------------------------------\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nOlympic Games](/sport/articles/c2v0j0j6nqlo)\\n\\n[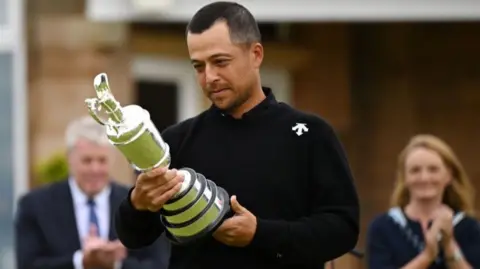\\\\\\n\\\\\\n\'Schauffele passes ultimate examination in classic Open\'\\\\\\n--------------------------------------------------------\\\\\\n\\\\\\nThe 152nd Open should be remembered as a classic, writes BBC golf correspondent Iain Carter.\\\\\\n\\\\\\n1 hr ago\\\\\\n\\\\\\nGolf](/sport/golf/articles/cv2g1glwypqo)\\n\\n* * *\\n\\n[Video\\\\\\n-----](https://www.bbc.com/video)\\n\\u00a0\\n\\n[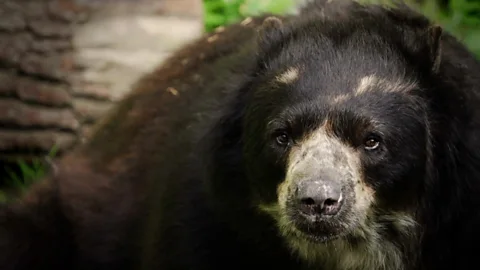\\\\\\n\\\\\\nThe \'Paddington bears\' facing climate threat\\\\\\n--------------------------------------------\\\\\\n\\\\\\nDrought forces the real Paddington Bear\\u00a0into deadly conflict with cattle farmers in the Andes.\\\\\\n\\\\\\nSee more](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\n\\n* * *\\n\\nBusiness\\n--------\\n\\n[Ryanair set to slash summer fares as profits drop\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n8 hrs ago\\\\\\n\\\\\\nBusiness](/news/articles/cj50d6q3jlro)\\n\\n[Former chancellor Zahawi mulling bid for the Telegraph\\\\\\n------------------------------------------------------\\\\\\n\\\\\\n26 mins ago\\\\\\n\\\\\\nBusiness](/news/articles/c4ng5q4jd62o)\\n\\n[Prime sued in trademark case by US Olympic committee\\\\\\n----------------------------------------------------\\\\\\n\\\\\\n1 day ago](/news/articles/c4ng785gjv0o)\\n\\n[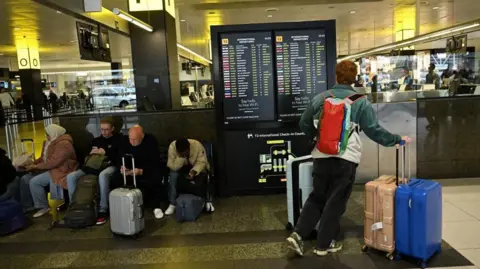\\\\\\n\\\\\\nCrowdStrike IT outage affected 8.5 million Windows devices, Microsoft says\\\\\\n--------------------------------------------------------------------------\\\\\\n\\\\\\nIt\\u2019s the first time that a number has been put on the glitch that is still causing problems around the world.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nTechnology](/news/articles/cpe3zgznwjno)\\n\\n* * *\\n\\nInnovation\\n----------\\n\\n[Company wins funding to make medicine in space\\\\\\n----------------------------------------------\\\\\\n\\\\\\n4 hrs ago](/news/articles/cp9vdxwjddeo)\\n\\n[Summer surge: why Covid-19 isn\'t yet seasonal\\\\\\n---------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\n\\n[Scam warning as fake emails and websites target users after outage\\\\\\n------------------------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nTechnology](/news/articles/cq5xy12pynyo)\\n\\n[\\\\\\n\\\\\\nAre fermented foods actually good for our health?\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nWhile humans have been eating fermented foods since ancient times, researchers are only starting to unravel some of the biggest questions about their health benefits.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\n\\n* * *\\n\\nCulture\\n-------\\n\\n[How to choose the most eco-friendly swimwear\\\\\\n--------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nCulture](/culture/article/20240719-how-to-choose-the-best-and-most-eco-friendly-swimwear)\\n\\n[In pictures: Colonial India through the eyes of foreign artists\\\\\\n---------------------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nAsia](/news/articles/c28ejl4nvgyo)\\n\\n[Auction for John Lennon glasses and Abbey Road photos\\\\\\n-----------------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nSurrey](/news/articles/cp085ym9l3ro)\\n\\n[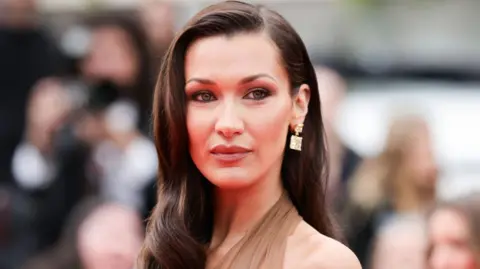\\\\\\n\\\\\\nBella Hadid\'s Adidas advert dropped after Israeli criticism\\\\\\n-----------------------------------------------------------\\\\\\n\\\\\\nThe model was chosen to promote shoes referencing the 1972 Munich Olympics, at which 11 Israeli athletes were killed.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nCulture](/news/articles/ceqdwpv8vw1o)\\n\\n* * *\\n\\nTravel\\n------\\n\\n[The Indian dish Kamala Harris loves\\\\\\n-----------------------------------\\\\\\n\\\\\\n1 hr ago\\\\\\n\\\\\\nTravel](/travel/article/20201026-dosa-indias-wholesome-fast-food-obsession)\\n\\n[Italy\'s most iconic trail reopens after 12 years\\\\\\n------------------------------------------------\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nTravel](/travel/article/20240722-cinque-terre-italys-path-of-love-reopens-after-12-years)\\n\\n[The US\'s little-known \'Ellis Island of the West\'\\\\\\n------------------------------------------------\\\\\\n\\\\\\n5 hrs ago\\\\\\n\\\\\\nTravel](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\n\\n[\\\\\\n\\\\\\nThe Turkish ice cream eaten with a knife and fork\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nDondurma isn\'t like any other ice cream you\'ll find, and the epicentre of its production is still reeling from the powerful earthquakes that decimated the nation.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\n\\n* * *\\n\\n[Travel\\\\\\n------](https://www.bbc.com/travel)\\n\\u00a0\\n\\n[\\\\\\n\\\\\\nThe US\'s little-known \'Ellis Island of the West\'\\\\\\n------------------------------------------------\\\\\\n\\\\\\nThis former quarantine and military station once processed as many as one million immigrants. Now, the picturesque island is one of the San Francisco Bay Area\'s best urban getaways.\\\\\\n\\\\\\nSee more](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\n\\n* * *\\n\\nSign up for our newsletters\\n---------------------------\\n\\n[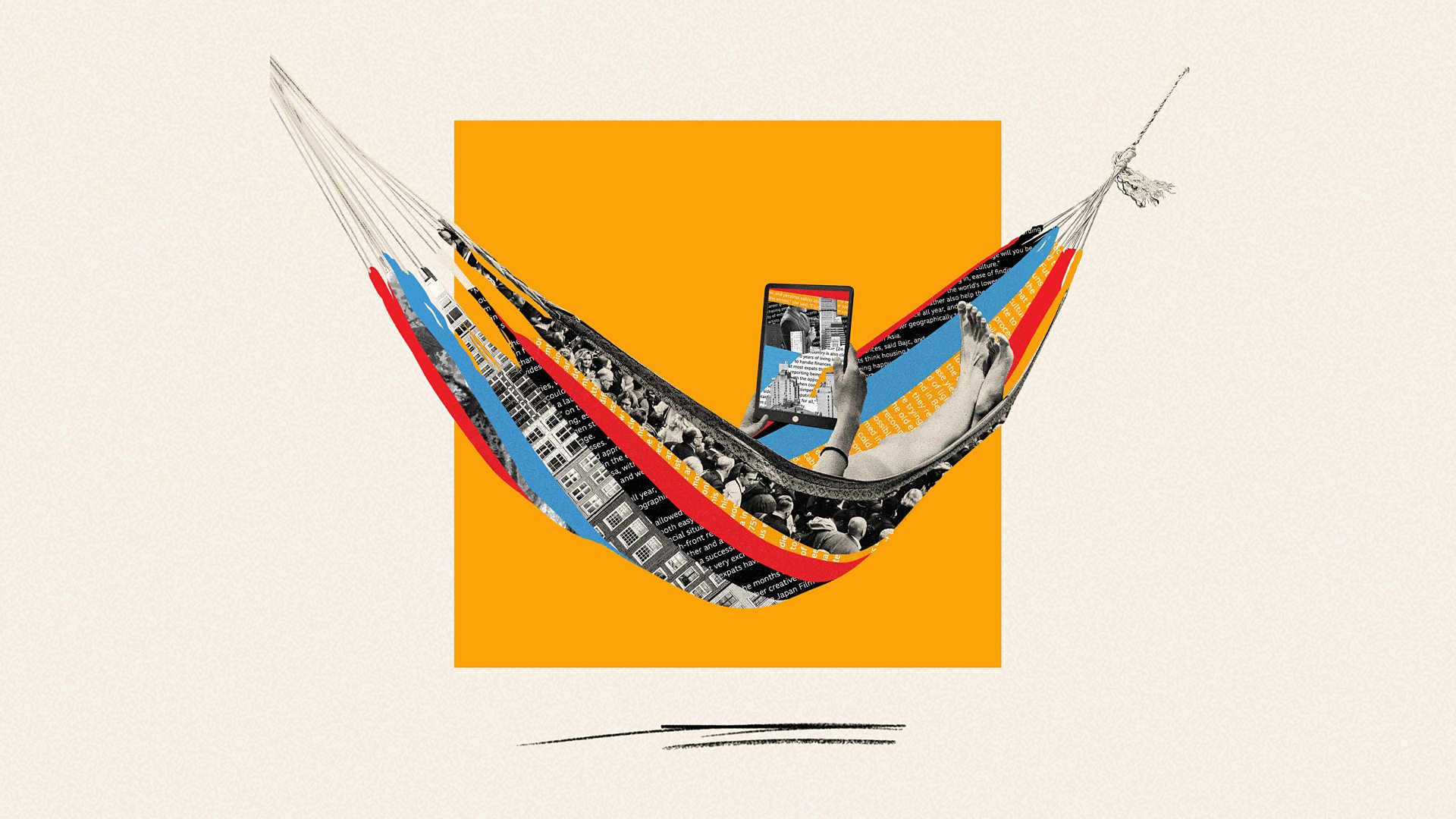\\\\\\n\\\\\\nThe Essential List\\\\\\n------------------\\\\\\n\\\\\\nThe week\'s best stories, handpicked by BBC editors, in your inbox every Tuesday and Friday.](https://cloud.email.bbc.com/SignUp10_08?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=essentiallist&at_campaign_type=owned)\\n\\n[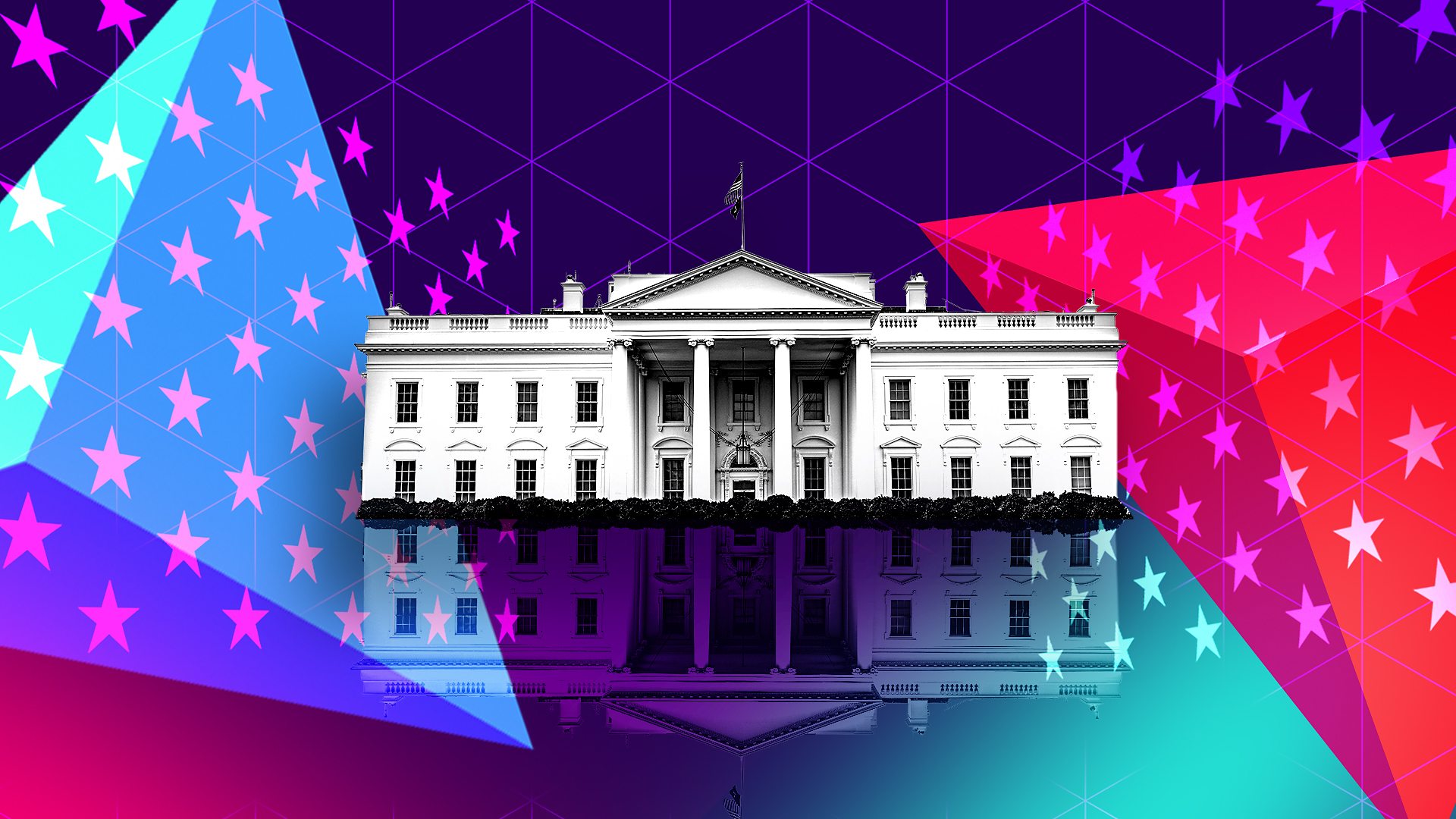\\\\\\n\\\\\\nUS Election Unspun\\\\\\n------------------\\\\\\n\\\\\\nCut through the spin with North America correspondent Anthony Zurcher - in your inbox every Wednesday.](https://cloud.email.bbc.com/US_Election_Unspun_newsletter_signup?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=uselectionunspun&at_campaign_type=owned)\\n\\n[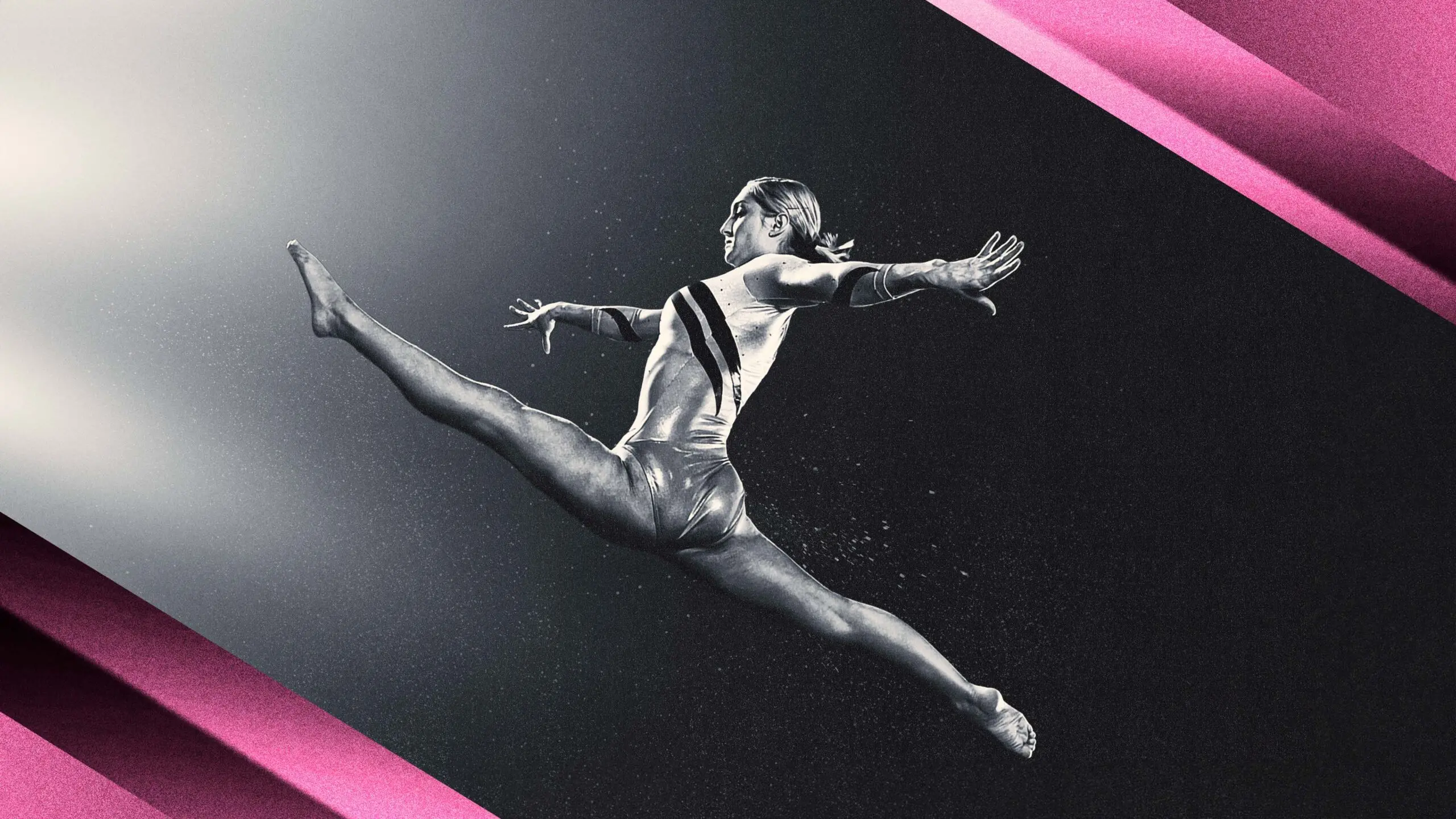\\\\\\n\\\\\\nMedal Moments\\\\\\n-------------\\\\\\n\\\\\\nYour global guide to the Paris Olympics, from key highlights to heroic stories, daily to your inbox throughout the Games.](https://cloud.email.bbc.com/medalmoments_newsletter_signup?&at_bbc_team=studios&at_medium=emails&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.com&at_format=Module&at_link_origin=homepage&at_campaign=olympics2024&at_campaign_id=&at_adset_name=&at_adset_id=&at_creation=&at_creative_id=&at_campaign_type=owned)\\n\\n* * *\\n\\nEarth\\n-----\\n\\n[The \'Paddington bears\' facing climate threat\\\\\\n--------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nNatural wonders](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\n\\n[The simple Japanese method for a tidier fridge\\\\\\n----------------------------------------------\\\\\\n\\\\\\n3 days ago\\\\\\n\\\\\\nFuture](/future/article/20240715-the-simple-japanese-method-for-a-tidier-and-less-wasteful-fridge)\\n\\n[Conspiracy theories swirl about geo-engineering, but could it help save the planet?\\\\\\n-----------------------------------------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nBBC InDepth](/news/articles/c98qp79gj4no)\\n\\n[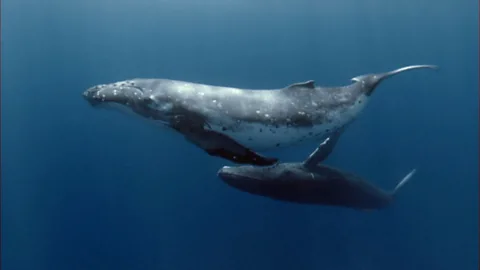\\\\\\n\\\\\\nFace to face with humpback whales\\\\\\n---------------------------------\\\\\\n\\\\\\nReece Parkinson discovers how locals are protecting their stunning marine environment.\\\\\\n\\\\\\n3 days ago\\\\\\n\\\\\\nTravel](/reel/video/p0jbpt60/face-to-face-with-humpback-whales)\\n\\n* * *\\n\\nScience and health\\n------------------\\n\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\n-----------------------------------------------------\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\n\\n[Are fermented foods actually good for our health?\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\n\\n[Summer surge: why Covid-19 isn\'t yet seasonal\\\\\\n---------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\n\\n[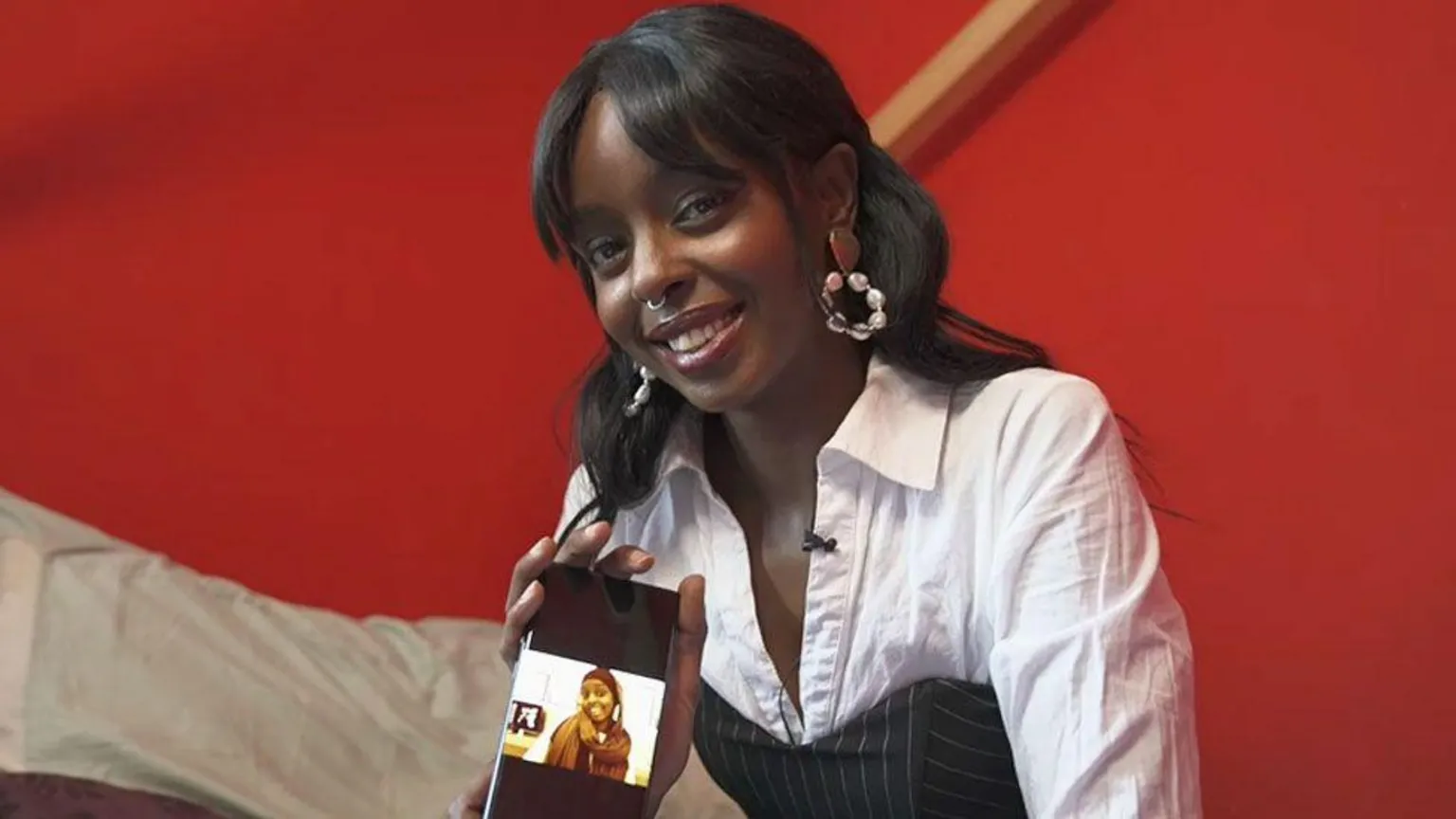\\\\\\n\\\\\\n\'A way to fight back\': FGM survivors reclaim vulvas with surgery\\\\\\n----------------------------------------------------------------\\\\\\n\\\\\\nIn Somalia, it\'s believed cutting off a girl\'s outer genitalia will guarantee their virginity.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nAfrica](/news/articles/cyx0perl8yno)\\n\\n* * *\\n\\nWorld\\u2019s Table\\n-------------\\n\\n[The Turkish ice cream eaten with a knife and fork\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\n\\n[India\'s cooling drinks to beat the heat\\\\\\n---------------------------------------\\\\\\n\\\\\\n7 days ago\\\\\\n\\\\\\nTravel](/travel/article/20240715-gond-katira-a-natural-way-to-cool-down-in-indias-scorching-summers)\\n\\n[The world\'s biggest restaurant is coming to Paris\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n9 Jul 2024\\\\\\n\\\\\\nTravel](/travel/article/20240708-paris-olympics-2024-worlds-biggest-restaurant)\\n\\n[\\\\\\n\\\\\\nThe wild ceremonies of the Turkish \'meatball\'\\\\\\n---------------------------------------------\\\\\\n\\\\\\nOne of the country\'s most popular fast-food items, \\u00e7i\\u011f k\\u00f6fte is traditionally associated with wild and rowdy gatherings in south-eastern Turkey.\\\\\\n\\\\\\n1 Jun 2024\\\\\\n\\\\\\nTravel](/travel/article/20240531-the-wild-ceremonies-surrounding-a-turkish-meatball)\\n\\n* * *\\n\\nThe Specialist\\n--------------\\n\\n[Guide to Helsinki\'s happiest places\\\\\\n-----------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nTravel](/travel/article/20240718-a-happiness-hackers-guide-to-the-happiest-outdoor-places-in-helsinki)\\n\\n[A pastry chef\'s favourite bakeries in Paris\\\\\\n-------------------------------------------\\\\\\n\\\\\\n5 days ago\\\\\\n\\\\\\nTravel](/travel/article/20240717-david-lebovitzs-ultimate-guide-to-the-best-bakeries-in-paris-right-now)\\n\\n[Chef Andrew Zimmern\'s favourite US restaurants\\\\\\n----------------------------------------------\\\\\\n\\\\\\n14 Jul 2024\\\\\\n\\\\\\nTravel](/travel/article/20240714-chef-andrew-zimmerns-favourite-us-restaurants)\\n\\n[\\\\\\n\\\\\\nA First Lady\'s guide to Iceland\\\\\\n-------------------------------\\\\\\n\\\\\\nEliza Reid moved to Iceland 20 years ago for love and now she\'s the First Lady. Here are her favourite ways to enjoy a \\"chill\\" Icelandic weekend.\\\\\\n\\\\\\n11 Jul 2024\\\\\\n\\\\\\nTravel](/travel/article/20240710-icelands-first-lady-takes-you-on-a-tour-of-her-super-chill-nation)\\n\\n* * *\\n\\n[British Broadcasting Corporation](/)\\n\\n* [Home](https://www.bbc.com/)\\n \\n* [News](/news)\\n \\n* [Sport](/sport)\\n \\n* [Business](/business)\\n \\n* [Innovation](/innovation)\\n \\n* [Culture](/culture)\\n \\n* [Travel](/travel)\\n \\n* [Earth](/future-planet)\\n \\n* [Video](/video)\\n \\n* [Live](/live)\\n \\n* [Audio](https://www.bbc.co.uk/sounds)\\n \\n* [Weather](https://www.bbc.com/weather)\\n \\n* [BBC Shop](https://shop.bbc.com/)\\n \\n\\nBBC in other languages\\n\\nFollow BBC on:\\n--------------\\n\\n* [Terms of Use](https://www.bbc.co.uk/usingthebbc/terms)\\n \\n* [About the BBC](https://www.bbc.co.uk/aboutthebbc)\\n \\n* [Privacy Policy](https://www.bbc.com/usingthebbc/privacy/)\\n \\n* [Cookies](https://www.bbc.com/usingthebbc/cookies/)\\n \\n* [Accessibility Help](https://www.bbc.co.uk/accessibility/)\\n \\n* [Contact the BBC](https://www.bbc.co.uk/contact)\\n \\n* [Advertise with us](https://www.bbc.com/advertisingcontact)\\n \\n* [Do not share or sell my info](https://www.bbc.com/usingthebbc/cookies/how-can-i-change-my-bbc-cookie-settings/)\\n \\n* [Contact technical support](https://www.bbc.com/contact-bbc-com-help)\\n \\n\\nCopyright 2024 BBC. All rights reserved.\\u00a0\\u00a0The _BBC_ is _not responsible for the content of external sites._\\u00a0[**Read about our approach to external linking.**](https://www.bbc.co.uk/editorialguidelines/guidance/feeds-and-links)", "markdown": "\\n\\n[British Broadcasting Corporation](/)\\n\\n[Watch Live](/watch-live-news)\\n\\nRegisterSign In\\n\\n* [Home](/)\\n \\n* [News](/news)\\n \\n* [Sport](/sport)\\n \\n* [Business](/business)\\n \\n* [Innovation](/innovation)\\n \\n* [Culture](/culture)\\n \\n* [Travel](/travel)\\n \\n* [Earth](/future-planet)\\n \\n* [Video](/video)\\n \\n* [Live](/live)\\n \\n\\nRegisterSign In\\n\\n[Home](/)\\n\\nNews\\n\\n[Sport](/sport)\\n\\nBusiness\\n\\nInnovation\\n\\nCulture\\n\\nTravel\\n\\nEarth\\n\\n[Video](/video)\\n\\nLive\\n\\n[Audio](https://www.bbc.co.uk/sounds)\\n\\n[Weather](https://www.bbc.com/weather)\\n\\n[Newsletters](https://www.bbc.com/newsletters)\\n\\n[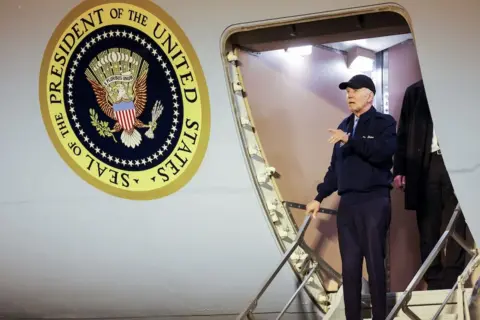\\\\\\n\\\\\\nThe last days of the Biden campaign \\u2013 BBC correspondent\\u2019s account\\\\\\n-----------------------------------------------------------------\\\\\\n\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\'s campaign.\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\n\\n[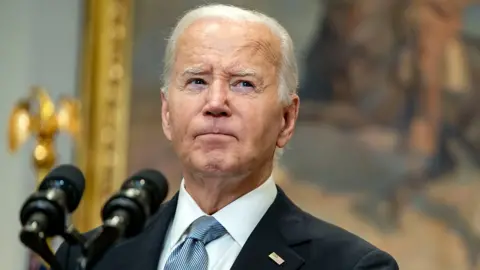\\\\\\n\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\n----------------------------------------------------------------------\\\\\\n\\\\\\nMost of the president\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\n\\\\\\n15 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\n\\n[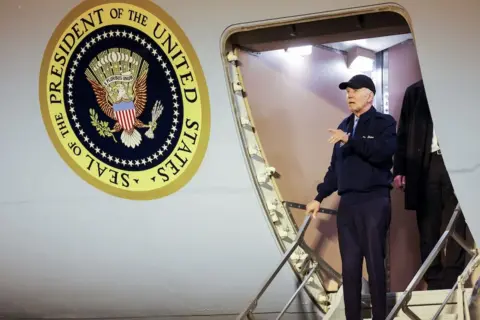\\\\\\n\\\\\\nThe last days of the Biden campaign \\u2013 BBC correspondent\\u2019s account\\\\\\n-----------------------------------------------------------------\\\\\\n\\\\\\nBBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\'s campaign.\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c250zqgrpqgo)\\n\\n[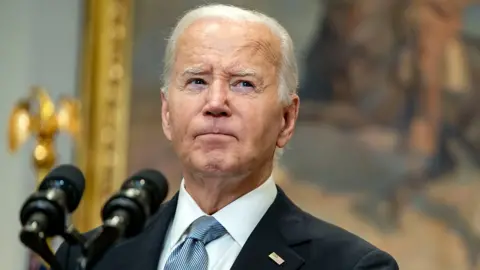\\\\\\n\\\\\\nIsolating at a beach house, Biden gave aides one minute notice of exit\\\\\\n----------------------------------------------------------------------\\\\\\n\\\\\\nMost of the president\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.\\\\\\n\\\\\\n15 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c3gdzmdje5xo)\\n\\n[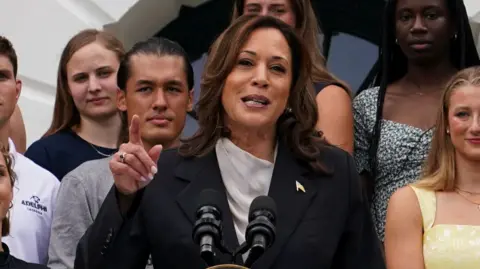\\\\\\n\\\\\\nLIVE\\\\\\n\\\\\\nKamala Harris speaks for first time since Biden left race - as endorsements mount\\\\\\n---------------------------------------------------------------------------------\\\\\\n\\\\\\nThe US vice-president appears at the White House for a pre-scheduled event - as key Democrats line up to back her candidacy.](https://www.bbc.com/news/live/cv2gryx1yx1t)\\n\\n* * *\\n\\n[What Biden quitting means for Harris, the Democrats and Trump\\\\\\n-------------------------------------------------------------\\\\\\n\\\\\\nPresident Biden has upended the 2024 White House race for the Democrats. Here is what it means for Kamala Harris, his party and Trump.\\\\\\n\\\\\\n19 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/cpwd8yzw45qo)\\n\\n[Who could be Kamala Harris\'s running mate?\\\\\\n------------------------------------------\\\\\\n\\\\\\nIt\\u2019s not a done deal but some potential rivals have quickly thrown their support behind her.\\\\\\n\\\\\\n10 mins ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\n\\n[LIVE\\\\\\n\\\\\\nTensions flare as Congress presses Secret Service boss on \'failed\' Trump rally security\\\\\\n---------------------------------------------------------------------------------------\\\\\\n\\\\\\nMembers of both parties have called for Kimberly Cheatle to resign in a House committee hearing that is seeking answers over security failures at the rally on 13 July.](https://www.bbc.com/news/live/c724wqpy4qnt)\\n\\n[The president\'s protectors are hardly noticeable - until things go wrong\\\\\\n------------------------------------------------------------------------\\\\\\n\\\\\\nThe attempted assassination of Donald Trump has raised questions about the Secret Service\'s record.\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c16j896003xo)\\n\\n* * *\\n\\nOnly from the BBC\\n-----------------\\n\\n[\\\\\\n\\\\\\nWhy you are probably sitting for too long\\\\\\n-----------------------------------------\\\\\\n\\\\\\nSitting down is ingrained in most peoples\' days. But staying sedentary for too long can increase the risk of serious health conditions like cardiovascular disease and type 2 diabetes.\\\\\\n\\\\\\n4 hrs ago\\\\\\n\\\\\\nFuture](/future/article/20240722-why-you-are-probably-sitting-down-for-too-long)\\n\\n[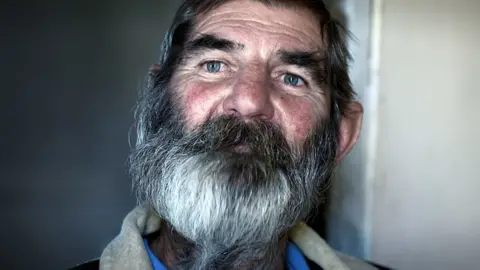\\\\\\n\\\\\\nMass killer who \\u2018hunted\\u2019 black people says police encouraged him\\\\\\n----------------------------------------------------------------\\\\\\n\\\\\\nEx-security guard, Louis van Schoor, killed dozens in South Africa but was only jailed for seven murders.\\\\\\n\\\\\\n17 hrs ago\\\\\\n\\\\\\nAfrica](/news/articles/c51yqdy3q61o)\\n\\n* * *\\n\\n[More news\\\\\\n---------](https://www.bbc.com/news)\\n\\u00a0\\n\\n[\\\\\\n\\\\\\nUAE jails 57 Bangladeshis over protests against own government\\\\\\n--------------------------------------------------------------\\\\\\n\\\\\\nThree defendants were sentenced to life after being convicted of \\"inciting riots\\" in the Gulf state.\\\\\\n\\\\\\n5 hrs ago\\\\\\n\\\\\\nWorld](/news/articles/crgk8gnpg0zo)\\n\\n[\\\\\\n\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\n\\n[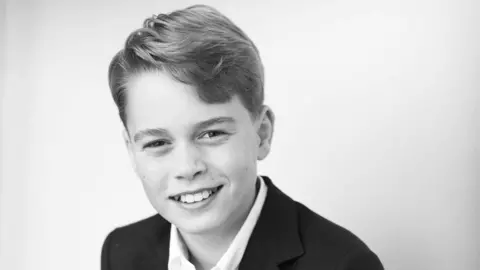\\\\\\n\\\\\\nNew Prince George photo released on 11th birthday\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\n\\\\\\n7 hrs ago\\\\\\n\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\n\\n[Piastri wins in Hungary after Norris team orders row\\\\\\n----------------------------------------------------\\\\\\n\\\\\\nOscar Piastri takes his maiden grand prix victory ahead of McLaren team-mate Lando Norris in a dramatic race in Hungary.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nFormula 1](/sport/formula1/articles/cg3jzy3e8q1o)\\n\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\n-----------------------------------------------------\\\\\\n\\\\\\nThe virus is transmitted from animals such as pigs and fruit bats to humans.\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\n\\n[\\\\\\n\\\\\\nIsrael orders evacuation of part of Gaza humanitarian zone\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\nStrikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\n\\n[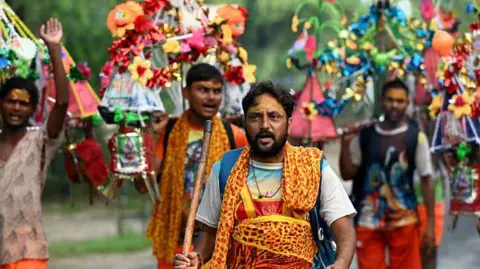\\\\\\n\\\\\\nIndia court blocks order for eateries to display owners\' names\\\\\\n--------------------------------------------------------------\\\\\\n\\\\\\nCritics say ordering restaurants to prominently display names of owners is discriminatory towards Muslims.\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/czrj18yp489o)\\n\\n[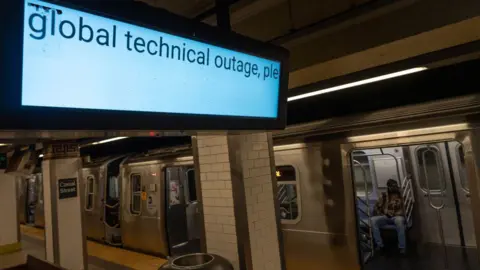\\\\\\n\\\\\\n\'Significant number\' of devices fixed - CrowdStrike\\\\\\n---------------------------------------------------\\\\\\n\\\\\\nCybersecurity firm behind global outage says it continues to focus on restoring all impacted computers.\\\\\\n\\\\\\n13 hrs ago\\\\\\n\\\\\\nBusiness](/news/articles/cgl7e33n1d0o)\\n\\n[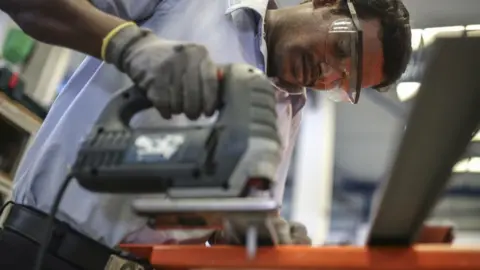\\\\\\n\\\\\\nModi\'s new budget faces jobs crisis test in India\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nMr Modi\'s biggest challenge in his third term will be bridging the rich-poor divide.\\\\\\n\\\\\\n16 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/cq5jwyel12qo)\\n\\n[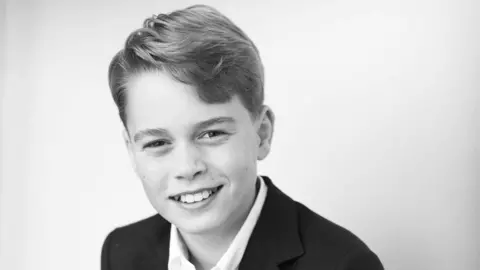\\\\\\n\\\\\\nNew Prince George photo released on 11th birthday\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nKensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.\\\\\\n\\\\\\n7 hrs ago\\\\\\n\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\n\\n* * *\\n\\nMust watch\\n----------\\n\\n[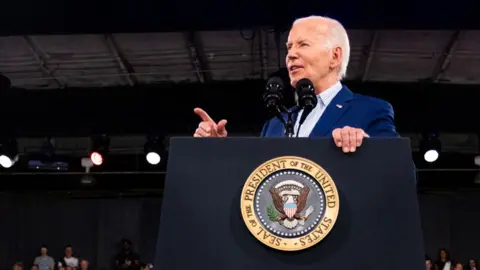\\\\\\n\\\\\\nBiden\\u2019s disastrous few weeks... in 90 seconds\\\\\\n---------------------------------------------\\\\\\n\\\\\\nPresident Biden has faced intense pressure to step aside since his faltering debate performance.\\\\\\n\\\\\\n17 hrs ago\\\\\\n\\\\\\nUS & Canada](/news/videos/cx028eq4qg1o)\\n\\n[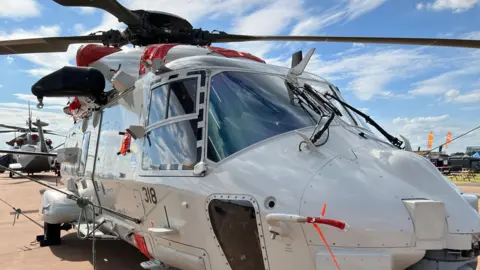\\\\\\n\\\\\\nInside the Netherlands Navy\'s anti-submarine helicopter\\\\\\n-------------------------------------------------------\\\\\\n\\\\\\nThe NH90 was on display at the 2024 Royal International Air Tattoo show at RAF Fairford.\\\\\\n\\\\\\n4 hrs ago\\\\\\n\\\\\\nEngland](/news/videos/cmj24x03r8po)\\n\\n[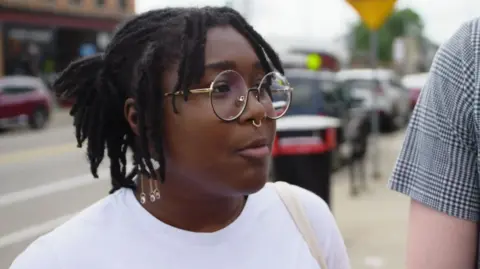\\\\\\n\\\\\\nDemocrats in Michigan react to Biden dropping out\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nDemocratic voters in Michigan give their take on Joe Biden withdrawing from the US presidential race.\\\\\\n\\\\\\n18 hrs ago](/news/videos/c51yrr2z74no)\\n\\n[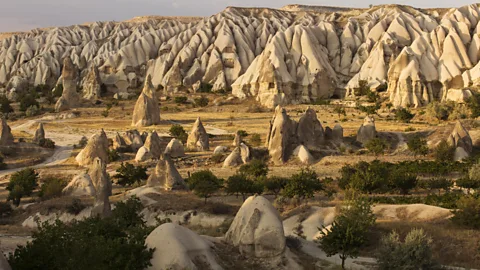\\\\\\n\\\\\\nTurkey\'s answer to \'Burning Man\'\\\\\\n--------------------------------\\\\\\n\\\\\\nA music and art extravaganza takes place in an \'otherworldly\' landscape amid unique volcanic rock formations.\\\\\\n\\\\\\n13 hrs ago\\\\\\n\\\\\\nCulture & Experiences](/reel/video/p0jch19q/turkey-s-answer-to-burning-man-)\\n\\n[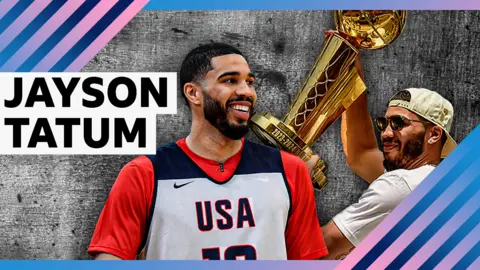\\\\\\n\\\\\\nTatum on handling criticism and \'joy\' of Olympics\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nTeam USA and Boston Celtics power forward Jayson Tatum says \\"basketball can\'t be your sole purpose\\" as he speaks about facing criticism, and the \\"joy\\" that playing in the Olympics brings.\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nOlympic Games](/sport/basketball/videos/cg640yk3n3zo)\\n\\n[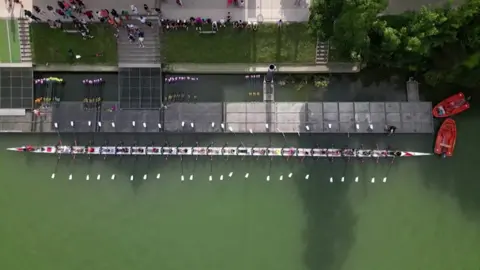\\\\\\n\\\\\\nWorld\'s longest rowing boat to carry Olympic torch\\\\\\n--------------------------------------------------\\\\\\n\\\\\\nThe 24-seater boat will take the Olympic torch down a section of the River Marne on Sunday.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nEurope](/news/videos/cqe6q917y1jo)\\n\\n[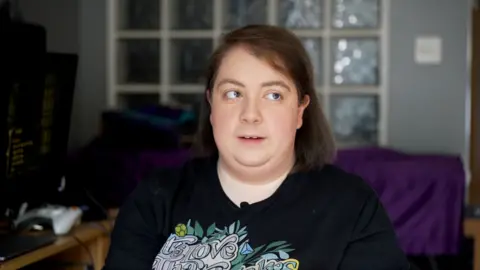\\\\\\n\\\\\\nAccessibility brings disabled gamers a sense of community\\\\\\n---------------------------------------------------------\\\\\\n\\\\\\nGreater accessibility in game development is opening the genre to more people with disabilities.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nScotland](/news/videos/c0jq593xqk8o)\\n\\n[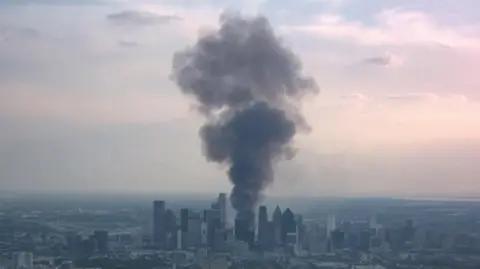\\\\\\n\\\\\\nWatch: Spire collapses as fire engulfs Texas church\\\\\\n---------------------------------------------------\\\\\\n\\\\\\nA blaze at a historic church in Dallas has caused huge plumes of smoke to rise over the Texan city\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nUS & Canada](/news/videos/cjk325014g7o)\\n\\n* * *\\n\\nIn History\\n----------\\n\\n[\\\\\\n\\\\\\n\'He was after my life\': WW1 soldier\'s confession\\\\\\n------------------------------------------------\\\\\\n\\\\\\nWorld War One broke out on 28 July, 1914. Fifty years later, one of the German soldiers, Stefan Westmann, told the BBC about his experiences fighting in the conflict.\\\\\\n\\\\\\nSee more](/culture/article/20240718-a-ww1-soldier-on-the-brutality-of-conflict)\\n\\n* * *\\n\\n[Olympic Games\\\\\\n-------------](https://www.bbc.com/sport/olympics)\\n\\u00a0\\n\\n[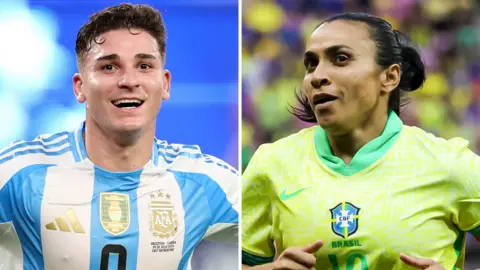\\\\\\n\\\\\\nTen footballers to watch out for at Paris Olympics\\\\\\n--------------------------------------------------\\\\\\n\\\\\\nFrom Manchester City\'s Julian Alvarez to Brazil icon Marta, BBC Sport picks out 10 footballers to watch at the Olympics.\\\\\\n\\\\\\nSee more](/sport/football/articles/cek91m98g48o)\\n\\n* * *\\n\\nUS and Canada news\\n------------------\\n\\n[\'The right move but is it too late?\' Democratic voters react\\\\\\n------------------------------------------------------------\\\\\\n\\\\\\nJust now\\\\\\n\\\\\\nUS & Canada](/news/articles/crgk8rgm87lo)\\n\\n[Who could be Kamala Harris\'s running mate?\\\\\\n------------------------------------------\\\\\\n\\\\\\n10 mins ago\\\\\\n\\\\\\nUS & Canada](/news/articles/c80ekdwk9zro)\\n\\n[Biden has backed Harris. What happens next in US election?\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\n18 mins ago\\\\\\n\\\\\\nUS & Canada](/news/articles/cq5xdq71drro)\\n\\n[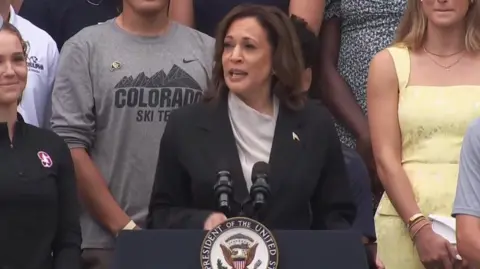\\\\\\n\\\\\\nBiden\'s legacy of accomplishment is unmatched - Harris\\\\\\n------------------------------------------------------\\\\\\n\\\\\\nThe US Vice-President praised Joe Biden\'s track record as US president.\\\\\\n\\\\\\n34 mins ago\\\\\\n\\\\\\nUS & Canada](/news/videos/c51y936y9g2o)\\n\\n* * *\\n\\nGlobal news\\n-----------\\n\\n[At least six killed in Croatia nursing home shooting\\\\\\n----------------------------------------------------\\\\\\n\\\\\\n7 mins ago\\\\\\n\\\\\\nEurope](/news/articles/cn08d7vyj6wo)\\n\\n[Israel orders evacuation of part of Gaza humanitarian zone\\\\\\n----------------------------------------------------------\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nMiddle East](/news/articles/cgerz8we1vgo)\\n\\n[Russian-US journalist jailed for \'false information\'\\\\\\n----------------------------------------------------\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nEurope](/news/articles/cn08d7j1qj5o)\\n\\n[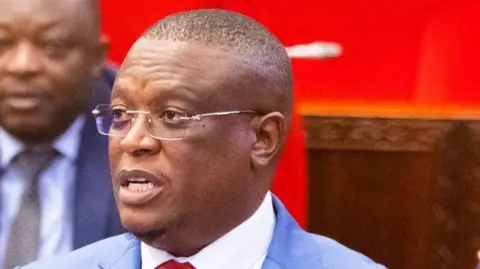\\\\\\n\\\\\\nTanzanian minister sacked after poll rigging remarks\\\\\\n----------------------------------------------------\\\\\\n\\\\\\nNape Nnauye caused outrage for saying he could help an MP rig elections - comments, he said, he made in jest.\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nAfrica](/news/articles/cd1e48677w0o)\\n\\n* * *\\n\\nUK news\\n-------\\n\\n[Campaigners in court over Magna Carta incident\\\\\\n----------------------------------------------\\\\\\n\\\\\\n54 mins ago\\\\\\n\\\\\\nUK](/news/articles/cxw29lg4y8go)\\n\\n[New Prince George photo released on 11th birthday\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n7 hrs ago\\\\\\n\\\\\\nUK](/news/articles/cw4yxd3dw7qo)\\n\\n[\'We have one of the best comedy scenes in the UK\'\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n12 hrs ago\\\\\\n\\\\\\nUK](/news/articles/c4ngrdpwlx3o)\\n\\n[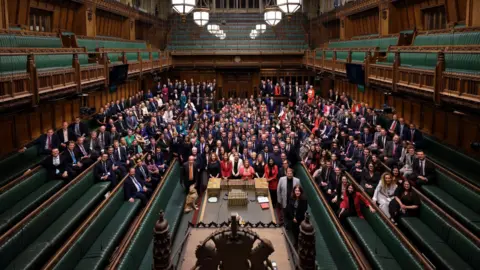\\\\\\n\\\\\\nHow does a surprise MP prepare for life in office?\\\\\\n--------------------------------------------------\\\\\\n\\\\\\nNew MPs in the East describe the experience of unexpectedly picking up the reins of public life.\\\\\\n\\\\\\n12 hrs ago\\\\\\n\\\\\\nEngland](/news/articles/cxr2g7rk3dxo)\\n\\n* * *\\n\\nSport\\n-----\\n\\n[Ferdinand\'s persuasive powers sealed Man Utd\'s Yoro deal\\\\\\n--------------------------------------------------------\\\\\\n\\\\\\n49 mins ago\\\\\\n\\\\\\nMan Utd](/sport/football/articles/c9e950ev0xpo)\\n\\n[Aston Villa complete \\u00a350m deal for Everton\'s Onana\\\\\\n--------------------------------------------------\\\\\\n\\\\\\n2 hrs ago\\\\\\n\\\\\\nPremier League](/sport/football/articles/cgrlgzx6gpro)\\n\\n[Australia would not pick convicted rapist Olympian\\\\\\n--------------------------------------------------\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nOlympic Games](/sport/articles/c2v0j0j6nqlo)\\n\\n[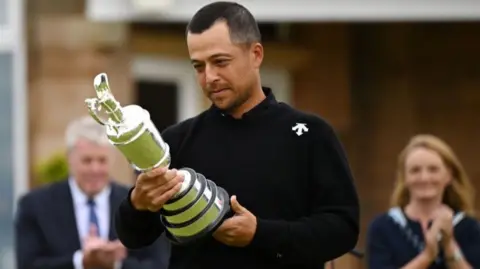\\\\\\n\\\\\\n\'Schauffele passes ultimate examination in classic Open\'\\\\\\n--------------------------------------------------------\\\\\\n\\\\\\nThe 152nd Open should be remembered as a classic, writes BBC golf correspondent Iain Carter.\\\\\\n\\\\\\n1 hr ago\\\\\\n\\\\\\nGolf](/sport/golf/articles/cv2g1glwypqo)\\n\\n* * *\\n\\n[Video\\\\\\n-----](https://www.bbc.com/video)\\n\\u00a0\\n\\n[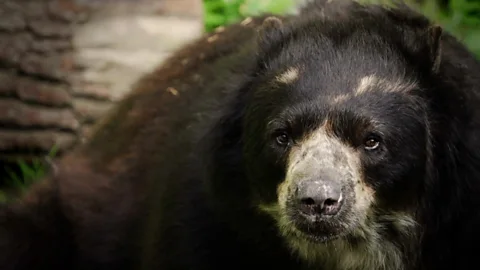\\\\\\n\\\\\\nThe \'Paddington bears\' facing climate threat\\\\\\n--------------------------------------------\\\\\\n\\\\\\nDrought forces the real Paddington Bear\\u00a0into deadly conflict with cattle farmers in the Andes.\\\\\\n\\\\\\nSee more](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\n\\n* * *\\n\\nBusiness\\n--------\\n\\n[Ryanair set to slash summer fares as profits drop\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n8 hrs ago\\\\\\n\\\\\\nBusiness](/news/articles/cj50d6q3jlro)\\n\\n[Former chancellor Zahawi mulling bid for the Telegraph\\\\\\n------------------------------------------------------\\\\\\n\\\\\\n26 mins ago\\\\\\n\\\\\\nBusiness](/news/articles/c4ng5q4jd62o)\\n\\n[Prime sued in trademark case by US Olympic committee\\\\\\n----------------------------------------------------\\\\\\n\\\\\\n1 day ago](/news/articles/c4ng785gjv0o)\\n\\n[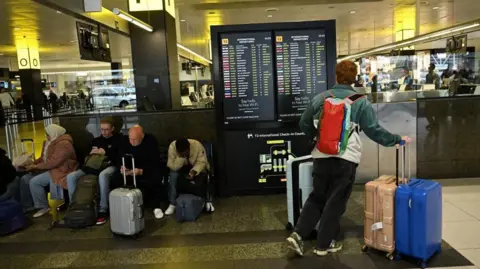\\\\\\n\\\\\\nCrowdStrike IT outage affected 8.5 million Windows devices, Microsoft says\\\\\\n--------------------------------------------------------------------------\\\\\\n\\\\\\nIt\\u2019s the first time that a number has been put on the glitch that is still causing problems around the world.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nTechnology](/news/articles/cpe3zgznwjno)\\n\\n* * *\\n\\nInnovation\\n----------\\n\\n[Company wins funding to make medicine in space\\\\\\n----------------------------------------------\\\\\\n\\\\\\n4 hrs ago](/news/articles/cp9vdxwjddeo)\\n\\n[Summer surge: why Covid-19 isn\'t yet seasonal\\\\\\n---------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\n\\n[Scam warning as fake emails and websites target users after outage\\\\\\n------------------------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nTechnology](/news/articles/cq5xy12pynyo)\\n\\n[\\\\\\n\\\\\\nAre fermented foods actually good for our health?\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nWhile humans have been eating fermented foods since ancient times, researchers are only starting to unravel some of the biggest questions about their health benefits.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\n\\n* * *\\n\\nCulture\\n-------\\n\\n[How to choose the most eco-friendly swimwear\\\\\\n--------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nCulture](/culture/article/20240719-how-to-choose-the-best-and-most-eco-friendly-swimwear)\\n\\n[In pictures: Colonial India through the eyes of foreign artists\\\\\\n---------------------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nAsia](/news/articles/c28ejl4nvgyo)\\n\\n[Auction for John Lennon glasses and Abbey Road photos\\\\\\n-----------------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nSurrey](/news/articles/cp085ym9l3ro)\\n\\n[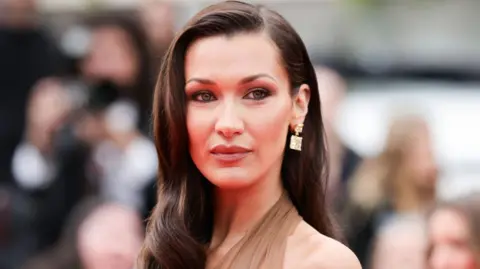\\\\\\n\\\\\\nBella Hadid\'s Adidas advert dropped after Israeli criticism\\\\\\n-----------------------------------------------------------\\\\\\n\\\\\\nThe model was chosen to promote shoes referencing the 1972 Munich Olympics, at which 11 Israeli athletes were killed.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nCulture](/news/articles/ceqdwpv8vw1o)\\n\\n* * *\\n\\nTravel\\n------\\n\\n[The Indian dish Kamala Harris loves\\\\\\n-----------------------------------\\\\\\n\\\\\\n1 hr ago\\\\\\n\\\\\\nTravel](/travel/article/20201026-dosa-indias-wholesome-fast-food-obsession)\\n\\n[Italy\'s most iconic trail reopens after 12 years\\\\\\n------------------------------------------------\\\\\\n\\\\\\n3 hrs ago\\\\\\n\\\\\\nTravel](/travel/article/20240722-cinque-terre-italys-path-of-love-reopens-after-12-years)\\n\\n[The US\'s little-known \'Ellis Island of the West\'\\\\\\n------------------------------------------------\\\\\\n\\\\\\n5 hrs ago\\\\\\n\\\\\\nTravel](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\n\\n[\\\\\\n\\\\\\nThe Turkish ice cream eaten with a knife and fork\\\\\\n-------------------------------------------------\\\\\\n\\\\\\nDondurma isn\'t like any other ice cream you\'ll find, and the epicentre of its production is still reeling from the powerful earthquakes that decimated the nation.\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\n\\n* * *\\n\\n[Travel\\\\\\n------](https://www.bbc.com/travel)\\n\\u00a0\\n\\n[\\\\\\n\\\\\\nThe US\'s little-known \'Ellis Island of the West\'\\\\\\n------------------------------------------------\\\\\\n\\\\\\nThis former quarantine and military station once processed as many as one million immigrants. Now, the picturesque island is one of the San Francisco Bay Area\'s best urban getaways.\\\\\\n\\\\\\nSee more](/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us)\\n\\n* * *\\n\\nSign up for our newsletters\\n---------------------------\\n\\n[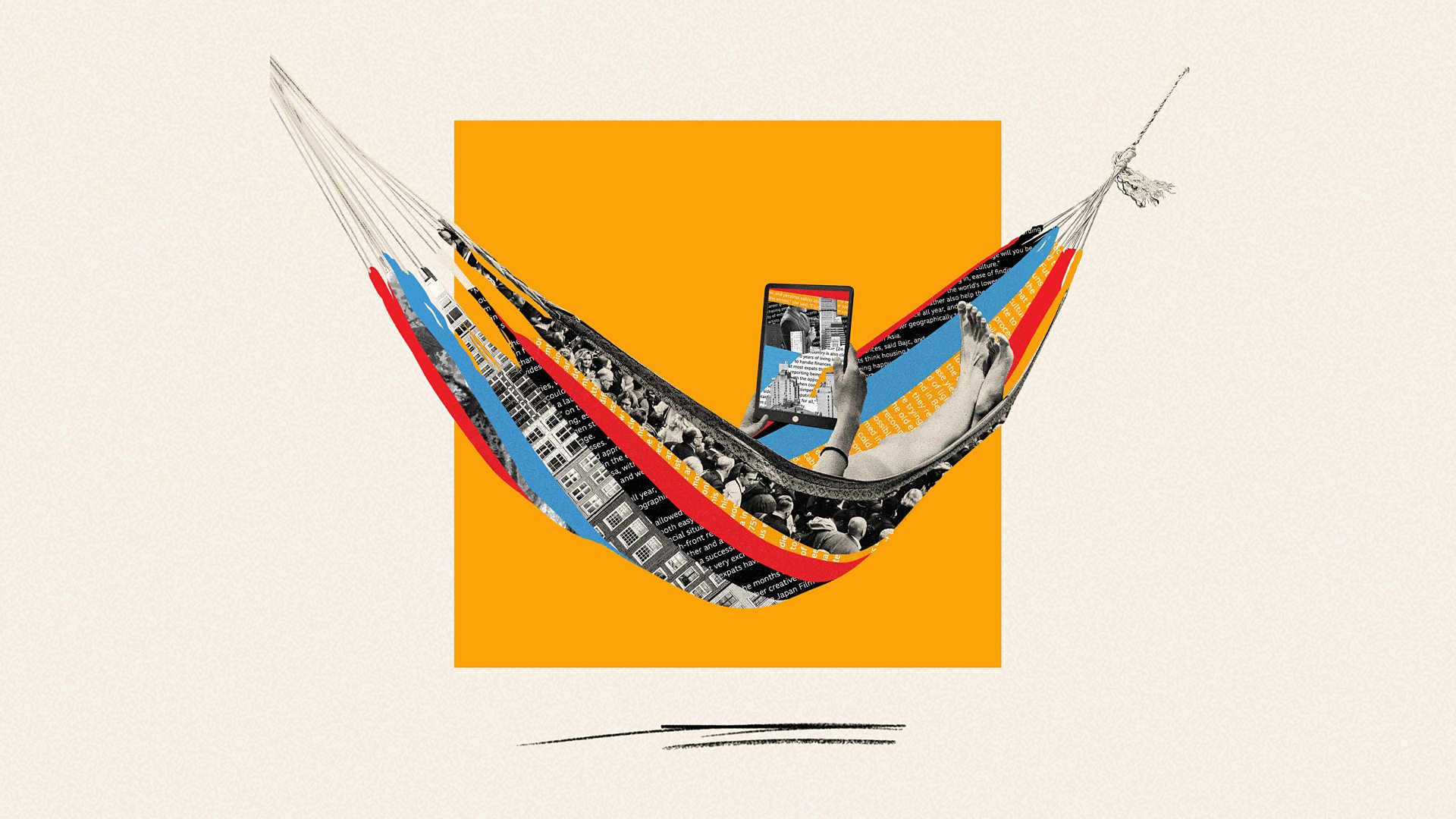\\\\\\n\\\\\\nThe Essential List\\\\\\n------------------\\\\\\n\\\\\\nThe week\'s best stories, handpicked by BBC editors, in your inbox every Tuesday and Friday.](https://cloud.email.bbc.com/SignUp10_08?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=essentiallist&at_campaign_type=owned)\\n\\n[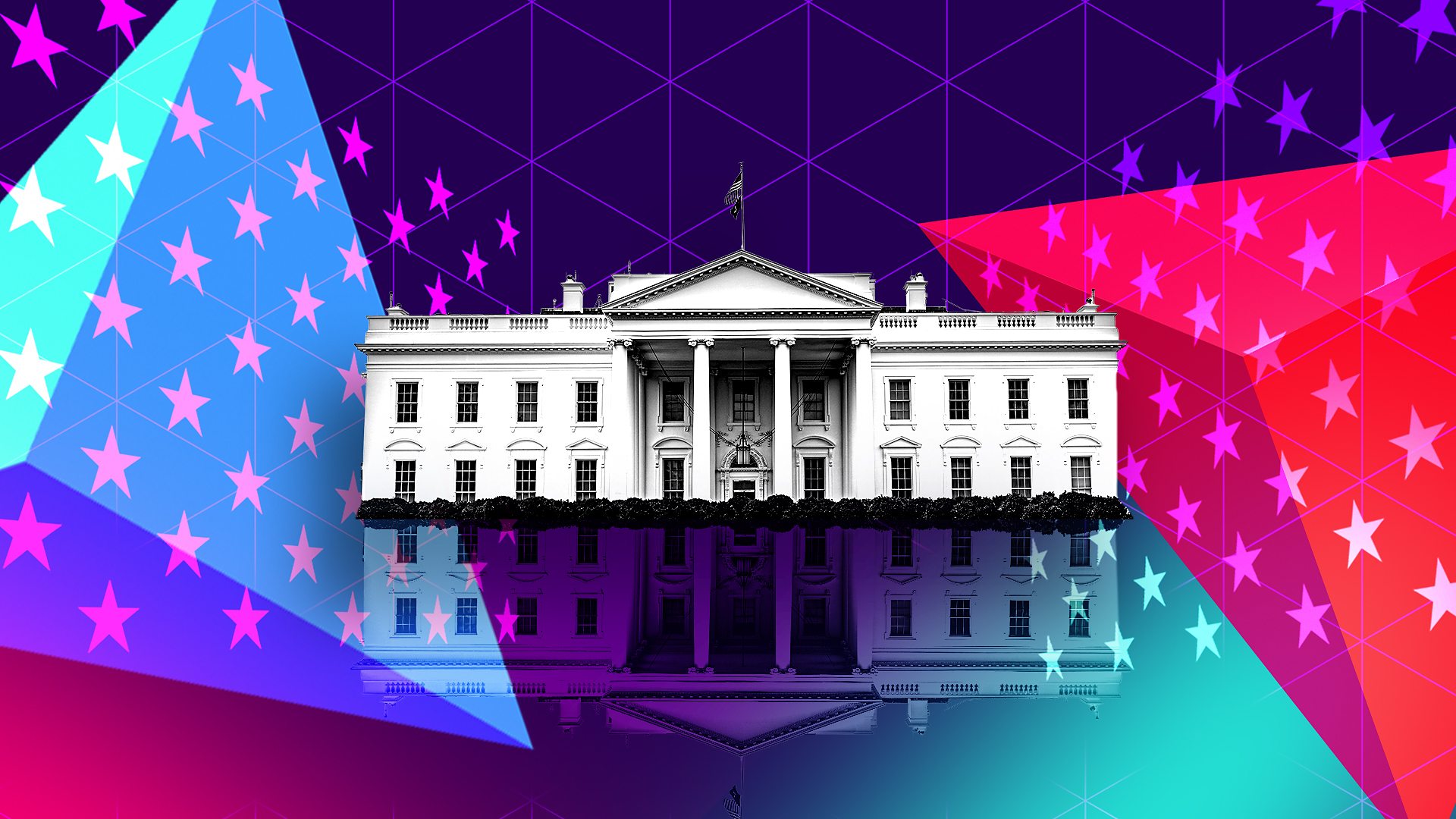\\\\\\n\\\\\\nUS Election Unspun\\\\\\n------------------\\\\\\n\\\\\\nCut through the spin with North America correspondent Anthony Zurcher - in your inbox every Wednesday.](https://cloud.email.bbc.com/US_Election_Unspun_newsletter_signup?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=uselectionunspun&at_campaign_type=owned)\\n\\n[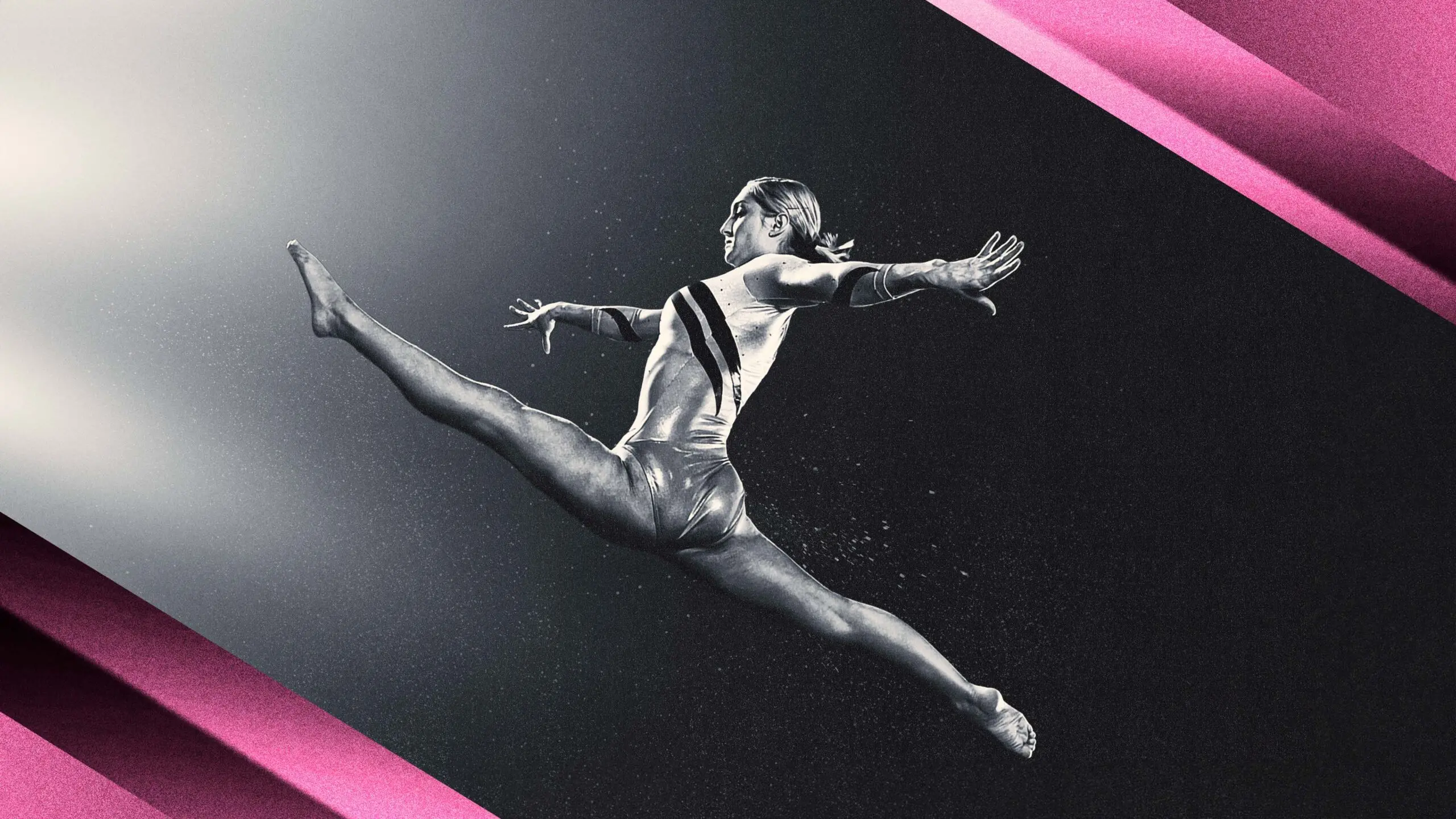\\\\\\n\\\\\\nMedal Moments\\\\\\n-------------\\\\\\n\\\\\\nYour global guide to the Paris Olympics, from key highlights to heroic stories, daily to your inbox throughout the Games.](https://cloud.email.bbc.com/medalmoments_newsletter_signup?&at_bbc_team=studios&at_medium=emails&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.com&at_format=Module&at_link_origin=homepage&at_campaign=olympics2024&at_campaign_id=&at_adset_name=&at_adset_id=&at_creation=&at_creative_id=&at_campaign_type=owned)\\n\\n* * *\\n\\nEarth\\n-----\\n\\n[The \'Paddington bears\' facing climate threat\\\\\\n--------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nNatural wonders](/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-)\\n\\n[The simple Japanese method for a tidier fridge\\\\\\n----------------------------------------------\\\\\\n\\\\\\n3 days ago\\\\\\n\\\\\\nFuture](/future/article/20240715-the-simple-japanese-method-for-a-tidier-and-less-wasteful-fridge)\\n\\n[Conspiracy theories swirl about geo-engineering, but could it help save the planet?\\\\\\n-----------------------------------------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nBBC InDepth](/news/articles/c98qp79gj4no)\\n\\n[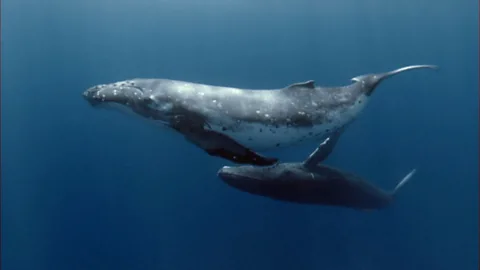\\\\\\n\\\\\\nFace to face with humpback whales\\\\\\n---------------------------------\\\\\\n\\\\\\nReece Parkinson discovers how locals are protecting their stunning marine environment.\\\\\\n\\\\\\n3 days ago\\\\\\n\\\\\\nTravel](/reel/video/p0jbpt60/face-to-face-with-humpback-whales)\\n\\n* * *\\n\\nScience and health\\n------------------\\n\\n[India alert after boy dies from Nipah virus in Kerala\\\\\\n-----------------------------------------------------\\\\\\n\\\\\\n6 hrs ago\\\\\\n\\\\\\nAsia](/news/articles/cj50d7e9vp6o)\\n\\n[Are fermented foods actually good for our health?\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nFuture](/future/article/20240719-are-fermented-foods-actually-good-for-you)\\n\\n[Summer surge: why Covid-19 isn\'t yet seasonal\\\\\\n---------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nFuture](/future/article/20240719-why-covid-19-is-spreading-this-summer)\\n\\n[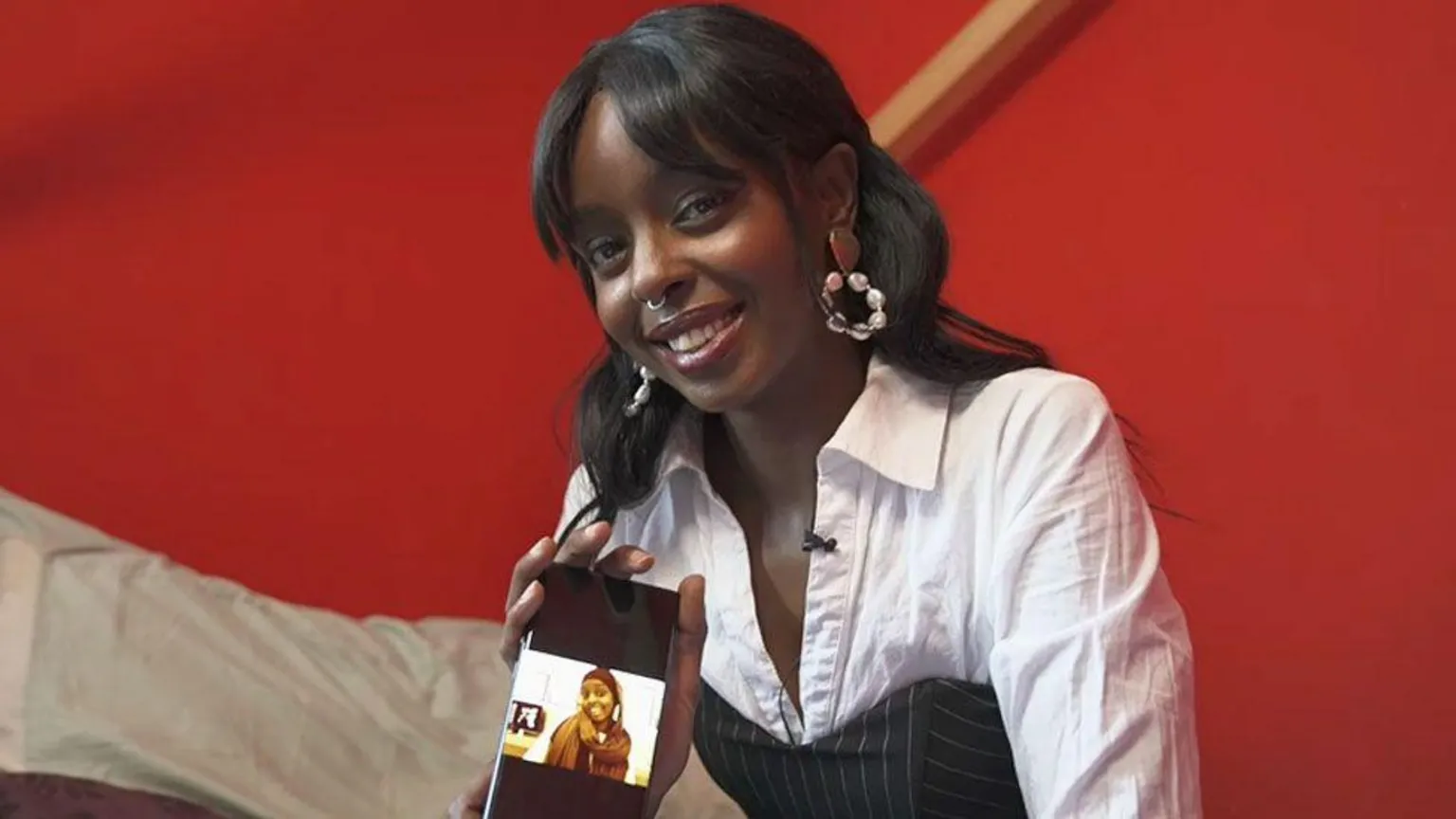\\\\\\n\\\\\\n\'A way to fight back\': FGM survivors reclaim vulvas with surgery\\\\\\n----------------------------------------------------------------\\\\\\n\\\\\\nIn Somalia, it\'s believed cutting off a girl\'s outer genitalia will guarantee their virginity.\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nAfrica](/news/articles/cyx0perl8yno)\\n\\n* * *\\n\\nWorld\\u2019s Table\\n-------------\\n\\n[The Turkish ice cream eaten with a knife and fork\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n1 day ago\\\\\\n\\\\\\nTravel](/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork)\\n\\n[India\'s cooling drinks to beat the heat\\\\\\n---------------------------------------\\\\\\n\\\\\\n7 days ago\\\\\\n\\\\\\nTravel](/travel/article/20240715-gond-katira-a-natural-way-to-cool-down-in-indias-scorching-summers)\\n\\n[The world\'s biggest restaurant is coming to Paris\\\\\\n-------------------------------------------------\\\\\\n\\\\\\n9 Jul 2024\\\\\\n\\\\\\nTravel](/travel/article/20240708-paris-olympics-2024-worlds-biggest-restaurant)\\n\\n[\\\\\\n\\\\\\nThe wild ceremonies of the Turkish \'meatball\'\\\\\\n---------------------------------------------\\\\\\n\\\\\\nOne of the country\'s most popular fast-food items, \\u00e7i\\u011f k\\u00f6fte is traditionally associated with wild and rowdy gatherings in south-eastern Turkey.\\\\\\n\\\\\\n1 Jun 2024\\\\\\n\\\\\\nTravel](/travel/article/20240531-the-wild-ceremonies-surrounding-a-turkish-meatball)\\n\\n* * *\\n\\nThe Specialist\\n--------------\\n\\n[Guide to Helsinki\'s happiest places\\\\\\n-----------------------------------\\\\\\n\\\\\\n2 days ago\\\\\\n\\\\\\nTravel](/travel/article/20240718-a-happiness-hackers-guide-to-the-happiest-outdoor-places-in-helsinki)\\n\\n[A pastry chef\'s favourite bakeries in Paris\\\\\\n-------------------------------------------\\\\\\n\\\\\\n5 days ago\\\\\\n\\\\\\nTravel](/travel/article/20240717-david-lebovitzs-ultimate-guide-to-the-best-bakeries-in-paris-right-now)\\n\\n[Chef Andrew Zimmern\'s favourite US restaurants\\\\\\n----------------------------------------------\\\\\\n\\\\\\n14 Jul 2024\\\\\\n\\\\\\nTravel](/travel/article/20240714-chef-andrew-zimmerns-favourite-us-restaurants)\\n\\n[\\\\\\n\\\\\\nA First Lady\'s guide to Iceland\\\\\\n-------------------------------\\\\\\n\\\\\\nEliza Reid moved to Iceland 20 years ago for love and now she\'s the First Lady. Here are her favourite ways to enjoy a \\"chill\\" Icelandic weekend.\\\\\\n\\\\\\n11 Jul 2024\\\\\\n\\\\\\nTravel](/travel/article/20240710-icelands-first-lady-takes-you-on-a-tour-of-her-super-chill-nation)\\n\\n* * *\\n\\n[British Broadcasting Corporation](/)\\n\\n* [Home](https://www.bbc.com/)\\n \\n* [News](/news)\\n \\n* [Sport](/sport)\\n \\n* [Business](/business)\\n \\n* [Innovation](/innovation)\\n \\n* [Culture](/culture)\\n \\n* [Travel](/travel)\\n \\n* [Earth](/future-planet)\\n \\n* [Video](/video)\\n \\n* [Live](/live)\\n \\n* [Audio](https://www.bbc.co.uk/sounds)\\n \\n* [Weather](https://www.bbc.com/weather)\\n \\n* [BBC Shop](https://shop.bbc.com/)\\n \\n\\nBBC in other languages\\n\\nFollow BBC on:\\n--------------\\n\\n* [Terms of Use](https://www.bbc.co.uk/usingthebbc/terms)\\n \\n* [About the BBC](https://www.bbc.co.uk/aboutthebbc)\\n \\n* [Privacy Policy](https://www.bbc.com/usingthebbc/privacy/)\\n \\n* [Cookies](https://www.bbc.com/usingthebbc/cookies/)\\n \\n* [Accessibility Help](https://www.bbc.co.uk/accessibility/)\\n \\n* [Contact the BBC](https://www.bbc.co.uk/contact)\\n \\n* [Advertise with us](https://www.bbc.com/advertisingcontact)\\n \\n* [Do not share or sell my info](https://www.bbc.com/usingthebbc/cookies/how-can-i-change-my-bbc-cookie-settings/)\\n \\n* [Contact technical support](https://www.bbc.com/contact-bbc-com-help)\\n \\n\\nCopyright 2024 BBC. All rights reserved.\\u00a0\\u00a0The _BBC_ is _not responsible for the content of external sites._\\u00a0[**Read about our approach to external linking.**](https://www.bbc.co.uk/editorialguidelines/guidance/feeds-and-links)", "html": "<!DOCTYPE html><html lang=\\"en-GB\\"><body><div id=\\"__next\\"><div class=\\"app\\"><div class=\\"sc-db60fb3f-0 hyYukw\\"><a href=\\"#main-content\\" aria-label=\\"Skip to content\\" role=\\"link\\" class=\\"sc-db60fb3f-1 bfyvlX\\">Skip to content</a></div><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 wUsAB\\"><div data-testid=\\"dotcom-interstitial\\" id=\\"dotcom-interstitial\\" class=\\"dotcom-ad\\"></div></div><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 wUsAB\\"><div data-testid=\\"dotcom-top\\" id=\\"dotcom-top\\" class=\\"dotcom-ad\\"></div></div><header class=\\"sc-49542412-0 bbWPuq\\"><div class=\\"sc-49542412-1 cwjPWg\\"><div class=\\"sc-49542412-9 etdLat hide-when-no-script\\"><button role=\\"button\\" aria-expanded=\\"false\\" aria-label=\\"Open menu\\" class=\\"sc-49542412-3 ipGSFC\\"><svg viewBox=\\"0 0 32 32\\" width=\\"20\\" height=\\"20\\" category=\\"actions\\" icon=\\"list-view-text\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M1 7.5h30V1.9H1v5.6zm0 22.6h30v-5.6H1v5.6zm0-11.3h30v-5.6H1v5.6z\\"></path></svg></button><button role=\\"button\\" aria-label=\\"Search BBC\\" class=\\"sc-49542412-3 sc-49542412-4 ipGSFC eojOvQ\\"><svg viewBox=\\"0 0 32 32\\" width=\\"20\\" height=\\"20\\" category=\\"actions\\" icon=\\"search\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"m30.6 28.1-8.3-8.3c1.5-2 2.4-4.4 2.4-7.2C24.7 6 19.6 1 13 1S1.4 6.1 1.4 12.7 6.5 24.3 13 24.3c2.3 0 4.4-.6 6.2-1.8l8.5 8.5 2.9-2.9zM4 12.6c0-5.2 3.9-9.1 9-9.1s9 3.9 9 9.1c0 5.2-3.9 9.1-9 9.1s-9-3.9-9-9.1z\\"></path></svg></button></div><div class=\\"sc-49542412-10 jTsKD\\"><div class=\\"sc-49542412-2 hrGuyi\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/\\" class=\\"sc-2e6baa30-0 gILusN\\"><svg xmlns=\\"http://www.w3.org/2000/svg\\" viewBox=\\"0 0 112 32\\" category=\\"logo\\" icon=\\"bbc\\" class=\\"sc-1097f7fe-0 jbvZzi\\"><title>British Broadcasting Corporation</title><path d=\\"M111.99999,4.44444577e-05 L111.99999,32.0000444 L79.9999905,32.0000444 L79.9999905,4.44444577e-05 L111.99999,4.44444577e-05 Z M72.0000119,-3.55271368e-15 L72.0000119,32 L40.0000119,32 L40.0000119,-3.55271368e-15 L72.0000119,-3.55271368e-15 Z M32,-3.55271368e-15 L32,32 L-1.13686838e-13,32 L-1.13686838e-13,-3.55271368e-15 L32,-3.55271368e-15 Z M97.469329,6.80826869 C96.0294397,6.80826869 94.7294393,7.02226876 93.5693278,7.44982444 C92.4089942,7.87782457 91.4137717,8.49471364 90.5841047,9.30049166 C89.7538823,10.1067141 89.1188821,11.07327 88.6785486,12.199937 C88.2378818,13.3269373 88.0177706,14.5896043 88.0177706,15.9876048 C88.0177706,17.4188274 88.2296596,18.7062722 88.6531042,19.8493837 C89.0763265,20.9929396 89.6861045,21.9591621 90.482438,22.748829 C91.2784383,23.5383848 92.2522163,24.1430516 93.4042167,24.5624962 C94.5558837,24.9819408 95.8516619,25.1917186 97.2914401,25.1917186 C98.3752182,25.1917186 99.4086629,25.072163 100.391219,24.8338296 C101.37333,24.5956073 102.237108,24.2706072 102.982664,23.8592738 L102.982664,23.8592738 L102.982664,20.4292727 C101.40733,21.4001619 99.6881074,21.8851621 97.8251069,21.8851621 C96.6054399,21.8851621 95.567884,21.6549398 94.7126615,21.194273 C93.8572168,20.7338284 93.2049944,20.0633837 92.7564387,19.1831613 C92.3073275,18.3032721 92.0831052,17.2380496 92.0831052,15.9876048 C92.0831052,14.7377155 92.3156608,13.6766041 92.7816609,12.8044927 C93.2474389,11.9327147 93.916328,11.2664922 94.7888838,10.8058254 C95.6609951,10.3453809 96.715551,10.1148252 97.9521069,10.1148252 C98.8496628,10.1148252 99.7052186,10.2342697 100.518108,10.4726031 C101.331219,10.7112699 102.084664,11.0609366 102.779442,11.5212701 L102.779442,11.5212701 L102.779442,8.01738016 C102.017219,7.62260227 101.191441,7.32260218 100.302219,7.11671323 C99.4129963,6.91126872 98.4685515,6.80826869 97.469329,6.80826869 Z M55.7552388,7.00000208 L49.0000146,7.00000208 L49.0000146,25.0000021 L56.1713501,25.0000021 C57.590906,25.0000021 58.8063508,24.7903407 59.8181289,24.3706739 C60.8297959,23.9513405 61.6087961,23.3553403 62.1555741,22.5832289 C62.7020187,21.8114509 62.9754632,20.8882284 62.9754632,19.8140059 C62.9754632,18.7232278 62.6941298,17.7960053 62.1312407,17.0321162 C61.5681294,16.2686715 60.7563514,15.7104491 59.6957955,15.3580046 C60.4625736,14.9891156 61.0420182,14.4894488 61.4335738,13.8601152 C61.8252406,13.2307817 62.0210185,12.4881148 62.0210185,11.6321146 C62.0210185,10.1385586 61.4742405,8.99311379 60.3812402,8.19578022 C59.2877954,7.39889109 57.745795,7.00000208 55.7552388,7.00000208 L55.7552388,7.00000208 Z M15.7552269,7.00000208 L9.00000268,7.00000208 L9.00000268,25.0000021 L16.1713381,25.0000021 C17.5908941,25.0000021 18.8062278,24.7903407 19.8182281,24.3706739 C20.8296729,23.9513405 21.6087842,23.3553403 22.1555621,22.5832289 C22.7021179,21.8114509 22.9755624,20.8882284 22.9755624,19.8140059 C22.9755624,18.7232278 22.6941179,17.7960053 22.1311177,17.0321162 C21.5682286,16.2686715 20.7563395,15.7104491 19.6957836,15.3580046 C20.4625616,14.9891156 21.0418952,14.4894488 21.4335619,13.8601152 C21.8252287,13.2307817 22.0210066,12.4881148 22.0210066,11.6321146 C22.0210066,10.1385586 21.4741175,8.99311379 20.3811172,8.19578022 C19.2877835,7.39889109 17.7457831,7.00000208 15.7552269,7.00000208 L15.7552269,7.00000208 Z M55.8775833,17.2209385 C58.1128062,17.2209385 59.2308065,18.0434943 59.2308065,19.6881614 C59.2308065,20.4602728 58.9369175,21.0518285 58.3496951,21.4629397 C57.7622505,21.8743843 56.9216947,22.0797177 55.8286944,22.0797177 L55.8286944,22.0797177 L52.6469157,22.0797177 L52.6469157,17.2209385 Z M15.8775714,17.2209385 C18.1129054,17.2209385 19.2307946,18.0434943 19.2307946,19.6881614 C19.2307946,20.4602728 18.9370167,21.0518285 18.3496832,21.4629397 C17.7622386,21.8743843 16.9216828,22.0797177 15.8286825,22.0797177 L15.8286825,22.0797177 L12.6469038,22.0797177 L12.6469038,17.2209385 Z M55.412572,9.92028073 C57.3541282,9.92028073 58.3252396,10.6338365 58.3252396,12.0600591 C58.3252396,12.7988371 58.0763506,13.373504 57.5786838,13.7846152 C57.0807948,14.1960598 56.3587946,14.4013932 55.412572,14.4013932 L55.412572,14.4013932 L52.6469046,14.4013932 L52.6469046,9.92028073 Z M15.4125601,9.92028073 C17.3541163,9.92028073 18.3252277,10.6338365 18.3252277,12.0600591 C18.3252277,12.7988371 18.0762276,13.373504 17.5786719,13.7846152 C17.0807829,14.1960598 16.3587826,14.4013932 15.4125601,14.4013932 L15.4125601,14.4013932 L12.6468927,14.4013932 L12.6468927,9.92028073 Z\\"></path></svg></a></div></div></div><div class=\\"sc-49542412-11 fdaSom\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/watch-live-news\\" class=\\"sc-2e6baa30-0 gILusN\\"><div class=\\"sc-6e33c01-3 kApzhn\\"><div class=\\"sc-6e33c01-1 gIdQwG\\"><span data-testid=\\"watch-now-live-icon\\" class=\\"sc-6e33c01-0 llEIeC\\"><svg width=\\"32\\" height=\\"32\\" viewBox=\\"0 0 32 32\\" xmlns=\\"http://www.w3.org/2000/svg\\" data-testid=\\"live-icon-svg-styled\\" class=\\"sc-3387039d-0 hgdstu sc-1097f7fe-0 jmthjj\\"><path id=\\"outer\\" d=\\"M13.3922 31.3913C6.25751 30.2611 0.846472 24.5392 0.0835577 17.3057C-0.410924 12.714 1.3127 7.95289 4.63278 4.61868C12.2761 -3.01046 25.1467 -0.891255 29.922 8.78645C32.6204 14.2257 31.9988 20.5268 28.2972 25.4293C24.8924 29.922 19.0151 32.2672 13.3922 31.3913ZM19.0858 28.6505C20.329 28.3255 22.2363 27.4778 23.2394 26.7856C24.2142 26.1215 25.8955 24.4686 26.616 23.4655C27.4213 22.3352 28.2972 20.4986 28.6787 19.0999C29.1167 17.4611 29.1167 14.0986 28.6787 12.4597C28.0288 10.0721 26.7573 7.96702 24.963 6.22927C23.6774 5.01426 22.9003 4.4774 21.3745 3.75687C19.3683 2.81029 18.3087 2.59837 15.7939 2.59837C13.2791 2.59837 12.2195 2.81029 10.2134 3.75687C8.68753 4.4774 7.91049 5.01426 6.62484 6.22927C4.83058 7.96702 3.57318 10.0721 2.90916 12.488C2.4712 14.0703 2.4712 17.4893 2.90916 19.0717C3.57318 21.4876 4.83058 23.5926 6.62484 25.3304C8.15067 26.7856 9.35155 27.5344 11.386 28.2973C13.5193 29.1026 16.7829 29.258 19.0858 28.6505Z\\"></path><path id=\\"inner\\" d=\\"M13.8318 24.0437C10.5541 23.2949 7.94042 20.6671 7.24815 17.3894C6.42872 13.5042 8.40665 9.57661 12.0658 7.83886C16.2901 5.84681 21.4327 7.72584 23.4389 12.0066C25.6287 16.683 23.1422 22.2918 18.1974 23.8459C16.9965 24.2274 15.0186 24.3122 13.8318 24.0437Z\\"></path></svg></span><span class=\\"sc-6e33c01-2 eKiSGW\\">Watch Live</span></div></div></a></div><div class=\\"sc-49542412-12 ePBico\\"><button type=\\"button\\" aria-label=\\"Register\\" class=\\"sc-ec3ac5c8-2 sc-ec3ac5c8-3 eRCykh fsjdUI\\"><span data-testid=\\"button-text\\" aria-hidden=\\"false\\" class=\\"sc-ec3ac5c8-1 bABdmH\\">Register</span></button><button type=\\"button\\" aria-label=\\"Sign In\\" class=\\"sc-ec3ac5c8-2 sc-ec3ac5c8-5 eRCykh jKWpcL\\"><span data-testid=\\"button-text\\" aria-hidden=\\"false\\" class=\\"sc-ec3ac5c8-1 bABdmH\\">Sign In</span></button></div></div></div></header><nav data-testid=\\"level1-navigation-container\\" class=\\"sc-df0290d6-9 akwuv\\"><section class=\\"sc-f116bf72-0 hkTqj\\"><nav class=\\"sc-f116bf72-1 xECcw\\"><ul class=\\"sc-f116bf72-2 bXpjTY\\"><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 jhHpBQ\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink-active\\" href=\\"/\\" class=\\"sc-f116bf72-4 yKcKi\\">Home</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/news\\" class=\\"sc-f116bf72-4 eqTiTw\\">News</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/sport\\" class=\\"sc-f116bf72-4 eqTiTw\\">Sport</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/business\\" class=\\"sc-f116bf72-4 eqTiTw\\">Business</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/innovation\\" class=\\"sc-f116bf72-4 eqTiTw\\">Innovation</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/culture\\" class=\\"sc-f116bf72-4 eqTiTw\\">Culture</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/travel\\" class=\\"sc-f116bf72-4 eqTiTw\\">Travel</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/future-planet\\" class=\\"sc-f116bf72-4 eqTiTw\\">Earth</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 kkeorx\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/video\\" class=\\"sc-f116bf72-4 eqTiTw\\">Video</a></div></li><li data-testid=\\"mainNavigationItemStyled\\" class=\\"sc-f116bf72-3 cHJCBW\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"mainNavigationLink\\" href=\\"/live\\" class=\\"sc-f116bf72-4 eqTiTw\\">Live</a></div></li></ul></nav></section></nav><button class=\\"sc-d1bbe396-0 gMtoMM\\"></button><div data-testid=\\"drawer-background\\" direction=\\"left\\" class=\\"sc-d1bbe396-1 bgdPNO\\"><div class=\\"sc-b11b9313-0 WHFVw\\"><div class=\\"sc-b11b9313-6 gsHPIY\\"><div data-testid=\\"search-input-wrapper\\" class=\\"sc-e1a87ea7-0 fsAmTf\\"><input type=\\"text\\" placeholder=\\"Search news, topics and more\\" data-testid=\\"search-input-field\\" class=\\"sc-e1a87ea7-1 iARAvt\\" value=\\"\\"><button type=\\"button\\" data-testid=\\"search-input-search-button\\" class=\\"sc-73629686-2 sc-73629686-3 blWAHP jQFVUQ\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 hFVpuf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"actions\\" icon=\\"search\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"m30.6 28.1-8.3-8.3c1.5-2 2.4-4.4 2.4-7.2C24.7 6 19.6 1 13 1S1.4 6.1 1.4 12.7 6.5 24.3 13 24.3c2.3 0 4.4-.6 6.2-1.8l8.5 8.5 2.9-2.9zM4 12.6c0-5.2 3.9-9.1 9-9.1s9 3.9 9 9.1c0 5.2-3.9 9.1-9 9.1s-9-3.9-9-9.1z\\"></path></svg></span></button></div></div><div class=\\"sc-5470c371-7 jspHbo\\"><div class=\\"sc-75742244-12 fhcbTQ\\"><button type=\\"button\\" aria-label=\\"Register\\" class=\\"sc-ec3ac5c8-2 sc-ec3ac5c8-3 dVyokB fsjdUI\\"><span data-testid=\\"button-text\\" aria-hidden=\\"false\\" class=\\"sc-ec3ac5c8-1 bABdmH\\">Register</span></button><button type=\\"button\\" aria-label=\\"Sign In\\" class=\\"sc-ec3ac5c8-2 sc-ec3ac5c8-5 dVyokB jKWpcL\\"><span data-testid=\\"button-text\\" aria-hidden=\\"false\\" class=\\"sc-ec3ac5c8-1 bABdmH\\">Sign In</span></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" tabindex=\\"-1\\" href=\\"/\\" class=\\"sc-2e6baa30-0 gILusN\\"><button data-testid=\\"level1NavButton-/home\\" selected=\\"\\" class=\\"sc-b11b9313-2 gWSVBt\\"><span data-testid=\\"level1NavText-/home\\" class=\\"sc-b11b9313-3 hGrftD\\">Home</span></button></a></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/news\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/news\\" class=\\"sc-b11b9313-3 hGrftD\\">News</span><div data-testid=\\"menuItem-ChevronIconWrapper-/news\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/news\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" tabindex=\\"-1\\" href=\\"/sport\\" class=\\"sc-2e6baa30-0 gILusN\\"><button data-testid=\\"level1NavButton-/sport\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/sport\\" class=\\"sc-b11b9313-3 hGrftD\\">Sport</span></button></a></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/business\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/business\\" class=\\"sc-b11b9313-3 hGrftD\\">Business</span><div data-testid=\\"menuItem-ChevronIconWrapper-/business\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/business\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/innovation\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/innovation\\" class=\\"sc-b11b9313-3 hGrftD\\">Innovation</span><div data-testid=\\"menuItem-ChevronIconWrapper-/innovation\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/innovation\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/culture\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/culture\\" class=\\"sc-b11b9313-3 hGrftD\\">Culture</span><div data-testid=\\"menuItem-ChevronIconWrapper-/culture\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/culture\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/travel\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/travel\\" class=\\"sc-b11b9313-3 hGrftD\\">Travel</span><div data-testid=\\"menuItem-ChevronIconWrapper-/travel\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/travel\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/future-planet\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/future-planet\\" class=\\"sc-b11b9313-3 hGrftD\\">Earth</span><div data-testid=\\"menuItem-ChevronIconWrapper-/future-planet\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/future-planet\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" tabindex=\\"-1\\" href=\\"/video\\" class=\\"sc-2e6baa30-0 gILusN\\"><button data-testid=\\"level1NavButton-/video\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/video\\" class=\\"sc-b11b9313-3 hGrftD\\">Video</span></button></a></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><div><button data-testid=\\"level1NavButton-/live\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-/live\\" class=\\"sc-b11b9313-3 hGrftD\\">Live</span><div data-testid=\\"menuItem-ChevronIconWrapper-/live\\" class=\\"sc-b11b9313-1 MYgSf\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-down\\" data-testid=\\"menuItem-ChevronIcon-/live\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M17.7 22.6.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4zm0 0L.9 4.2v6.7L16 27.8 31.1 11V4.3L14.3 22.6h3.4z\\"></path></svg></div></button></div></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><a target=\\"_self\\" href=\\"https://www.bbc.co.uk/sounds\\" class=\\"sc-2e6baa30-0 gILusN\\"><button data-testid=\\"level1NavButton-https://www.bbc.co.uk/sounds\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-https://www.bbc.co.uk/sounds\\">Audio</span></button></a></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><a target=\\"_self\\" href=\\"https://www.bbc.com/weather\\" class=\\"sc-2e6baa30-0 gILusN\\"><button data-testid=\\"level1NavButton-https://www.bbc.com/weather\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-https://www.bbc.com/weather\\">Weather</span></button></a></div><div data-testid=\\"navigationPanel-navItem-level1-false\\" class=\\"sc-b11b9313-5 eaJOnH\\"><a target=\\"_self\\" href=\\"https://www.bbc.com/newsletters\\" class=\\"sc-2e6baa30-0 gILusN\\"><button data-testid=\\"level1NavButton-https://www.bbc.com/newsletters\\" class=\\"sc-b11b9313-2 jFnfdm\\"><span data-testid=\\"level1NavText-https://www.bbc.com/newsletters\\">Newsletters</span></button></a></div></div></div><main id=\\"main-content\\"><article class=\\"sc-9636e898-0 dYtsiK\\"><section data-testid=\\"vermont-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Section without title - vermont\\" data-analytics_group_type=\\"vermont-7\\" data-analytics_group_position=\\"1\\" data-analytics_group_item_count=\\"7\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"vermont-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><div data-testid=\\"Vermont-grid\\" class=\\"sc-e70150c3-0 fbvxoY\\"><div class=\\"sc-93223220-0 bOZIBp\\"><div data-testid=\\"first-grid\\" class=\\"sc-93223220-0 sc-e70150c3-1 gPmTJa fNRFwC\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c250zqgrpqgo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp\\" loading=\\"lazy\\" alt=\\"Biden\'s last public appearance in Delaware before announcing he would withdraw from the race\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The last days of the Biden campaign \\u2013 BBC correspondent\\u2019s account<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">BBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\'s campaign.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c3gdzmdje5xo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 huzxDy\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp\\" loading=\\"lazy\\" alt=\\"Joe Biden looks on during a speech at the White House on 14 July \\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Isolating at a beach house, Biden gave aides one minute notice of exit<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Most of the president\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">15 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div></div><div data-testid=\\"first-grid-narrow\\" class=\\"sc-93223220-0 sc-e70150c3-2 jrqxhV jzPkfJ\\"><div data-testid=\\"dundee-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c250zqgrpqgo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"dundee-article\\" class=\\"sc-1a9a6d5b-1 iYglrs\\"><div data-testid=\\"card-image-wrapper\\" class=\\"sc-1a9a6d5b-3 jsqFvT\\"><div data-testid=\\"card-media\\" class=\\"sc-1a9a6d5b-4 fGVWed\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/05a8/live/0dce30e0-47ee-11ef-96a8-e710c6bfc866.jpg.webp\\" loading=\\"lazy\\" alt=\\"Biden\'s last public appearance in Delaware before announcing he would withdraw from the race\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-93223220-0 gcYoBW\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The last days of the Biden campaign \\u2013 BBC correspondent\\u2019s account<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-1a9a6d5b-0 kGKNqV\\">BBC correspondent Tom Bateman takes us behind the scenes in the final days of Biden\'s campaign.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div><div data-testid=\\"dundee-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c3gdzmdje5xo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"dundee-article\\" class=\\"sc-1a9a6d5b-1 iYglrs\\"><div data-testid=\\"card-image-wrapper\\" class=\\"sc-1a9a6d5b-3 jsqFvT\\"><div data-testid=\\"card-media\\" class=\\"sc-1a9a6d5b-4 fGVWed\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/ffdd/live/f2086770-4808-11ef-96a8-e710c6bfc866.jpg.webp\\" loading=\\"lazy\\" alt=\\"Joe Biden looks on during a speech at the White House on 14 July \\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-93223220-0 gcYoBW\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Isolating at a beach house, Biden gave aides one minute notice of exit<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-1a9a6d5b-0 kGKNqV\\">Most of the president\'s senior aides found out about his decision to exit the race minutes before he publicly announced it.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">15 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div></div><div class=\\"sc-93223220-0 jrqxhV\\"><div data-testid=\\"westminster-card\\"><div data-testid=\\"westminster-live\\" class=\\"sc-6781995d-3 fGXoHh\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/news/live/cv2gryx1yx1t\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"westminster\\" class=\\"sc-6781995d-2 jOKQKE\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-6781995d-4 fTeUGL\\"><div data-testid=\\"card-media\\" class=\\"sc-6781995d-1 iHDalG\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/ace/standard/240/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/ace/standard/320/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/ace/standard/480/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/ace/standard/640/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/ace/standard/800/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/ace/standard/1024/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/ace/standard/1536/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/ace/standard/480/cpsprodpb/7856/live/1ce684a0-4844-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"eager\\" alt=\\"Kamala Harris gestures as she speaks at the White House on Monday\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-6781995d-5 dWflPh\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 hJUdDc\\"><div class=\\"sc-4d9540e7-1 sc-4fedabc7-2 gglKuy jOAobE\\"><svg width=\\"32\\" height=\\"32\\" viewBox=\\"0 0 32 32\\" xmlns=\\"http://www.w3.org/2000/svg\\" data-testid=\\"live-icon-svg-styled\\" class=\\"sc-3387039d-0 hgdstu sc-1097f7fe-0 jmthjj\\"><path id=\\"outer\\" d=\\"M13.3922 31.3913C6.25751 30.2611 0.846472 24.5392 0.0835577 17.3057C-0.410924 12.714 1.3127 7.95289 4.63278 4.61868C12.2761 -3.01046 25.1467 -0.891255 29.922 8.78645C32.6204 14.2257 31.9988 20.5268 28.2972 25.4293C24.8924 29.922 19.0151 32.2672 13.3922 31.3913ZM19.0858 28.6505C20.329 28.3255 22.2363 27.4778 23.2394 26.7856C24.2142 26.1215 25.8955 24.4686 26.616 23.4655C27.4213 22.3352 28.2972 20.4986 28.6787 19.0999C29.1167 17.4611 29.1167 14.0986 28.6787 12.4597C28.0288 10.0721 26.7573 7.96702 24.963 6.22927C23.6774 5.01426 22.9003 4.4774 21.3745 3.75687C19.3683 2.81029 18.3087 2.59837 15.7939 2.59837C13.2791 2.59837 12.2195 2.81029 10.2134 3.75687C8.68753 4.4774 7.91049 5.01426 6.62484 6.22927C4.83058 7.96702 3.57318 10.0721 2.90916 12.488C2.4712 14.0703 2.4712 17.4893 2.90916 19.0717C3.57318 21.4876 4.83058 23.5926 6.62484 25.3304C8.15067 26.7856 9.35155 27.5344 11.386 28.2973C13.5193 29.1026 16.7829 29.258 19.0858 28.6505Z\\"></path><path id=\\"inner\\" d=\\"M13.8318 24.0437C10.5541 23.2949 7.94042 20.6671 7.24815 17.3894C6.42872 13.5042 8.40665 9.57661 12.0658 7.83886C16.2901 5.84681 21.4327 7.72584 23.4389 12.0066C25.6287 16.683 23.1422 22.2918 18.1974 23.8459C16.9965 24.2274 15.0186 24.3122 13.8318 24.0437Z\\"></path></svg><span class=\\"sc-4fedabc7-4 dWoXdj\\">LIVE</span></div><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 eVkEgC\\">Kamala Harris speaks for first time since Biden left race - as endorsements mount<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-6781995d-0 eZxCk\\">The US vice-president appears at the White House for a pre-scheduled event - as key Democrats line up to back her candidacy.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"></div></div></div></a></div><hr class=\\"sc-6781995d-6 bbWpTX\\"></div></div></div></div><div data-testid=\\"second-grid\\" class=\\"sc-93223220-0 sc-e70150c3-3 jPpGMu kdbokE\\"><div data-testid=\\"manchester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cpwd8yzw45qo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"manchester-article\\" class=\\"sc-e5949eb5-1 cMUTXL\\"><div data-testid=\\"card-text-wrapper\\" class=\\"sc-e5949eb5-2 eOoDdE\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">What Biden quitting means for Harris, the Democrats and Trump<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-e5949eb5-0 gpCoKv\\">President Biden has upended the 2024 White House race for the Democrats. Here is what it means for Kamala Harris, his party and Trump.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">19 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div><div data-testid=\\"manchester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c80ekdwk9zro\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"manchester-article\\" class=\\"sc-e5949eb5-1 cMUTXL\\"><div data-testid=\\"card-text-wrapper\\" class=\\"sc-e5949eb5-2 eOoDdE\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Who could be Kamala Harris\'s running mate? <!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-e5949eb5-0 gpCoKv\\">It\\u2019s not a done deal but some potential rivals have quickly thrown their support behind her.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">10 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div><div data-testid=\\"manchester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/news/live/c724wqpy4qnt\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"manchester-live\\" class=\\"sc-e5949eb5-1 cMUTXL\\"><div data-testid=\\"card-text-wrapper\\" class=\\"sc-e5949eb5-2 eOoDdE\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><div class=\\"sc-4d9540e7-1 sc-4fedabc7-2 cfxUiL cXvgVW\\"><svg width=\\"32\\" height=\\"32\\" viewBox=\\"0 0 32 32\\" xmlns=\\"http://www.w3.org/2000/svg\\" data-testid=\\"live-icon-svg-styled\\" class=\\"sc-3387039d-0 hgdstu sc-1097f7fe-0 jmthjj\\"><path id=\\"outer\\" d=\\"M13.3922 31.3913C6.25751 30.2611 0.846472 24.5392 0.0835577 17.3057C-0.410924 12.714 1.3127 7.95289 4.63278 4.61868C12.2761 -3.01046 25.1467 -0.891255 29.922 8.78645C32.6204 14.2257 31.9988 20.5268 28.2972 25.4293C24.8924 29.922 19.0151 32.2672 13.3922 31.3913ZM19.0858 28.6505C20.329 28.3255 22.2363 27.4778 23.2394 26.7856C24.2142 26.1215 25.8955 24.4686 26.616 23.4655C27.4213 22.3352 28.2972 20.4986 28.6787 19.0999C29.1167 17.4611 29.1167 14.0986 28.6787 12.4597C28.0288 10.0721 26.7573 7.96702 24.963 6.22927C23.6774 5.01426 22.9003 4.4774 21.3745 3.75687C19.3683 2.81029 18.3087 2.59837 15.7939 2.59837C13.2791 2.59837 12.2195 2.81029 10.2134 3.75687C8.68753 4.4774 7.91049 5.01426 6.62484 6.22927C4.83058 7.96702 3.57318 10.0721 2.90916 12.488C2.4712 14.0703 2.4712 17.4893 2.90916 19.0717C3.57318 21.4876 4.83058 23.5926 6.62484 25.3304C8.15067 26.7856 9.35155 27.5344 11.386 28.2973C13.5193 29.1026 16.7829 29.258 19.0858 28.6505Z\\"></path><path id=\\"inner\\" d=\\"M13.8318 24.0437C10.5541 23.2949 7.94042 20.6671 7.24815 17.3894C6.42872 13.5042 8.40665 9.57661 12.0658 7.83886C16.2901 5.84681 21.4327 7.72584 23.4389 12.0066C25.6287 16.683 23.1422 22.2918 18.1974 23.8459C16.9965 24.2274 15.0186 24.3122 13.8318 24.0437Z\\"></path></svg><span class=\\"sc-4fedabc7-4 hjkPtM\\">LIVE</span></div><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Tensions flare as Congress presses Secret Service boss on \'failed\' Trump rally security<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-e5949eb5-0 gpCoKv\\">Members of both parties have called for Kimberly Cheatle to resign in a House committee hearing that is seeking answers over security failures at the rally on 13 July.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"></div></div></div></a></div></div><div data-testid=\\"manchester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c16j896003xo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"manchester-article\\" class=\\"sc-e5949eb5-1 cMUTXL\\"><div data-testid=\\"card-text-wrapper\\" class=\\"sc-e5949eb5-2 eOoDdE\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The president\'s protectors are hardly noticeable - until things go wrong<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-e5949eb5-0 gpCoKv\\">The attempted assassination of Donald Trump has raised questions about the Secret Service\'s record.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">6 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div></div></div></div></section><section data-testid=\\"indiana-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Only from the BBC\\" data-analytics_group_type=\\"indiana-2\\" data-analytics_group_position=\\"2\\" data-analytics_group_item_count=\\"2\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"indiana-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"indiana-line\\" class=\\"sc-54d40974-0 kJcwKJ\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 ibsSfW\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 data-testid=\\"indiana-title\\" class=\\"sc-54d40974-2 eKfxoB\\">Only from the BBC</h2></div></div></div><div data-testid=\\"indiana-grid-2\\" class=\\"sc-9031d59c-0 jKLwmR\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future/article/20240722-why-you-are-probably-sitting-down-for-too-long\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jcwm61.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jcwm61.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jcwm61.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jcwm61.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jcwm61.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jcwm61.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jcwm61.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jcwm61.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jcwm61.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jcwm61.jpg.webp\\" loading=\\"lazy\\" alt=\\"Two people sit in deck chairs surrounded by water (Credit: Getty Images)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Why you are probably sitting for too long<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Sitting down is ingrained in most peoples\' days. But staying sedentary for too long can increase the risk of serious health conditions like cardiovascular disease and type 2 diabetes.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">4 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Future</span></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c51yqdy3q61o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/229c/live/a8aaa380-4520-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"South African convicted killer Louis van Schoor stares into the middle distance\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Mass killer who \\u2018hunted\\u2019 black people says police encouraged him<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Ex-security guard, Louis van Schoor, killed dozens in South Africa but was only jailed for seven murders.\\n</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">17 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Africa</span></div></div></div></a></div></div></div></div></section><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 fHxdon\\"><div data-testid=\\"dotcom-mid_1\\" id=\\"dotcom-mid_1\\" class=\\"dotcom-ad\\"></div></div><section data-testid=\\"virginia-section-outer-8\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"More news\\" data-analytics_group_type=\\"virginia-8\\" data-analytics_group_position=\\"4\\" data-analytics_group_item_count=\\"8\\" data-analytics_group_link=\\"https://www.bbc.com/news\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"virginia-section-8\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"virginia-line\\" class=\\"sc-54d40974-0 kJcwKJ\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 bfKEqT\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/news\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div class=\\"sc-54d40974-5 eErSgx\\"><h2 data-testid=\\"virginia-title\\" class=\\"sc-54d40974-2 eKfxoB\\">More news</h2> <!-- --><svg viewBox=\\"0 0 32 32\\" width=\\"15\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-right\\" data-testid=\\"section-title-chevron\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M21.6 14.3 5.5 31h6.4l14.6-15L11.9 1H5.5l16.1 16.7v-3.4z\\"></path></svg></div></a></div></div><div data-testid=\\"undefined-grid-8\\" class=\\"sc-b38350e4-1 dlepEy\\"><div class=\\"sc-93223220-0 dyVGhC\\"><div data-testid=\\"london-card\\"><div data-testid=\\"london-article\\" class=\\"sc-f98732b0-3 ephYtw\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/crgk8gnpg0zo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"london\\" class=\\"sc-f98732b0-2 bNZLNs\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-f98732b0-4 emSCvS\\"><div data-testid=\\"card-media\\" class=\\"sc-f98732b0-1 jSgXDY\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 50vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/303f/live/e836a6a0-481a-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"File photo showing downtown Dubai\'s skyline (12 June 2021)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-f98732b0-5 gGpWNz\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 dsoipF\\">UAE jails 57 Bangladeshis over protests against own government<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-f98732b0-0 iQbkqW\\">Three defendants were sentenced to life after being convicted of \\"inciting riots\\" in the Gulf state.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">5 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">World</span></div></div></div></a></div><div class=\\"sc-f98732b0-6 fbvHdc\\"></div></div></div></div><div class=\\"sc-93223220-0 sc-b38350e4-4 jqlqhc hprnWz\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cgerz8we1vgo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"A Palestinian woman sitting on a wheelchair is pulled by a man as they flee eastern Khan Younis in response to an Israeli evacuation order, in the southern Gaza Strip (22 July 2024)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Israel orders evacuation of part of Gaza humanitarian zone<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Strikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Middle East</span></div></div></div></a></div></div></div><div class=\\"sc-93223220-0 sc-b38350e4-5 jqlqhc cdefVm\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cw4yxd3dw7qo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"Black and white photo of Prince George in shirt and suit\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">New Prince George photo released on 11th birthday<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Kensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">7 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">UK</span></div></div></div></a></div></div></div><div class=\\"sc-93223220-0 biogCF\\"><div data-testid=\\"manchester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/formula1/articles/cg3jzy3e8q1o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"manchester-article\\" class=\\"sc-e5949eb5-1 cMUTXL\\"><div data-testid=\\"card-text-wrapper\\" class=\\"sc-e5949eb5-2 eOoDdE\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Piastri wins in Hungary after Norris team orders row<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-e5949eb5-0 gpCoKv\\">Oscar Piastri takes his maiden grand prix victory ahead of McLaren team-mate Lando Norris in a dramatic race in Hungary.</p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Formula 1</span></div></div></div></a></div></div><div data-testid=\\"manchester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cj50d7e9vp6o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"manchester-article\\" class=\\"sc-e5949eb5-1 cMUTXL\\"><div data-testid=\\"card-text-wrapper\\" class=\\"sc-e5949eb5-2 eOoDdE\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">India alert after boy dies from Nipah virus in Kerala<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-e5949eb5-0 gpCoKv\\">The virus is transmitted from animals such as pigs and fruit bats to humans. </p><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">6 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Asia</span></div></div></div></a></div></div></div><div class=\\"sc-93223220-0 sc-b38350e4-2 cmkdDu QUMNJ\\"><div class=\\"sc-b38350e4-3 gugNoq\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cgerz8we1vgo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/94c8/live/2424db40-4830-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"A Palestinian woman sitting on a wheelchair is pulled by a man as they flee eastern Khan Younis in response to an Israeli evacuation order, in the southern Gaza Strip (22 July 2024)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Israel orders evacuation of part of Gaza humanitarian zone<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Strikes are reported near Khan Younis, after people are told to leave eastern areas in the zone.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Middle East</span></div></div></div></a></div></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/czrj18yp489o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/b9f0/live/7390b470-47e9-11ef-80f2-8f08f27244b7.jpg.webp\\" loading=\\"lazy\\" alt=\\"A Kanwariya carries holy water collected from Ganga River in Haridwar during Kanwar Yatra at Sector 14A, on July 10, 2023 in Noida\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">India court blocks order for eateries to display owners\' names<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Critics say ordering restaurants to prominently display names of owners is discriminatory towards Muslims.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">6 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Asia</span></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cgl7e33n1d0o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/bb5b/live/363718b0-47c3-11ef-be99-e9774e2b4d6b.jpg.webp\\" loading=\\"lazy\\" alt=\\"Information screen informs train travellers of global IT outage. \\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">\'Significant number\' of devices fixed - CrowdStrike<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Cybersecurity firm behind global outage says it continues to focus on restoring all impacted computers.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">13 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Business</span></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cq5jwyel12qo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/7f64/live/70d23da0-45ad-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"An employee uses a jigsaw to cut a plastic pipe at the Grundfos AS factory in Chennai, India, on Monday, Nov. 27, 2017.\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Modi\'s new budget faces jobs crisis test in India<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Mr Modi\'s biggest challenge in his third term will be bridging the rich-poor divide.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">16 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Asia</span></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cw4yxd3dw7qo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 768px) 33vw, 96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/d483/live/f8c3d070-480a-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"Black and white photo of Prince George in shirt and suit\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">New Prince George photo released on 11th birthday<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Kensington Palace has posted the image, taken by his mother, Catherine, Princess of Wales.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">7 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">UK</span></div></div></div></a></div></div></div></div></div></section><section data-testid=\\"texas-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Must watch\\" data-analytics_group_type=\\"texas-8\\" data-analytics_group_position=\\"5\\" data-analytics_group_item_count=\\"8\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 bPAIKF\\"><div data-testid=\\"texas-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"texas-line\\" class=\\"sc-54d40974-0 hmqmHz\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 ibsSfW\\"><div><div class=\\"sc-54d40974-5 bJiMTl\\"><h2 data-testid=\\"texas-title\\" class=\\"sc-54d40974-2 bpvsYf\\">Must watch</h2></div></div><div class=\\"sc-1592b9e3-0 iIHNpr\\"><button data-testid=\\"left-arrow\\" disabled=\\"\\" aria-label=\\"Previous Page\\" class=\\"sc-1592b9e3-1 kCHTgr\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-left\\" class=\\"sc-2c06e71a-0 fsMljb\\"><path d=\\"M10.4 14.3 26.5 31h-6.4L5.5 16 20.1 1h6.4L10.4 17.7v-3.4z\\"></path></svg></button><button data-testid=\\"right-arrow\\" aria-label=\\"Next Page\\" class=\\"sc-1592b9e3-1 cNkCqE\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-right\\" class=\\"sc-2c06e71a-0 fsMljb\\"><path d=\\"M21.6 14.3 5.5 31h6.4l14.6-15L11.9 1H5.5l16.1 16.7v-3.4z\\"></path></svg></button></div></div><div data-testid=\\"texas-grid\\" class=\\"sc-8a8cf983-0 iYKspt\\"><div class=\\"sc-8a8cf983-1 gaQDrT\\"><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/cx028eq4qg1o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/cc3c/live/cd687d10-4808-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"Biden speaks at a rally\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Biden\\u2019s disastrous few weeks... in 90 seconds<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">President Biden has faced intense pressure to step aside since his faltering debate performance.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">17 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">US & Canada</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/cmj24x03r8po\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/80df/live/5d6dff00-482e-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"Royal Netherlands Navy\'s anti-submarine helicopter\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Inside the Netherlands Navy\'s anti-submarine helicopter<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">The NH90 was on display at the 2024 Royal International Air Tattoo show at RAF Fairford.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">4 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">England</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/c51yrr2z74no\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/757d/live/00b226b0-47b6-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"Woman with glasses and nose ring\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Democrats in Michigan react to Biden dropping out<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">Democratic voters in Michigan give their take on Joe Biden withdrawing from the US presidential race.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">18 hrs ago</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/reel/video/p0jch19q/turkey-s-answer-to-burning-man-\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jchx33.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jchx33.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jchx33.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jchx33.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jchx33.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jchx33.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jchx33.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jchx33.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jchx33.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jchx33.jpg.webp\\" loading=\\"lazy\\" alt=\\"Turkey\'s answer to \'Burning Man\'\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Turkey\'s answer to \'Burning Man\'<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">A music and art extravaganza takes place in an \'otherworldly\' landscape amid unique volcanic rock formations.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">13 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">Culture & Experiences</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/basketball/videos/cg640yk3n3zo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/aac4/live/37ef8560-4837-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"Jayson Tatum\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Tatum on handling criticism and \'joy\' of Olympics<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">Team USA and Boston Celtics power forward Jayson Tatum says \\"basketball can\'t be your sole purpose\\" as he speaks about facing criticism, and the \\"joy\\" that playing in the Olympics brings.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">2 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">Olympic Games</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/cqe6q917y1jo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/598c/live/ee81bb50-4750-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"The world\'s longest rowing boat\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">World\'s longest rowing boat to carry Olympic torch<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">The 24-seater boat will take the Olympic torch down a section of the River Marne on Sunday.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">Europe</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/c0jq593xqk8o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/1d34/live/14fec1f0-4513-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"Kellie Dingwall\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Accessibility brings disabled gamers a sense of community<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">Greater accessibility in game development is opening the genre to more people with disabilities. </p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">Scotland</span></div></div></div></a></div></div></div><div class=\\"sc-8a8cf983-2 jupLmo\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/cjk325014g7o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-dd979baf-0 jEhueU\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1280px) 20vw, (min-width: 1008px) 25vw, (min-width: 800px) 33vw, 50vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/7bc6/live/13011710-46dd-11ef-9e1c-3b4a473456a6.jpg.webp\\" loading=\\"lazy\\" alt=\\"A plume of smoke rises above Dallas, Texas\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 eTiihu\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 iscyVn\\">Watch: Spire collapses as fire engulfs Texas church<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 fsrXqA\\">A blaze at a historic church in Dallas has caused huge plumes of smoke to rise over the Texan city</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 iIHPGq\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 gsPugk\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 dLCxCA\\">US & Canada</span></div></div></div></a></div></div></div></div></div></div></section><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 fHxdon\\"><div data-testid=\\"dotcom-mid_2\\" id=\\"dotcom-mid_2\\" class=\\"dotcom-ad\\"></div></div><section data-testid=\\"oregon-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"In History\\" data-analytics_group_type=\\"oregon-1\\" data-analytics_group_position=\\"7\\" data-analytics_group_item_count=\\"1\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"oregon-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"oregon-line\\" class=\\"sc-54d40974-0 kJcwKJ\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 ibsSfW\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 data-testid=\\"oregon-title\\" class=\\"sc-54d40974-2 eKfxoB\\">In History</h2></div></div></div><div data-testid=\\"oregon-grid\\" class=\\"sc-93223220-0 bVbxSm\\"><div data-testid=\\"windsor-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/culture/article/20240718-a-ww1-soldier-on-the-brutality-of-conflict\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"windsor-article\\" class=\\"sc-ceaf77c0-2 dxUpSs\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-ceaf77c0-4 gSbhqz\\"><div data-testid=\\"card-media\\" class=\\"sc-ceaf77c0-1 kLSjAK\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1280px) 50vw, (min-width: 1008px) 66vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jcw58f.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jcw58f.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jcw58f.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jcw58f.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jcw58f.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jcw58f.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jcw58f.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jcw58f.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jcw58f.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jcw58f.jpg.webp\\" loading=\\"lazy\\" alt=\\"Stefan Westmann (Credit: BBC Archive)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-ceaf77c0-5 jDazYH\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 dsoipF\\">\'He was after my life\': WW1 soldier\'s confession<!-- --></h2></div></div><div data-testid=\\"card-description\\" class=\\"sc-ceaf77c0-0 eICGnP\\">World War One broke out on 28 July, 1914. Fifty years later, one of the German soldiers, Stefan Westmann, told the BBC about his experiences fighting in the conflict.</div><div data-testid=\\"card-button\\" class=\\"sc-ceaf77c0-6 kCHiOr\\"><button type=\\"button\\" aria-label=\\"Learn more\\" class=\\"sc-73629686-2 sc-73629686-4 blWAHP iolhqz\\"><span data-testid=\\"button-text\\" aria-hidden=\\"true\\" class=\\"sc-73629686-1 eUXcZD\\">See more</span></button></div></div></div></a></div></div></div></div></section><section data-testid=\\"montana-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Olympic Games\\" data-analytics_group_type=\\"montana-1\\" data-analytics_group_position=\\"8\\" data-analytics_group_item_count=\\"1\\" data-analytics_group_link=\\"https://www.bbc.com/sport/olympics\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"montana-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"montana-line\\" class=\\"sc-54d40974-0 kJcwKJ\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 bfKEqT\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/sport/olympics\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div class=\\"sc-54d40974-5 eErSgx\\"><h2 data-testid=\\"montana-title\\" class=\\"sc-54d40974-2 eKfxoB\\">Olympic Games</h2> <!-- --><svg viewBox=\\"0 0 32 32\\" width=\\"15\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-right\\" data-testid=\\"section-title-chevron\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M21.6 14.3 5.5 31h6.4l14.6-15L11.9 1H5.5l16.1 16.7v-3.4z\\"></path></svg></div></a></div></div><div data-testid=\\"montana-grid\\" class=\\"sc-93223220-0 bVbxSm\\"><div data-testid=\\"windsor-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/football/articles/cek91m98g48o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"windsor-article\\" class=\\"sc-ceaf77c0-2 gYCJCs\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-ceaf77c0-4 iUPAAG\\"><div data-testid=\\"card-media\\" class=\\"sc-ceaf77c0-1 kLSjAK\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1008px) 75vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/76b3/live/1c0146e0-4756-11ef-967d-737978ba9b63.png.webp\\" loading=\\"lazy\\" alt=\\"Julian Alvarez and Marta\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-ceaf77c0-5 fekbBw\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 dsoipF\\">Ten footballers to watch out for at Paris Olympics<!-- --></h2></div></div><div data-testid=\\"card-description\\" class=\\"sc-ceaf77c0-0 eICGnP\\">From Manchester City\'s Julian Alvarez to Brazil icon Marta, BBC Sport picks out 10 footballers to watch at the Olympics.</div><div data-testid=\\"card-button\\" class=\\"sc-ceaf77c0-6 kCHiOr\\"><button type=\\"button\\" aria-label=\\"Learn more\\" class=\\"sc-73629686-2 sc-73629686-4 blWAHP iolhqz\\"><span data-testid=\\"button-text\\" aria-hidden=\\"true\\" class=\\"sc-73629686-1 eUXcZD\\">See more</span></button></div></div></div></a></div></div></div></div></section><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 fHxdon\\"><div data-testid=\\"dotcom-mid_3\\" id=\\"dotcom-mid_3\\" class=\\"dotcom-ad\\"></div></div><section data-testid=\\"wyoming-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Section without title - wyoming\\" data-analytics_group_type=\\"wyoming-0\\" data-analytics_group_position=\\"10\\" data-analytics_group_item_count=\\"0\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"wyoming-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><div class=\\"sc-93223220-0 ggGYNa\\"><div data-testid=\\"wyoming-group-grid\\" class=\\"sc-93223220-0 jitrPO\\"><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">US and Canada news</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/crgk8rgm87lo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">\'The right move but is it too late?\' Democratic voters react<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">Just now</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c80ekdwk9zro\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Who could be Kamala Harris\'s running mate? <!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">10 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cq5xdq71drro\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Biden has backed Harris. What happens next in US election?<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">18 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/videos/c51y936y9g2o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/44e6/live/0560ada0-4845-11ef-b74c-bb483a802c97.jpg.webp\\" loading=\\"lazy\\" alt=\\"Kamala Harris speaking at the White House\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 jsCOsl\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Biden\'s legacy of accomplishment is unmatched - Harris<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">The US Vice-President praised Joe Biden\'s track record as US president.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">34 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">US & Canada</span></div></div></div></a></div></div></div></div><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Global news</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cn08d7vyj6wo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">At least six killed in Croatia nursing home shooting<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">7 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Europe</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cgerz8we1vgo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Israel orders evacuation of part of Gaza humanitarian zone<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Middle East</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cn08d7j1qj5o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Russian-US journalist jailed for \'false information\'<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Europe</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cd1e48677w0o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/1f9f/live/ec833280-481c-11ef-96a8-e710c6bfc866.jpg.webp\\" loading=\\"lazy\\" alt=\\"Former information minister of Tanzania, Nape Nnauye\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Tanzanian minister sacked after poll rigging remarks<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Nape Nnauye caused outrage for saying he could help an MP rig elections - comments, he said, he made in jest.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Africa</span></div></div></div></a></div></div></div></div></div><div data-testid=\\"wyoming-group-grid\\" class=\\"sc-93223220-0 jitrPO\\"><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">UK news</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cxw29lg4y8go\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Campaigners in court over Magna Carta incident<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">54 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">UK</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cw4yxd3dw7qo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">New Prince George photo released on 11th birthday<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">7 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">UK</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c4ngrdpwlx3o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">\'We have one of the best comedy scenes in the UK\'<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">12 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">UK</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cxr2g7rk3dxo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/a921/live/f7aeabe0-4465-11ef-a959-6902b6903fc8.jpg.webp\\" loading=\\"lazy\\" alt=\\"Members of Parliament, newly elected in the 2024 general election, gather in the House of Commons Chamber for a group photo\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">How does a surprise MP prepare for life in office?<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">New MPs in the East describe the experience of unexpectedly picking up the reins of public life.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">12 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">England</span></div></div></div></a></div></div></div></div><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Sport</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/football/articles/c9e950ev0xpo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Ferdinand\'s persuasive powers sealed Man Utd\'s Yoro deal<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">49 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Man Utd</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/football/articles/cgrlgzx6gpro\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Aston Villa complete \\u00a350m deal for Everton\'s Onana<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Premier League</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/articles/c2v0j0j6nqlo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Australia would not pick convicted rapist Olympian<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Olympic Games</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport/golf/articles/cv2g1glwypqo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/e404/live/2ccb8690-483f-11ef-96a8-e710c6bfc866.jpg.webp\\" loading=\\"lazy\\" alt=\\"Xander Schaufelle looks at the Claret Jug\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">\'Schauffele passes ultimate examination in classic Open\'<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">The 152nd Open should be remembered as a classic, writes BBC golf correspondent Iain Carter.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 hr ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Golf</span></div></div></div></a></div></div></div></div></div></div></div></section><section data-testid=\\"montana-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Video\\" data-analytics_group_type=\\"montana-1\\" data-analytics_group_position=\\"11\\" data-analytics_group_item_count=\\"1\\" data-analytics_group_link=\\"https://www.bbc.com/video\\" class=\\"sc-35aa3a40-1 gdcnpz\\"><div data-testid=\\"montana-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"montana-line\\" class=\\"sc-54d40974-0 hmqmHz\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 bfKEqT\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/video\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div class=\\"sc-54d40974-5 bJiMTl\\"><h2 data-testid=\\"montana-title\\" class=\\"sc-54d40974-2 bpvsYf\\">Video</h2> <!-- --><svg viewBox=\\"0 0 32 32\\" width=\\"15\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-right\\" data-testid=\\"section-title-chevron\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M21.6 14.3 5.5 31h6.4l14.6-15L11.9 1H5.5l16.1 16.7v-3.4z\\"></path></svg></div></a></div></div><div data-testid=\\"montana-grid\\" class=\\"sc-93223220-0 bVbxSm\\"><div data-testid=\\"windsor-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"windsor-video\\" class=\\"sc-ceaf77c0-2 gYCJCs\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-ceaf77c0-4 iUPAAG\\"><div data-testid=\\"card-media\\" class=\\"sc-ceaf77c0-1 kLSjAK\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1008px) 75vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jbv23y.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jbv23y.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jbv23y.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jbv23y.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jbv23y.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jbv23y.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jbv23y.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jbv23y.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jbv23y.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jbv23y.jpg.webp\\" loading=\\"lazy\\" alt=\\"Saving the real \'Paddington Bear\' in Bolivia\\" class=\\"sc-814e9212-0 frJKYu\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 grvvut\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 gglKuy\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-ceaf77c0-5 gznBZw\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 HiPPA\\">The \'Paddington bears\' facing climate threat<!-- --></h2></div></div><div data-testid=\\"card-description\\" class=\\"sc-ceaf77c0-0 eQvYOJ\\">Drought forces the real Paddington Bear into deadly conflict with cattle farmers in the Andes.</div><div data-testid=\\"card-button\\" class=\\"sc-ceaf77c0-6 kCHiOr\\"><button type=\\"button\\" aria-label=\\"Learn more\\" class=\\"sc-73629686-2 sc-73629686-4 blWAHP erTKhD\\"><span data-testid=\\"button-text\\" aria-hidden=\\"true\\" class=\\"sc-73629686-1 eUXcZD\\">See more</span></button></div></div></div></a></div></div></div></div></section><section data-testid=\\"wyoming-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Section without title - wyoming\\" data-analytics_group_type=\\"wyoming-0\\" data-analytics_group_position=\\"12\\" data-analytics_group_item_count=\\"0\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"wyoming-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><div class=\\"sc-93223220-0 ggGYNa\\"><div data-testid=\\"wyoming-group-grid\\" class=\\"sc-93223220-0 jitrPO\\"><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Business</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cj50d6q3jlro\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Ryanair set to slash summer fares as profits drop<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">8 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Business</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c4ng5q4jd62o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Former chancellor Zahawi mulling bid for the Telegraph<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">26 mins ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Business</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c4ng785gjv0o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Prime sued in trademark case by US Olympic committee<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cpe3zgznwjno\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/2bda/live/f864fb00-46bf-11ef-93d9-870ecb62df8e.jpg.webp\\" loading=\\"lazy\\" alt=\\"people at Melbourne airport\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">CrowdStrike IT outage affected 8.5 million Windows devices, Microsoft says<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">It\\u2019s the first time that a number has been put on the glitch that is still causing problems around the world.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Technology</span></div></div></div></a></div></div></div></div><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Innovation</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cp9vdxwjddeo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Company wins funding to make medicine in space<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">4 hrs ago</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future/article/20240719-why-covid-19-is-spreading-this-summer\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Summer surge: why Covid-19 isn\'t yet seasonal<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Future</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cq5xy12pynyo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Scam warning as fake emails and websites target users after outage<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Technology</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future/article/20240719-are-fermented-foods-actually-good-for-you\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jchp6h.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jchp6h.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jchp6h.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jchp6h.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jchp6h.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jchp6h.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jchp6h.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jchp6h.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jchp6h.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jchp6h.jpg.webp\\" loading=\\"lazy\\" alt=\\"A jar of kombucha against a pastel pink background (Credit: Getty Images)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Are fermented foods actually good for our health?<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">While humans have been eating fermented foods since ancient times, researchers are only starting to unravel some of the biggest questions about their health benefits.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Future</span></div></div></div></a></div></div></div></div></div><div data-testid=\\"wyoming-group-grid\\" class=\\"sc-93223220-0 jitrPO\\"><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Culture</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/culture/article/20240719-how-to-choose-the-best-and-most-eco-friendly-swimwear\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">How to choose the most eco-friendly swimwear<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Culture</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c28ejl4nvgyo\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">In pictures: Colonial India through the eyes of foreign artists<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Asia</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cp085ym9l3ro\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Auction for John Lennon glasses and Abbey Road photos<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Surrey</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/ceqdwpv8vw1o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/480/cpsprodpb/da1b/live/4c41b850-4678-11ef-895c-59d6554481d1.jpg.webp\\" loading=\\"lazy\\" alt=\\"A picture of Bella Hadid\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Bella Hadid\'s Adidas advert dropped after Israeli criticism<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">The model was chosen to promote shoes referencing the 1972 Munich Olympics, at which 11 Israeli athletes were killed.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Culture</span></div></div></div></a></div></div></div></div><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Travel</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20201026-dosa-indias-wholesome-fast-food-obsession\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The Indian dish Kamala Harris loves<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 hr ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240722-cinque-terre-italys-path-of-love-reopens-after-12-years\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Italy\'s most iconic trail reopens after 12 years<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The US\'s little-known \'Ellis Island of the West\'<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">5 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jchl7h.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jchl7h.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jchl7h.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jchl7h.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jchl7h.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jchl7h.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jchl7h.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jchl7h.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jchl7h.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jchl7h.jpg.webp\\" loading=\\"lazy\\" alt=\\"Dondurma (Credit: Alamy)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The Turkish ice cream eaten with a knife and fork<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Dondurma isn\'t like any other ice cream you\'ll find, and the epicentre of its production is still reeling from the powerful earthquakes that decimated the nation.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></div></a></div></div></div></div></div></div></div></section><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 fHxdon\\"><div data-testid=\\"dotcom-mid_4\\" id=\\"dotcom-mid_4\\" class=\\"dotcom-ad\\"></div></div><section data-testid=\\"oregon-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Travel\\" data-analytics_group_type=\\"oregon-1\\" data-analytics_group_position=\\"14\\" data-analytics_group_item_count=\\"1\\" data-analytics_group_link=\\"https://www.bbc.com/travel\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"oregon-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"oregon-line\\" class=\\"sc-54d40974-0 kJcwKJ\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 bfKEqT\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/travel\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div class=\\"sc-54d40974-5 eErSgx\\"><h2 data-testid=\\"oregon-title\\" class=\\"sc-54d40974-2 eKfxoB\\">Travel</h2> <!-- --><svg viewBox=\\"0 0 32 32\\" width=\\"15\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"chevron-right\\" data-testid=\\"section-title-chevron\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M21.6 14.3 5.5 31h6.4l14.6-15L11.9 1H5.5l16.1 16.7v-3.4z\\"></path></svg></div></a></div></div><div data-testid=\\"oregon-grid\\" class=\\"sc-93223220-0 bVbxSm\\"><div data-testid=\\"windsor-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"windsor-article\\" class=\\"sc-ceaf77c0-2 dxUpSs\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-ceaf77c0-4 gSbhqz\\"><div data-testid=\\"card-media\\" class=\\"sc-ceaf77c0-1 kLSjAK\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1280px) 50vw, (min-width: 1008px) 66vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jchx86.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jchx86.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jchx86.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jchx86.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jchx86.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jchx86.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jchx86.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jchx86.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jchx86.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jchx86.jpg.webp\\" loading=\\"lazy\\" alt=\\"Angel Island (Credit: Alamy)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-ceaf77c0-5 jDazYH\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 dsoipF\\">The US\'s little-known \'Ellis Island of the West\'<!-- --></h2></div></div><div data-testid=\\"card-description\\" class=\\"sc-ceaf77c0-0 eICGnP\\">This former quarantine and military station once processed as many as one million immigrants. Now, the picturesque island is one of the San Francisco Bay Area\'s best urban getaways.</div><div data-testid=\\"card-button\\" class=\\"sc-ceaf77c0-6 kCHiOr\\"><button type=\\"button\\" aria-label=\\"Learn more\\" class=\\"sc-73629686-2 sc-73629686-4 blWAHP iolhqz\\"><span data-testid=\\"button-text\\" aria-hidden=\\"true\\" class=\\"sc-73629686-1 eUXcZD\\">See more</span></button></div></div></div></a></div></div></div></div></section><section data-testid=\\"nevada-section-outer-3\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Sign up for our newsletters\\" data-analytics_group_type=\\"nevada-3\\" data-analytics_group_position=\\"15\\" data-analytics_group_item_count=\\"3\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"nevada-section-3\\" class=\\"sc-35aa3a40-2 cVXNac\\"><hr data-testid=\\"nevada-line\\" class=\\"sc-54d40974-0 kJcwKJ\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 ibsSfW\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 data-testid=\\"nevada-title\\" class=\\"sc-54d40974-2 eKfxoB\\">Sign up for our newsletters</h2></div></div></div><div data-testid=\\"nevada-grid-3\\" class=\\"sc-93223220-0 sc-ea30ba59-0 eINTLz gulFAT\\"><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://cloud.email.bbc.com/SignUp10_08?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=essentiallist&at_campaign_type=owned\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-customCard\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1008px) 25vw, (min-width: 600px) 33vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xp9.jpg.webp\\" loading=\\"lazy\\" alt=\\"The Essential List\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 jsCOsl\\"></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The Essential List<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">The week\'s best stories, handpicked by BBC editors, in your inbox every Tuesday and Friday.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://cloud.email.bbc.com/US_Election_Unspun_newsletter_signup?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=uselectionunspun&at_campaign_type=owned\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-customCard\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1008px) 25vw, (min-width: 600px) 33vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/1920x1080/p0h74xqg.jpg.webp\\" loading=\\"lazy\\" alt=\\"US Election Unspun\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 jsCOsl\\"></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">US Election Unspun<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Cut through the spin with North America correspondent Anthony Zurcher - in your inbox every Wednesday.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"></div></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://cloud.email.bbc.com/medalmoments_newsletter_signup?&at_bbc_team=studios&at_medium=emails&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.com&at_format=Module&at_link_origin=homepage&at_campaign=olympics2024&at_campaign_id=&at_adset_name=&at_adset_id=&at_creation=&at_creative_id=&at_campaign_type=owned\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-customCard\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"(min-width: 1008px) 25vw, (min-width: 600px) 33vw, 100vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/raw/p0j53vjd.jpg.webp\\" loading=\\"lazy\\" alt=\\"Medal Moments\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 jsCOsl\\"></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Medal Moments<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Your global guide to the Paris Olympics, from key highlights to heroic stories, daily to your inbox throughout the Games.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"></div></div></div></a></div></div></div></div></section><div data-component=\\"ad-slot\\" data-testid=\\"ad-unit\\" class=\\"sc-c361b622-0 fHxdon\\"><div data-testid=\\"dotcom-mid_5\\" id=\\"dotcom-mid_5\\" class=\\"dotcom-ad\\"></div></div><section data-testid=\\"wyoming-section-outer\\" data-analytics-group=\\"true\\" data-analytics_group_name=\\"Section without title - wyoming\\" data-analytics_group_type=\\"wyoming-0\\" data-analytics_group_position=\\"17\\" data-analytics_group_item_count=\\"0\\" data-analytics_group_link=\\"\\" class=\\"sc-35aa3a40-1 hMBIHa\\"><div data-testid=\\"wyoming-section\\" class=\\"sc-35aa3a40-2 cVXNac\\"><div class=\\"sc-93223220-0 ggGYNa\\"><div data-testid=\\"wyoming-group-grid\\" class=\\"sc-93223220-0 jitrPO\\"><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Earth</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-video\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><div class=\\"sc-4d9540e7-1 sc-4fedabc7-2 cfxUiL geTTpO\\"><svg viewBox=\\"0 0 32 32\\" width=\\"3em\\" height=\\"3em\\" category=\\"playback-avkx\\" icon=\\"play\\" data-testid=\\"inline-icon\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The \'Paddington bears\' facing climate threat<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Natural wonders</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future/article/20240715-the-simple-japanese-method-for-a-tidier-and-less-wasteful-fridge\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The simple Japanese method for a tidier fridge<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Future</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/c98qp79gj4no\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Conspiracy theories swirl about geo-engineering, but could it help save the planet?<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">BBC InDepth</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/reel/video/p0jbpt60/face-to-face-with-humpback-whales\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-video\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0jbqk2h.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0jbqk2h.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0jbqk2h.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0jbqk2h.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0jbqk2h.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0jbqk2h.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0jbqk2h.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0jbqk2h.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0jbqk2h.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0jbqk2h.jpg.webp\\" loading=\\"lazy\\" alt=\\"Humpback whale\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div><div data-testid=\\"content-type-label\\" class=\\"sc-4d9540e7-0 jsCOsl\\"><div data-testid=\\"content-type-icon-wrapper\\" class=\\"sc-4d9540e7-1 kjJkQD\\"><svg viewBox=\\"0 0 32 32\\" width=\\"30\\" height=\\"30\\" category=\\"playback-avkx\\" icon=\\"play\\" type=\\"playback-avkx:play\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M29 16 5.8 1v30L29 16z\\"></path></svg></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Face to face with humpback whales<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Reece Parkinson discovers how locals are protecting their stunning marine environment.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">3 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></div></a></div></div></div></div><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">Science and health</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cj50d7e9vp6o\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">India alert after boy dies from Nipah virus in Kerala<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">6 hrs ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Asia</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future/article/20240719-are-fermented-foods-actually-good-for-you\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Are fermented foods actually good for our health?<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Future</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future/article/20240719-why-covid-19-is-spreading-this-summer\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Summer surge: why Covid-19 isn\'t yet seasonal<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Future</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news/articles/cyx0perl8yno\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/news/240/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 240w,https://ichef.bbci.co.uk/news/320/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 320w,https://ichef.bbci.co.uk/news/480/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 480w,https://ichef.bbci.co.uk/news/640/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 640w,https://ichef.bbci.co.uk/news/800/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 800w,https://ichef.bbci.co.uk/news/1024/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 1024w,https://ichef.bbci.co.uk/news/1536/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp 1536w\\" src=\\"https://ichef.bbci.co.uk/news/1536/cpsprodpb/3884/live/affa3d80-45e3-11ef-837a-9936fb8608b6.jpg.webp\\" loading=\\"lazy\\" alt=\\"A young woman\'s face with a red background\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">\'A way to fight back\': FGM survivors reclaim vulvas with surgery<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">In Somalia, it\'s believed cutting off a girl\'s outer genitalia will guarantee their virginity. </p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Africa</span></div></div></div></a></div></div></div></div></div><div data-testid=\\"wyoming-group-grid\\" class=\\"sc-93223220-0 jitrPO\\"><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">World\\u2019s Table</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The Turkish ice cream eaten with a knife and fork<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 day ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240715-gond-katira-a-natural-way-to-cool-down-in-indias-scorching-summers\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">India\'s cooling drinks to beat the heat<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">7 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240708-paris-olympics-2024-worlds-biggest-restaurant\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The world\'s biggest restaurant is coming to Paris<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">9 Jul 2024</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240531-the-wild-ceremonies-surrounding-a-turkish-meatball\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0hzj391.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0hzj391.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0hzj391.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0hzj391.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0hzj391.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0hzj391.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0hzj391.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0hzj391.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0hzj391.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0hzj391.jpg.webp\\" loading=\\"lazy\\" alt=\\"Plate of \\u00e7i\\u011f k\\u00f6fte made with raw meat (Credit: Paul Benjamin Osterlund)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">The wild ceremonies of the Turkish \'meatball\'<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">One of the country\'s most popular fast-food items, \\u00e7i\\u011f k\\u00f6fte is traditionally associated with wild and rowdy gatherings in south-eastern Turkey.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">1 Jun 2024</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></div></a></div></div></div></div><div data-testid=\\"wyoming-card-grid\\"><hr class=\\"sc-54d40974-0 bDwYmM\\"><div data-testid=\\"section-title-wrapper\\" class=\\"sc-54d40974-1 dQkuqR\\"><div><div class=\\"sc-54d40974-5 eErSgx\\"><h2 class=\\"sc-54d40974-2 eKfxoB\\">The Specialist</h2></div></div></div><div class=\\"sc-93223220-0 bBjmKu\\"><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240718-a-happiness-hackers-guide-to-the-happiest-outdoor-places-in-helsinki\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Guide to Helsinki\'s happiest places<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">2 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240717-david-lebovitzs-ultimate-guide-to-the-best-bakeries-in-paris-right-now\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">A pastry chef\'s favourite bakeries in Paris<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">5 days ago</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"chester-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240714-chef-andrew-zimmerns-favourite-us-restaurants\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"chester-article\\" class=\\"sc-417019fc-0 bnGVfD\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">Chef Andrew Zimmern\'s favourite US restaurants<!-- --></h2></div></div><div class=\\"sc-4e537b1-0 hJDQRX\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">14 Jul 2024</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></a></div></div><div data-testid=\\"edinburgh-card\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel/article/20240710-icelands-first-lady-takes-you-on-a-tour-of-her-super-chill-nation\\" class=\\"sc-2e6baa30-0 gILusN\\"><div data-testid=\\"edinburgh-article\\" class=\\"sc-b8778340-0 kFuHJG\\"><div data-testid=\\"card-media-wrapper\\" class=\\"sc-b8778340-1 jvzsLx\\"><div data-testid=\\"card-media\\" class=\\"sc-b8778340-2 kUyIkJ\\"><div class=\\"sc-814e9212-1 fcEyBx\\"><img src=\\"/bbcx/grey-placeholder.png\\" class=\\"sc-814e9212-0 cCvKR hide-when-no-script\\"><img sizes=\\"96vw\\" srcset=\\"https://ichef.bbci.co.uk/images/ic/160x90/p0j9bt66.jpg.webp 160w,https://ichef.bbci.co.uk/images/ic/240x135/p0j9bt66.jpg.webp 240w,https://ichef.bbci.co.uk/images/ic/320x180/p0j9bt66.jpg.webp 320w,https://ichef.bbci.co.uk/images/ic/480x270/p0j9bt66.jpg.webp 480w,https://ichef.bbci.co.uk/images/ic/640x360/p0j9bt66.jpg.webp 640w,https://ichef.bbci.co.uk/images/ic/800x450/p0j9bt66.jpg.webp 800w,https://ichef.bbci.co.uk/images/ic/1024x576/p0j9bt66.jpg.webp 1024w,https://ichef.bbci.co.uk/images/ic/1376x774/p0j9bt66.jpg.webp 1376w,https://ichef.bbci.co.uk/images/ic/1920x1080/p0j9bt66.jpg.webp 1920w\\" src=\\"https://ichef.bbci.co.uk/images/ic/480x270/p0j9bt66.jpg.webp\\" loading=\\"lazy\\" alt=\\"Iceland in winter (Credit: Getty Images)\\" class=\\"sc-814e9212-0 hIXOPW\\"></div></div></div><div data-testid=\\"card-text-wrapper\\" class=\\"sc-b8778340-3 gxEarx\\"><div class=\\"sc-4fedabc7-1 kbvCmj\\"><div class=\\"sc-4fedabc7-0 kZtaAl\\"><h2 data-testid=\\"card-headline\\" class=\\"sc-4fedabc7-3 zTZri\\">A First Lady\'s guide to Iceland<!-- --></h2></div></div><p data-testid=\\"card-description\\" class=\\"sc-b8778340-4 kYtujW\\">Eliza Reid moved to Iceland 20 years ago for love and now she\'s the First Lady. Here are her favourite ways to enjoy a \\"chill\\" Icelandic weekend.</p><div class=\\"sc-4e537b1-0 gtLVrL\\"><span data-testid=\\"card-metadata-lastupdated\\" class=\\"sc-4e537b1-1 dsUUMv\\">11 Jul 2024</span><div data-testid=\\"card-metadata-separator\\" class=\\"sc-4e537b1-3 lmDmEx\\"></div><span data-testid=\\"card-metadata-tag\\" class=\\"sc-4e537b1-2 eRsxHt\\">Travel</span></div></div></div></a></div></div></div></div></div></div></div></section></article><div class=\\"zephr_meter_beta\\"></div><div class=\\"zephr_index_beta\\"></div></main><hr data-testid=\\"main-footer-divider\\" class=\\"sc-be00b565-2 cxZZfa\\"><footer data-testid=\\"main-footer\\" class=\\"sc-be00b565-0 hfihLu\\"><div class=\\"sc-be00b565-3 bMDFAT\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/\\" class=\\"sc-2e6baa30-0 gILusN\\"><svg xmlns=\\"http://www.w3.org/2000/svg\\" viewBox=\\"0 0 112 32\\" category=\\"logo\\" icon=\\"bbc\\" style=\\"height:30px\\" class=\\"sc-1097f7fe-0 jbvZzi\\"><title>British Broadcasting Corporation</title><path d=\\"M111.99999,4.44444577e-05 L111.99999,32.0000444 L79.9999905,32.0000444 L79.9999905,4.44444577e-05 L111.99999,4.44444577e-05 Z M72.0000119,-3.55271368e-15 L72.0000119,32 L40.0000119,32 L40.0000119,-3.55271368e-15 L72.0000119,-3.55271368e-15 Z M32,-3.55271368e-15 L32,32 L-1.13686838e-13,32 L-1.13686838e-13,-3.55271368e-15 L32,-3.55271368e-15 Z M97.469329,6.80826869 C96.0294397,6.80826869 94.7294393,7.02226876 93.5693278,7.44982444 C92.4089942,7.87782457 91.4137717,8.49471364 90.5841047,9.30049166 C89.7538823,10.1067141 89.1188821,11.07327 88.6785486,12.199937 C88.2378818,13.3269373 88.0177706,14.5896043 88.0177706,15.9876048 C88.0177706,17.4188274 88.2296596,18.7062722 88.6531042,19.8493837 C89.0763265,20.9929396 89.6861045,21.9591621 90.482438,22.748829 C91.2784383,23.5383848 92.2522163,24.1430516 93.4042167,24.5624962 C94.5558837,24.9819408 95.8516619,25.1917186 97.2914401,25.1917186 C98.3752182,25.1917186 99.4086629,25.072163 100.391219,24.8338296 C101.37333,24.5956073 102.237108,24.2706072 102.982664,23.8592738 L102.982664,23.8592738 L102.982664,20.4292727 C101.40733,21.4001619 99.6881074,21.8851621 97.8251069,21.8851621 C96.6054399,21.8851621 95.567884,21.6549398 94.7126615,21.194273 C93.8572168,20.7338284 93.2049944,20.0633837 92.7564387,19.1831613 C92.3073275,18.3032721 92.0831052,17.2380496 92.0831052,15.9876048 C92.0831052,14.7377155 92.3156608,13.6766041 92.7816609,12.8044927 C93.2474389,11.9327147 93.916328,11.2664922 94.7888838,10.8058254 C95.6609951,10.3453809 96.715551,10.1148252 97.9521069,10.1148252 C98.8496628,10.1148252 99.7052186,10.2342697 100.518108,10.4726031 C101.331219,10.7112699 102.084664,11.0609366 102.779442,11.5212701 L102.779442,11.5212701 L102.779442,8.01738016 C102.017219,7.62260227 101.191441,7.32260218 100.302219,7.11671323 C99.4129963,6.91126872 98.4685515,6.80826869 97.469329,6.80826869 Z M55.7552388,7.00000208 L49.0000146,7.00000208 L49.0000146,25.0000021 L56.1713501,25.0000021 C57.590906,25.0000021 58.8063508,24.7903407 59.8181289,24.3706739 C60.8297959,23.9513405 61.6087961,23.3553403 62.1555741,22.5832289 C62.7020187,21.8114509 62.9754632,20.8882284 62.9754632,19.8140059 C62.9754632,18.7232278 62.6941298,17.7960053 62.1312407,17.0321162 C61.5681294,16.2686715 60.7563514,15.7104491 59.6957955,15.3580046 C60.4625736,14.9891156 61.0420182,14.4894488 61.4335738,13.8601152 C61.8252406,13.2307817 62.0210185,12.4881148 62.0210185,11.6321146 C62.0210185,10.1385586 61.4742405,8.99311379 60.3812402,8.19578022 C59.2877954,7.39889109 57.745795,7.00000208 55.7552388,7.00000208 L55.7552388,7.00000208 Z M15.7552269,7.00000208 L9.00000268,7.00000208 L9.00000268,25.0000021 L16.1713381,25.0000021 C17.5908941,25.0000021 18.8062278,24.7903407 19.8182281,24.3706739 C20.8296729,23.9513405 21.6087842,23.3553403 22.1555621,22.5832289 C22.7021179,21.8114509 22.9755624,20.8882284 22.9755624,19.8140059 C22.9755624,18.7232278 22.6941179,17.7960053 22.1311177,17.0321162 C21.5682286,16.2686715 20.7563395,15.7104491 19.6957836,15.3580046 C20.4625616,14.9891156 21.0418952,14.4894488 21.4335619,13.8601152 C21.8252287,13.2307817 22.0210066,12.4881148 22.0210066,11.6321146 C22.0210066,10.1385586 21.4741175,8.99311379 20.3811172,8.19578022 C19.2877835,7.39889109 17.7457831,7.00000208 15.7552269,7.00000208 L15.7552269,7.00000208 Z M55.8775833,17.2209385 C58.1128062,17.2209385 59.2308065,18.0434943 59.2308065,19.6881614 C59.2308065,20.4602728 58.9369175,21.0518285 58.3496951,21.4629397 C57.7622505,21.8743843 56.9216947,22.0797177 55.8286944,22.0797177 L55.8286944,22.0797177 L52.6469157,22.0797177 L52.6469157,17.2209385 Z M15.8775714,17.2209385 C18.1129054,17.2209385 19.2307946,18.0434943 19.2307946,19.6881614 C19.2307946,20.4602728 18.9370167,21.0518285 18.3496832,21.4629397 C17.7622386,21.8743843 16.9216828,22.0797177 15.8286825,22.0797177 L15.8286825,22.0797177 L12.6469038,22.0797177 L12.6469038,17.2209385 Z M55.412572,9.92028073 C57.3541282,9.92028073 58.3252396,10.6338365 58.3252396,12.0600591 C58.3252396,12.7988371 58.0763506,13.373504 57.5786838,13.7846152 C57.0807948,14.1960598 56.3587946,14.4013932 55.412572,14.4013932 L55.412572,14.4013932 L52.6469046,14.4013932 L52.6469046,9.92028073 Z M15.4125601,9.92028073 C17.3541163,9.92028073 18.3252277,10.6338365 18.3252277,12.0600591 C18.3252277,12.7988371 18.0762276,13.373504 17.5786719,13.7846152 C17.0807829,14.1960598 16.3587826,14.4013932 15.4125601,14.4013932 L15.4125601,14.4013932 L12.6468927,14.4013932 L12.6468927,9.92028073 Z\\"></path></svg></a></div></div><section class=\\"sc-19b1eb93-0 hYxbtM\\"><nav role=\\"navigation\\" aria-label=\\"Footer navigation\\"><ul class=\\"sc-19b1eb93-1 fwyFkM\\"><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Home</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/news\\" class=\\"sc-19b1eb93-2 hsqCXf\\">News</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/sport\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Sport</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/business\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Business</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/innovation\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Innovation</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/culture\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Culture</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/travel\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Travel</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/future-planet\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Earth</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/video\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Video</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a data-testid=\\"internal-link\\" href=\\"/live\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Live</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.co.uk/sounds\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Audio</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/weather\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-19b1eb93-2 hsqCXf\\">Weather</a></div></li><li data-testid=\\"contentlink-li\\"><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://shop.bbc.com/\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-19b1eb93-2 hsqCXf\\">BBC Shop</a></div></li></ul></nav></section><section class=\\"sc-b310c64c-0 cUeFDY\\"><div class=\\"sc-b310c64c-1 gyQHkZ\\"><button type=\\"button\\" class=\\"sc-b310c64c-2 gdhjHH\\">BBC in other languages<!-- --><div class=\\"sc-b310c64c-3 iqAXuG\\"><svg viewBox=\\"0 0 32 32\\" width=\\"1em\\" height=\\"1em\\" category=\\"arrows\\" icon=\\"arrow-down\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M26.7 12.6 16 23.2 5.3 12.6V8.8h21.4v3.8z\\"></path></svg></div></button></div></section><div data-testid=\\"social-follow-us-container\\" class=\\"sc-97754ce7-0 iNEkxQ\\"><h2 class=\\"sc-97754ce7-1 friRUH\\">Follow BBC on:</h2><div data-testid=\\"social-follow-us-icon-buttons\\" class=\\"sc-97754ce7-2 gRRybj\\"><div class=\\"sc-97754ce7-5 hmVYPm\\"><button type=\\"button\\" data-testid=\\"social-button-x\\" class=\\"sc-73629686-2 sc-73629686-5 sc-73629686-6 clXhsB bJPKrX hIAEXL\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 fXpyYW\\"><svg width=\\"14\\" height=\\"14\\" viewBox=\\"0 0 14 14\\" fill=\\"none\\" xmlns=\\"http://www.w3.org/2000/svg\\" category=\\"social\\" icon=\\"x\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M8.3319 5.92804L13.5437 0H12.3087L7.78327 5.14724L4.16883 0H0L5.46574 7.78354L0 14H1.2351L6.01406 8.56434L9.83117 14H14L8.3316 5.92804H8.3319ZM6.64026 7.85211L6.08647 7.07705L1.68013 0.909776H3.57717L7.13314 5.88696L7.68693 6.66202L12.3093 13.1316H10.4122L6.64026 7.85241V7.85211Z\\"></path></svg></span></button></div><div class=\\"sc-97754ce7-5 hmVYPm\\"><button type=\\"button\\" data-testid=\\"social-button-facebook\\" class=\\"sc-73629686-2 sc-73629686-5 sc-73629686-6 clXhsB bJPKrX hIAEXL\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 fXpyYW\\"><svg width=\\"9\\" height=\\"16\\" viewBox=\\"0 0 9 16\\" xmlns=\\"http://www.w3.org/2000/svg\\" category=\\"social\\" icon=\\"facebook\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M6.80799 2.65602H8.31199V0.112024C7.58379 0.0363015 6.85211 -0.00108342 6.11999 2.38913e-05C3.94399 2.38913e-05 2.456 1.32802 2.456 3.76002V5.85601H0V8.70401H2.456V16H5.39999V8.70401H7.84799L8.21599 5.85601H5.39999V4.04002C5.39999 3.20002 5.62399 2.65602 6.80799 2.65602Z\\"></path></svg></span></button></div><div class=\\"sc-97754ce7-5 hmVYPm\\"><button type=\\"button\\" data-testid=\\"social-button-instagram\\" class=\\"sc-73629686-2 sc-73629686-5 sc-73629686-6 clXhsB bJPKrX hIAEXL\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 fXpyYW\\"><svg width=\\"18\\" height=\\"17\\" viewBox=\\"0 0 18 17\\" fill=\\"none\\" xmlns=\\"http://www.w3.org/2000/svg\\" category=\\"social\\" icon=\\"instagram\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M12.7227 0.189697H5.28726C4.13732 0.190927 3.03482 0.648288 2.22168 1.46143C1.40854 2.27456 0.951181 3.37706 0.949951 4.52701V11.9624C0.951181 13.1124 1.40854 14.2149 2.22168 15.028C3.03482 15.8411 4.13732 16.2985 5.28726 16.2997H12.7227C13.8726 16.2985 14.9751 15.8411 15.7882 15.028C16.6014 14.2149 17.0587 13.1124 17.06 11.9624V4.52701C17.0587 3.37706 16.6014 2.27456 15.7882 1.46143C14.9751 0.648288 13.8726 0.190927 12.7227 0.189697ZM9.00496 11.9624C8.26967 11.9624 7.55089 11.7444 6.93952 11.3359C6.32815 10.9274 5.85164 10.3467 5.57026 9.66741C5.28887 8.98809 5.21525 8.24058 5.3587 7.51942C5.50215 6.79826 5.85622 6.13583 6.37615 5.6159C6.89608 5.09597 7.55851 4.74189 8.27968 4.59845C9.00084 4.455 9.74834 4.52862 10.4277 4.81C11.107 5.09139 11.6876 5.56789 12.0961 6.17927C12.5046 6.79064 12.7227 7.50942 12.7227 8.24471C12.7216 9.23039 12.3296 10.1754 11.6326 10.8724C10.9357 11.5694 9.99064 11.9614 9.00496 11.9624ZM13.6521 4.52701C13.4683 4.52701 13.2886 4.4725 13.1357 4.37038C12.9829 4.26825 12.8638 4.12309 12.7934 3.95326C12.7231 3.78343 12.7047 3.59656 12.7405 3.41626C12.7764 3.23597 12.8649 3.07037 12.9949 2.94038C13.1249 2.8104 13.2905 2.72188 13.4708 2.68602C13.6511 2.65016 13.8379 2.66856 14.0078 2.73891C14.1776 2.80926 14.3227 2.92838 14.4249 3.08123C14.527 3.23407 14.5815 3.41376 14.5815 3.59759C14.5815 3.84409 14.4836 4.08049 14.3093 4.25479C14.135 4.42909 13.8986 4.52701 13.6521 4.52701ZM11.4834 8.24471C11.4834 8.7349 11.3381 9.21409 11.0657 9.62167C10.7934 10.0293 10.4063 10.3469 9.95343 10.5345C9.50055 10.7221 9.00221 10.7712 8.52144 10.6756C8.04066 10.5799 7.59904 10.3439 7.25242 9.99725C6.9058 9.65063 6.66975 9.20901 6.57412 8.72823C6.47849 8.24746 6.52757 7.74912 6.71516 7.29624C6.90275 6.84336 7.22042 6.45628 7.628 6.18394C8.03558 5.9116 8.51477 5.76624 9.00496 5.76624C9.66229 5.76624 10.2927 6.02737 10.7575 6.49217C11.2223 6.95697 11.4834 7.58738 11.4834 8.24471Z\\" fill=\\"black\\"></path></svg></span></button></div><div class=\\"sc-97754ce7-5 hmVYPm\\"><button type=\\"button\\" data-testid=\\"social-button-tiktok\\" class=\\"sc-73629686-2 sc-73629686-5 sc-73629686-6 clXhsB bJPKrX hIAEXL\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 fXpyYW\\"><svg width=\\"16\\" height=\\"19\\" viewBox=\\"0 0 16 19\\" fill=\\"none\\" xmlns=\\"http://www.w3.org/2000/svg\\" category=\\"social\\" icon=\\"tiktok\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M12.6992 2.92929C12.0014 2.13265 11.6168 1.10958 11.6171 0.0505371H8.46271V12.7089C8.43838 13.3939 8.14918 14.0427 7.65601 14.5187C7.16284 14.9948 6.50419 15.2609 5.81875 15.261C4.36917 15.261 3.16458 14.0768 3.16458 12.6068C3.16458 10.851 4.85917 9.53408 6.60479 10.0751V6.84929C3.08292 6.3797 0 9.11554 0 12.6068C0 16.0062 2.8175 18.4255 5.80854 18.4255C9.01396 18.4255 11.6171 15.8224 11.6171 12.6068V6.18575C12.8962 7.10434 14.4319 7.5972 16.0067 7.5945V4.44012C16.0067 4.44012 14.0875 4.532 12.6992 2.92929Z\\" fill=\\"black\\"></path></svg></span></button></div><div class=\\"sc-97754ce7-5 hmVYPm\\"><button type=\\"button\\" data-testid=\\"social-button-linkedin\\" class=\\"sc-73629686-2 sc-73629686-5 sc-73629686-6 clXhsB bJPKrX hIAEXL\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 fXpyYW\\"><svg width=\\"15\\" height=\\"15\\" viewBox=\\"0 0 15 15\\" xmlns=\\"http://www.w3.org/2000/svg\\" category=\\"social\\" icon=\\"linkedin\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M3.14795 1.57476C3.14775 1.99221 2.98172 2.39247 2.68639 2.6875C2.39107 2.98253 1.99063 3.14816 1.57319 3.14795C1.15575 3.14775 0.755481 2.98172 0.460451 2.68639C0.16542 2.39107 -0.000208525 1.99064 1.97032e-07 1.57319C0.000208919 1.15575 0.166238 0.755481 0.461564 0.460451C0.756889 0.16542 1.15732 -0.000208525 1.57476 1.97032e-07C1.99221 0.000208919 2.39247 0.166238 2.6875 0.461564C2.98253 0.756889 3.14816 1.15732 3.14795 1.57476ZM3.19517 4.31348H0.0472195V14.1666H3.19517V4.31348ZM8.16894 4.31348H5.03673V14.1666H8.13746V8.99607C8.13746 6.11569 11.8914 5.84811 11.8914 8.99607V14.1666H15V7.92576C15 3.07004 9.44386 3.25105 8.13746 5.63563L8.16894 4.31348Z\\"></path></svg></span></button></div><div class=\\"sc-97754ce7-5 hmVYPm\\"><button type=\\"button\\" data-testid=\\"social-button-youtube\\" class=\\"sc-73629686-2 sc-73629686-5 sc-73629686-6 clXhsB bJPKrX hIAEXL\\"><span data-testid=\\"button-icon-wrapper\\" class=\\"sc-73629686-0 fXpyYW\\"><svg width=\\"19\\" height=\\"15\\" viewBox=\\"0 0 19 15\\" fill=\\"none\\" xmlns=\\"http://www.w3.org/2000/svg\\" category=\\"social\\" icon=\\"youtube\\" aria-hidden=\\"true\\" class=\\"sc-1097f7fe-0 jmthjj\\"><path d=\\"M9.48685 0.29126C9.98138 0.293864 11.2186 0.305151 12.5337 0.35464L13.0004 0.37374C14.3238 0.431911 15.6463 0.532624 16.3029 0.703663C17.178 0.934609 17.8652 1.60661 18.0976 2.4592C18.468 3.81362 18.5144 6.45474 18.5199 7.09462L18.5208 7.22659V7.37766C18.5144 8.01753 18.468 10.6595 18.0976 12.0131C17.8624 12.8683 17.1743 13.5411 16.3029 13.7686C15.6463 13.9397 14.3238 14.0404 13.0004 14.0985L12.5337 14.1185C11.2186 14.1671 9.98138 14.1793 9.48685 14.181L9.26921 14.1819H9.03306C7.98658 14.1758 3.60989 14.1315 2.21705 13.7686C1.34283 13.5377 0.654744 12.8657 0.422296 12.0131C0.051861 10.6587 0.00555653 8.01753 0 7.37766V7.09462C0.00555653 6.45474 0.051861 3.81275 0.422296 2.4592C0.657523 1.60401 1.34561 0.931136 2.21798 0.704532C3.60989 0.340748 7.98751 0.296469 9.03399 0.29126H9.48685ZM7.40778 4.19824V10.2758L12.9643 7.23701L7.40778 4.19824Z\\" fill=\\"black\\"></path></svg></span></button></div></div></div><section class=\\"sc-4e8f0faa-0 ihVvAu\\"><nav><ul class=\\"sc-4e8f0faa-1 gQuDzC\\"><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.co.uk/usingthebbc/terms\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Terms of Use</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.co.uk/aboutthebbc\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">About the BBC</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/usingthebbc/privacy/\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Privacy Policy</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/usingthebbc/cookies/\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Cookies</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.co.uk/accessibility/\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Accessibility Help</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.co.uk/contact\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Contact the BBC</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/advertisingcontact\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Advertise with us</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/usingthebbc/cookies/how-can-i-change-my-bbc-cookie-settings/\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Do not share or sell my info</a></div></li><li><div data-testid=\\"anchor-inner-wrapper\\"><a href=\\"https://www.bbc.com/contact-bbc-com-help\\" data-testid=\\"external-anchor\\" target=\\"_self\\" class=\\"sc-4e8f0faa-2 hBaEGJ\\">Contact technical support</a></div></li></ul></nav></section><div class=\\"sc-be00b565-1 ljTcnM\\"><p class=\\"sc-eb7bd5f6-0 fYAfXe\\">Copyright 2024 BBC. All rights reserved. <!-- --><i id=\\"\\" class=\\"sc-7dcfb11b-0 kKcaog\\"> <!-- --></i>The<!-- --><i id=\\"bbc\\" class=\\"sc-7dcfb11b-0 kKcaog\\"> BBC <!-- --></i>is<!-- --><i id=\\"not-responsible-for-the-content-of-external-sites.\\" class=\\"sc-7dcfb11b-0 kKcaog\\"> not responsible for the content of external sites.<!-- --></i> <!-- --><a target=\\"_self\\" href=\\"https://www.bbc.co.uk/editorialguidelines/guidance/feeds-and-links\\" class=\\"sc-c9299ecf-0 bZUiKB\\"><b id=\\"read-about-our-approach-to-external-linking.\\" class=\\"sc-7dcfb11b-0 kVRnKf\\">Read about our approach to external linking.<!-- --></b></a></p><p class=\\"sc-eb7bd5f6-0 fYAfXe\\"> <!-- --></p></div></footer></div></div><next-route-announcer><p aria-live=\\"assertive\\" id=\\"__next-route-announcer__\\" role=\\"alert\\" style=\\"border: 0px; clip: rect(0px, 0px, 0px, 0px); height: 1px; margin: -1px; overflow: hidden; padding: 0px; position: absolute; width: 1px; white-space: nowrap; overflow-wrap: normal;\\"></p></next-route-announcer><div></div></body></html>", "metadata": {"title": "BBC Home - Breaking News, World News, US News, Sports, Business, Innovation, Climate, Culture, Travel, Video & AudioBritish Broadcasting CorporationBritish Broadcasting Corporation", "description": "Visit BBC for trusted reporting on the latest world and US news, sports, business, climate, innovation, culture and much more.", "robots": "NOODP, NOYDIR", "ogTitle": "BBC Home - Breaking News, World News, US News, Sports, Business, Innovation, Climate, Culture, Travel, Video & Audio", "ogDescription": "Visit BBC for trusted reporting on the latest world and US news, sports, business, climate, innovation, culture and much more.", "ogLocaleAlternate": [], "sourceURL": "https://www.bbc.com", "pageStatusCode": 200}, "linksOnPage": ["https://www.bbc.com/", "https://www.bbc.com/watch-live-news", "https://www.bbc.com/news", "https://www.bbc.com/sport", "https://www.bbc.com/business", "https://www.bbc.com/innovation", "https://www.bbc.com/culture", "https://www.bbc.com/travel", "https://www.bbc.com/future-planet", "https://www.bbc.com/video", "https://www.bbc.com/live", "https://www.bbc.co.uk/sounds", "https://www.bbc.com/weather", "https://www.bbc.com/newsletters", "https://www.bbc.com/news/articles/c250zqgrpqgo", "https://www.bbc.com/news/articles/c3gdzmdje5xo", "https://www.bbc.com/news/live/cv2gryx1yx1t", "https://www.bbc.com/news/articles/cpwd8yzw45qo", "https://www.bbc.com/news/articles/c80ekdwk9zro", "https://www.bbc.com/news/live/c724wqpy4qnt", "https://www.bbc.com/news/articles/c16j896003xo", "https://www.bbc.com/future/article/20240722-why-you-are-probably-sitting-down-for-too-long", "https://www.bbc.com/news/articles/c51yqdy3q61o", "https://www.bbc.com/news/articles/crgk8gnpg0zo", "https://www.bbc.com/news/articles/cgerz8we1vgo", "https://www.bbc.com/news/articles/cw4yxd3dw7qo", "https://www.bbc.com/sport/formula1/articles/cg3jzy3e8q1o", "https://www.bbc.com/news/articles/cj50d7e9vp6o", "https://www.bbc.com/news/articles/czrj18yp489o", "https://www.bbc.com/news/articles/cgl7e33n1d0o", "https://www.bbc.com/news/articles/cq5jwyel12qo", "https://www.bbc.com/news/videos/cx028eq4qg1o", "https://www.bbc.com/news/videos/cmj24x03r8po", "https://www.bbc.com/news/videos/c51yrr2z74no", "https://www.bbc.com/reel/video/p0jch19q/turkey-s-answer-to-burning-man-", "https://www.bbc.com/sport/basketball/videos/cg640yk3n3zo", "https://www.bbc.com/news/videos/cqe6q917y1jo", "https://www.bbc.com/news/videos/c0jq593xqk8o", "https://www.bbc.com/news/videos/cjk325014g7o", "https://www.bbc.com/culture/article/20240718-a-ww1-soldier-on-the-brutality-of-conflict", "https://www.bbc.com/sport/olympics", "https://www.bbc.com/sport/football/articles/cek91m98g48o", "https://www.bbc.com/news/articles/crgk8rgm87lo", "https://www.bbc.com/news/articles/cq5xdq71drro", "https://www.bbc.com/news/videos/c51y936y9g2o", "https://www.bbc.com/news/articles/cn08d7vyj6wo", "https://www.bbc.com/news/articles/cn08d7j1qj5o", "https://www.bbc.com/news/articles/cd1e48677w0o", "https://www.bbc.com/news/articles/cxw29lg4y8go", "https://www.bbc.com/news/articles/c4ngrdpwlx3o", "https://www.bbc.com/news/articles/cxr2g7rk3dxo", "https://www.bbc.com/sport/football/articles/c9e950ev0xpo", "https://www.bbc.com/sport/football/articles/cgrlgzx6gpro", "https://www.bbc.com/sport/articles/c2v0j0j6nqlo", "https://www.bbc.com/sport/golf/articles/cv2g1glwypqo", "https://www.bbc.com/reel/video/p0jbv222/climate-chaos-makes-paddington-bear-hangry-", "https://www.bbc.com/news/articles/cj50d6q3jlro", "https://www.bbc.com/news/articles/c4ng5q4jd62o", "https://www.bbc.com/news/articles/c4ng785gjv0o", "https://www.bbc.com/news/articles/cpe3zgznwjno", "https://www.bbc.com/news/articles/cp9vdxwjddeo", "https://www.bbc.com/future/article/20240719-why-covid-19-is-spreading-this-summer", "https://www.bbc.com/news/articles/cq5xy12pynyo", "https://www.bbc.com/future/article/20240719-are-fermented-foods-actually-good-for-you", "https://www.bbc.com/culture/article/20240719-how-to-choose-the-best-and-most-eco-friendly-swimwear", "https://www.bbc.com/news/articles/c28ejl4nvgyo", "https://www.bbc.com/news/articles/cp085ym9l3ro", "https://www.bbc.com/news/articles/ceqdwpv8vw1o", "https://www.bbc.com/travel/article/20201026-dosa-indias-wholesome-fast-food-obsession", "https://www.bbc.com/travel/article/20240722-cinque-terre-italys-path-of-love-reopens-after-12-years", "https://www.bbc.com/travel/article/20240719-angel-island-the-little-known-ellis-island-of-the-west-us", "https://www.bbc.com/travel/article/20240719-dondurma-the-turkish-ice-cream-eaten-with-a-knife-and-fork", "https://cloud.email.bbc.com/SignUp10_08?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=essentiallist&at_campaign_type=owned", "https://cloud.email.bbc.com/US_Election_Unspun_newsletter_signup?&at_bbc_team=studios&at_medium=display&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.comhp&at_format=Module&at_link_origin=homepage&at_campaign=uselectionunspun&at_campaign_type=owned", "https://cloud.email.bbc.com/medalmoments_newsletter_signup?&at_bbc_team=studios&at_medium=emails&at_objective=acquisition&at_ptr_type=&at_ptr_name=bbc.com&at_format=Module&at_link_origin=homepage&at_campaign=olympics2024&at_campaign_id=&at_adset_name=&at_adset_id=&at_creation=&at_creative_id=&at_campaign_type=owned", "https://www.bbc.com/future/article/20240715-the-simple-japanese-method-for-a-tidier-and-less-wasteful-fridge", "https://www.bbc.com/news/articles/c98qp79gj4no", "https://www.bbc.com/reel/video/p0jbpt60/face-to-face-with-humpback-whales", "https://www.bbc.com/news/articles/cyx0perl8yno", "https://www.bbc.com/travel/article/20240715-gond-katira-a-natural-way-to-cool-down-in-indias-scorching-summers", "https://www.bbc.com/travel/article/20240708-paris-olympics-2024-worlds-biggest-restaurant", "https://www.bbc.com/travel/article/20240531-the-wild-ceremonies-surrounding-a-turkish-meatball", "https://www.bbc.com/travel/article/20240718-a-happiness-hackers-guide-to-the-happiest-outdoor-places-in-helsinki", "https://www.bbc.com/travel/article/20240717-david-lebovitzs-ultimate-guide-to-the-best-bakeries-in-paris-right-now", "https://www.bbc.com/travel/article/20240714-chef-andrew-zimmerns-favourite-us-restaurants", "https://www.bbc.com/travel/article/20240710-icelands-first-lady-takes-you-on-a-tour-of-her-super-chill-nation", "https://shop.bbc.com/", "https://www.bbc.co.uk/usingthebbc/terms", "https://www.bbc.co.uk/aboutthebbc", "https://www.bbc.com/usingthebbc/privacy/", "https://www.bbc.com/usingthebbc/cookies/", "https://www.bbc.co.uk/accessibility/", "https://www.bbc.co.uk/contact", "https://www.bbc.com/advertisingcontact", "https://www.bbc.com/usingthebbc/cookies/how-can-i-change-my-bbc-cookie-settings/", "https://www.bbc.com/contact-bbc-com-help", "https://www.bbc.co.uk/editorialguidelines/guidance/feeds-and-links"]}, "returnCode": 200}', role=<ChatRole.USER: 'user'>, name=None, meta={})]}}